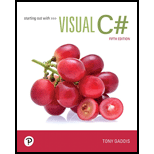
Starting Out With Visual C# (5th Edition)
5th Edition
ISBN: 9780135183519
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.9, Problem 7.23CP
Program Plan Intro
List:
- • The list is a class in C# which is similar to an array. But it is automatically adjust the size of the List object to store the items.
- • It is possible to delete the items in list like adding items.
- • An object of List automatically shrinks when items are deleted from it.
- • It helps to store and retrieve the items.
- • The format to declare a list is given below:
List<dataType> nameList= new List <dataType>();
- • Where,
- ○ “List” indicates the class.
- ○ “nameList” indicates an object of List class.
- ○ “dataType” indicates the type of data such “int”, “double”, “string”, and so on.
List initialization:
It is optional to initialize an object of List. Consider the following example,
List<int> numList= new List <int>(){1, 2, 3, 4, 5};
Explanation:
Here, the statement creates an object of List with the name of “numList” which hold the integer values “1, 2, 3, 4, 5”.
Adding the items into List:
The items are added to an existing List by using the Add() method.
The format to add the items to list is given below:
nameList.Add(items);
- • Where,
- ○ “Add” is keyword to add the items into List.
- ○ “nameList” indicates an object of List class.
- ○ “items” indicates the value to add into list and it can be in any data type such as “int”, “double”, “string”, and so on.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What happens when you remove the entry from position 4 from a List?
Select one:
a. All of these
b. The entry in the 4th position is returned (if there was a 4th entry)
c. If the List has 3 or fewer entries, an IndexOutOfBoundsException is thrown
d. If the List has 5 or more entries, the entries in positions 5 and up will move up
1 spot earlier to close up the gap vacated by removing the 4th entry
You are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework.
The “data.txt” file has three lines of data
100, 110, 120, 130, 140, 150, 160
100, 130, 160
1@0, 2@3, 3@END
You need to
1. create an empty unsorted list
2. add the numbers from the first line to list using putItem() function.
Then print all the current keys to command line in one line using printAll().
3. delete the numbers given by the second line in the list by using deleteItem() function.
Then print all the current keys to command line in one line using printAll()..
4. putItem () the numbers in the third line of the data file to the corresponding location in
the list. For example, 1@0 means adding number 1 at position 0 of the list.
Then print all the current keys to command line in one…
1. Write a Java program to create a new array list, add some colors (string) and print out the collection. The list should contain color "green".
2. Change the previous program to insert an element into the array list at the first position.
Change the application to print out the index of color "green".
Change the previous application to replace "green" value with "yellow".
Change the previous application to remove the third element from the list.
Change the application to sort the list of colors.
Change the application to shuffle the list of colors.
Change the application such that it swaps colors "Orange" and "Red".
Change the application to close the current list into a new list.
Change the application to trim the capacity of the initial array list to the current list size.
Change the application to empty the second list.
Change the application to test (print out a message) if the second list is empty.
Change the application to increase the capacity of…
Chapter 7 Solutions
Starting Out With Visual C# (5th Edition)
Ch. 7.1 - Prob. 7.1CPCh. 7.1 - What is the difference in the way you work with...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Is a variable of the Random class a reference type...Ch. 7.2 - Prob. 7.5CPCh. 7.2 - Prob. 7.6CPCh. 7.2 - Prob. 7.7CPCh. 7.2 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.4 - Prob. 7.11CPCh. 7.4 - Prob. 7.12CPCh. 7.4 - Prob. 7.13CPCh. 7.6 - Prob. 7.14CPCh. 7.6 - Prob. 7.15CPCh. 7.6 - Prob. 7.16CPCh. 7.7 - Prob. 7.17CPCh. 7.7 - Write a statement that assigns the value 50 to the...Ch. 7.7 - Prob. 7.19CPCh. 7.8 - Prob. 7.20CPCh. 7.8 - Write a statement that declares a jagged array of...Ch. 7.9 - Write a statement that initializes a List with 4...Ch. 7.9 - Prob. 7.23CPCh. 7.9 - Write a statement that clears the contents of the...Ch. 7.9 - Prob. 7.25CPCh. 7 - The memory that is allocated for a_______ variable...Ch. 7 - A variable that is used to reference an object is...Ch. 7 - When you want to work with an object, you use a...Ch. 7 - The_______ creates an object in memory and returns...Ch. 7 - A(n) is an object that can hold a group of values...Ch. 7 - The indicates the number of values that the array...Ch. 7 - Prob. 7MCCh. 7 - Prob. 8MCCh. 7 - When you create an array, you can optionally...Ch. 7 - Prob. 10MCCh. 7 - A(n)________ occurs when a loop iterates one time...Ch. 7 - C# provides a special loop that, in many...Ch. 7 - The foreach loop is designed to work with a...Ch. 7 - is a process that periodically runs, removing all...Ch. 7 - Various techniques known as_______ have been...Ch. 7 - Prob. 16MCCh. 7 - A(n) is a type of assignment operation that copies...Ch. 7 - Prob. 18MCCh. 7 - Prob. 19MCCh. 7 - The .NET Framework provides a class named_______,...Ch. 7 - When you are working with a value type, you are...Ch. 7 - Reference variables can be used only to reference...Ch. 7 - Individual variables are well suited for storing...Ch. 7 - Arrays are reference type objectsCh. 