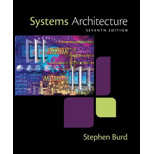
Systems Architecture
7th Edition
ISBN: 9781305080195
Author: Stephen D. Burd
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 3, Problem 1PE
Program Plan Intro
Inserting an element to linked list after the address of the element
Program Plan:
- Include required header files.
- Create a structure for “Node”.
- Declare a variable “element” to store the numeric values.
- Declare a pointer “next” to store the address of the next link.
- Define the function “insert_in_linked_list()”.
- Declare a local pointer “ptr”.
- Assign value for “ptr”.
- Assign memory space for new node and place data value in it.
- Link the new node.
- Place the element in the list.
- Link new node to the list.
- Define the function “insert_element()” to add an element to the end of the list.
- Iterate the “while” loop until last node becomes “null”.
- Move pointer “ptr” to the pointer next node.
- Assign memory allocation for the new node.
- Create the new assigned memory space to the pointer “ptr”.
- Place the element in the list.
- Set “NULL” to the mark the end of the list.
- Move pointer “ptr” to the pointer next node.
- Iterate the “while” loop until last node becomes “null”.
- Define the function “display_list()” to display all elements in the list.
- If the “ptr” is null, then returns null.
- Print the element in the list.
- Call the function “display_list()” recursively with next element of the list.
- Define main() function.
- Declare required variables.
- Declare pointer objects using structure.
- Assign memory allocation for first element of list.
- Set next of first element to “NULL”.
- Use “for” loop to iterate 10 elements in the list.
- Call the function “insert_element()” to insert each element in the list.
- Call the function “display_list()” to display the elements in the list.
- Read the element from user.
- Read the index of new element from the user.
- Set the temp pointer.
- Use “for” loop to search for index value entered by the user.
- Check condition and if the condition satisfies, then store the address in the pointer “temp_ptr”.
- Call the function “insert_in_linked_list” with “add_element” and “temp_ptr”.
- Call the function “display_list()” to display the elements of the list.
- Call the function “insert_element()” to insert each element in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Data structures
concatenate_dict(dict_list:list)->dict
This function will be given a single parameter known as the Dictionary List. Your job is to combine all the dictionaries found in the dictionary list into a single dictionary and return it. There are two rules for adding values to the dictionary:
1. You must add key-value pairs to the dictionary in the same order they are found in the Dictionary List.
2. If the key already exists, it cannot be overwritten. In other words, if two or more dictionaries have the same key, the key to be added cannot be overwritten by the subsequent dictionaries.
Example:
Dictionary List: [{'Z': 6, 'k': 10, 'w': 3, 'I': 8, 'Y': 5}, {'Y': 1, 'Z': 4}, {'X': 2, 'L': 5}]
Expected: {'Z': 6, 'k': 10, 'w': 3, 'I': 8, 'Y': 5, 'X': 2, 'L': 5}
Dictionary List: [{'z': 0}, {'z': 7}]
Expected: {'z': 0}
Dictionary List: [{'b': 7}, {'b': 10, 'A': 8, 'Z': 2, 'V': 1}]
Expected: {'b': 7, 'A': 8, 'Z': 2, 'V': 1}
in c++
Create a single linked list that contains the data (age) of your friends. Perform basic operations including insertion, deletion, searching and display. The insertion operation should only allow a friend’s data to be inserted in sorted order only.
Using user-defined function, create a C++ or Java application implementing a linked list datastructure. The application should be able to perform the following operations on the datastructure can with the following operations. i. insertion into the listii. deletion from the list iii. printing the content of the list
Chapter 3 Solutions
Systems Architecture
Ch. 3 - Prob. 1VECh. 3 - Prob. 2VECh. 3 - A(n) __________ is an integer stored in double the...Ch. 3 - Prob. 4VECh. 3 - Assembly (machine) language programs for most...Ch. 3 - Prob. 6VECh. 3 - Prob. 7VECh. 3 - Prob. 8VECh. 3 - Prob. 9VECh. 3 - A(n) __________ is an array of characters.