7 - Prob. 5TFCh. 7 - When you create a numeric array in C#, its...Ch. 7 - Prob. 7TFCh. 7 - You use the == operator to compare two array...Ch. 7 - Prob. 9TFCh. 7 - Prob. 10TFCh. 7 - How much memory is allocated by the compiler when...Ch. 7 - What type of variable is needed to work with an...Ch. 7 - What two steps are typically required for creating...Ch. 7 - Are variables well suited for processing lists of...Ch. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Prob. 10SACh. 7 - Assume names is a variable that references an...Ch. 7 - Prob. 2AWCh. 7 - Write code for a sequential search that determines...Ch. 7 - Prob. 4AWCh. 7 - Prob. 5AWCh. 7 - Create a List object that uses the binary search...Ch. 7 - Total Sales In the Chap07 folder of the Student...Ch. 7 - Sales Analysis Modify the application that you...Ch. 7 - Charge Account Validation In the Chap07 folder of...Ch. 7 - Drivers License Exam The local drivers license...Ch. 7 - World Series Champions In the Chap07 folder of the...Ch. 7 - Name Search In the Chap07 folder of the Student...Ch. 7 - Population Data In the Chap07 folder of the...Ch. 7 - Tic-Tac-Toe Simulator Create an application that...Ch. 7 - Jagged Array of Exam Scores Dr. Hunter teaches...
Knowledge Booster
Similar questions
- Exercise 1• Create a class that sorts a list in ascending order. Make use of the Collections method sort• The list should be a list of Strings.• Test your code with {“Hearts”, “Diamonds”, “Clubs”, “Spades”}• Before and after the sort, use the implicit call to the list’s toString method to output the list contents. Exercise 2• Create a class with the same requirement as in Exercise 1, but the sort should be in descending order• Make use of the Comparator interface• Make use of the static collection method reverseOrder javaarrow_forward***NEED HELP WITH THIS**** write a code that replicates the list's insert() method. Recall that insert(index, item) method inserts the item at specified index in the list. Write a code that: Asks the user to input 5 items in a list and display the list. Ask the user to input the index where they would like to insert a new item. Ask the user to input the item that they would like to insert into the list. Inserts the new item into the list and displays the modified list. If the index is greater than the length of the list, simply insert the new item at the end of list. Also i dont need to use the list's insert() method to do this program. I dont need to write a function, just the code to replicate the functionality of insert() method.arrow_forwardof range. 3. Use the method swap that you wrote in Exercise 2 to write a method that reverses the order of the items in a list alist.arrow_forward
- 1. Write a Java program to create a new array list, add some colors (string) and print out the collection. The list should contain color "green". 2. Change the previous program to insert an element into the array list at the first position. 3. Change the application to print out the index of color "green". 4. Change the previous application to replace "green" value with "yellow". 5. Change the previous application to remove the third element from the list. 6. Change the application to sort the list of colors. 7. Change the application to shuffle the list of colors. 8. Change the application such that it swaps colors "Orange" and "Red". 9. Change the application to copy the current list into a new list. 10. Change the application to trim the capacity of the initial array list to the current list size. 11. Change the application to empty the second list. 12. Change the application to test (print out a message) if the second list is empty. 13. Change the application to increase the…arrow_forwardScrabble Help: The file dictionary.txt contains all of the words in the Official Scrabble Player's Dictionary, Second Edition. Note: this list contains some offensive language. Write a class, WordLists, in Java that generates useful word lists for scrabble players using this list. Your class should contain the following methods: WordLists(String fileName): a constructor that takes the name of the dictionary file as the only parameter. lengthN(int n): returns an ArrayList of all length n words (Strings) in the dictionary file. endsWith(char lastLetter, int n): returns an ArrayList of words of length n ending with the letter lastLetter containsLetter(char included, int index, int n): returns an ArrayList of words of length n containing the letter included at position index. So for example the word "cannon" would be on the list returned by containsLetter('o',6,4) because it contains the letter 'o', at index 4, and is length 6. multiLetter(int m, char included): returns an ArrayList of…arrow_forward1. The codewords will be scrambled words. You will read the words used for the codes from a file and store it into an array. There are 60 words in the file that range in size from 3 characters to 7 characters. The file is called wordlist.txt and can be found attached to the assignment in IvyLearn.2. Start with input for an integer seed for the random number generator. There is no need to put a prompt before the cin operation.3. Ask the Player if they are ready to play and only proceed if they type a Y or y. If the user types an N or n then the program should finish. a. INPUT VALIDATION: Make sure the user has typed either y, Y, n, or N4. Create a variable to keep track of the number of guesses the user has made.5. Use the random number generator to pick a word from the list of words.6. Once you have chosen a word as the codeword you will need to scramble the letters to make it into a code. You should use the random number generator to help you mix up the letters.7. Display to the user…arrow_forward
- This will happen if you try to use an index that is out of range for a list.a. A ValueError exception will occur.b. An IndexError exception will occur.c. The list will be erased and the program will continue to run.d. Nothing—the invalid index will be ignored.arrow_forwardAdd the following functions and write a program to test these functions in the class linkedListType: a. Write the definition of a function that returns the data of the kth element of the linked list. If such element is not exist in the list, exit the program. b. Write the definition of a function that deletes the kth element of the linked list. If such element is not exist in the list, exit the program and display message as output. (Subject:Data stracture and algorithm )arrow_forwardAdd the following functions and write a program to test these functions in the class linkedListType: a. Write the definition of a function that returns the data of the kth element of the linked list. If such element is not exist in the list, exit the program. b. Write the definition of a function that deletes the kth element of the linked list. If such element does not exist in the list, exit the program and display message as output.arrow_forward
- a. Create a Main class "CheckList. Inside the main method of the Main class create n number of objects of the List class. For each of the objects perform 5 input operations using the push method. b. Now create a static method inside the Main class " Boolean CompareTo(List 11, List 12)" which will take any two objects of list type and return true if all the elements of the two objects are the same and return false otherwise. c. Now create a static method inside the Main class " Boolean Equals (List 11, List 12)" which will take any two objects of list type and return true if the size of the two objects are the same and return false otherwise. d. Before executing the operation mentioned in 3(a), if we declare Count variable of the List class as a static variable then what will be the impact of these two objects? Explain with your own words. e. Is it possible to call the List (int MaxSize) constructor from the List()constructor? If yes then please write that specific part of the code…arrow_forwardWrite a statement that creates a two-dimensional list with 5 rows and 3 columns. Then writenested loops that get an integer value from the user for each element in the list.arrow_forwardPython write a program in python that plays the game of Hangman. When the user plays Hangman, the computer first selects a secret word at random from a list built into the program. The program then prints out a row of dashes asks the user to guess a letter. If the user guesses a letter that is in the word, the word is redisplayed with all instances of that letter shown in the correct positions, along with any letters correctly guessed on previous turns. If the letter does not appear in the word, the user is charged with an incorrect guess. The user keeps guessing letters until either: * the user has correctly guessed all the letters in the word or * the user has made eight incorrect guesses. one for each letter in the secret word and Hangman comes from the fact that incorrect guesses are recorded by drawing an evolving picture of the user being hanged at a scaffold. For each incorrect guess, a new part of a stick-figure body the head, then the body, then each arm, each leg, and finally…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
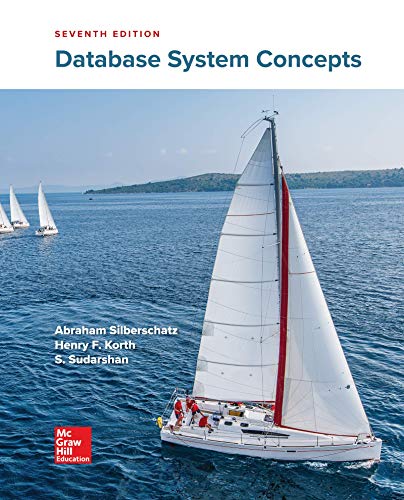
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
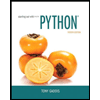
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
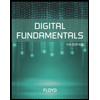
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
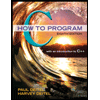
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
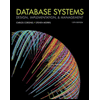
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
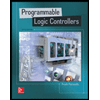
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education