Ch. 3 - Most Intel CPUs use the __________, in which each...Ch. 3 - Prob. 12VECh. 3 - A(n) __________ contains 8 __________.Ch. 3 - Prob. 14VECh. 3 - The result of adding, subtracting, or multiplying...Ch. 3 - Prob. 16VECh. 3 - Prob. 17VECh. 3 - Prob. 18VECh. 3 - Prob. 19VECh. 3 - Prob. 20VECh. 3 - Prob. 21VECh. 3 - Prob. 22VECh. 3 - ___________ occurs when the result of an...Ch. 3 - In a CPU, _______ arithmetic generally is easier...Ch. 3 - In the ________, memory addresses consist of a...Ch. 3 - Prob. 26VECh. 3 - Data represented in ________ is transmitted...Ch. 3 - Prob. 28VECh. 3 - Prob. 29VECh. 3 - A(n) ____________ is one instance or variable of a...Ch. 3 - Prob. 1RQCh. 3 - Why is binary data representation and signaling...Ch. 3 - Prob. 3RQCh. 3 - Prob. 4RQCh. 3 - Prob. 5RQCh. 3 - Prob. 6RQCh. 3 - Prob. 7RQCh. 3 - Why doesnt a CPU evaluate the expression 'A' = 'a'...Ch. 3 - Prob. 9RQCh. 3 - What primitive data types can normally be...Ch. 3 - Prob. 11RQCh. 3 - How is an array stored in main memory? How is a...Ch. 3 - Prob. 14RQCh. 3 - Prob. 1PECh. 3 - Prob. 2PECh. 3 - Prob. 4PECh. 3 - Prob. 5PECh. 3 - Prob. 6PECh. 3 - Prob. 1RPCh. 3 - Prob. 2RPCh. 3 - Prob. 3RP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- How is an array stored in main memory? How is a linked list stored in main memory? What are their comparative advantages and disadvantages? Give examples of data that would be best stored as an array and as a linked list.arrow_forwardLINKED LIST IMPLEMENTATION Subject: Data Structure and Algorithm in C++Create a Student Record Management system that can perform the following operations:1) Insert student records2) Delete student record3) Show student record4) Search student record The student record should contain the following items1) Name of Student2) Student Matriculation ID number3) Course in which the student is enrolled4) Total marks of the student Approach: With the basic knowledge of operations of Linked Lists like insertion, deletion of elements in linked list, the student record management can be created. Below are the functionalities explained that are to be implemented.●Check Record: It is a utility function of creating a record it checks before insertion that the Record Already exist or not. It uses the concept of checking for a Node with given Data in a linked list.-Create Record: It is as simple as creating a new node in the Empty Linked list or inserting a new node in a non-Empty linked list.-Search…arrow_forwardnumList: head: tail: data: 73 next: data: 37 next: data: 85 next: null After List Prepend(numList, node 58), determine the following values. Enter null if the pointer is null. numList's head pointer points to node Ex: 5 or null numList's tail pointer points to node node 58's next pointer points to node node 85's next pointer points to nodearrow_forward
- C++ PROGRAM DATA STRUCTURES Write a function to swap nodes in a Doubly Linked list.arrow_forwardContext and Problem Statement: A web search engine returns you a list ofweb-links that match the set of words in your query. Each web-link is assignedan integer for identification purposes(page-ID) This is done by maintaining an”inverted index”.An inverted index, at a very elementary level, is a mapping that takes a wordand returns to you a list of web-links which contains the word.That list wouldbe a sorted(ranked) array of page-IDs where the web-link with the highest rankfor the query is displayed on top. When your query to the web search enginecontains multiple words, the search engine needs to find the sorted array for eachword and then computes the intersection of these arrays. Given the number ofweb-pages existing in the world-wide-web, this work is very computationallychallenging !In this assignment you are required to write programs which takes as inputtwo sorted arrays and returns a new array containing the elements found inboth the sorted arrays. It is alright if the input…arrow_forwardASSUMING C LANGUAGE True or False: You can have the data portion of a Linked List be a Struct containing a Linked List itselfarrow_forward
- C Programming Language If you have the following node declaration:Homework 3struct Node {int number;struct Node * next;};typedef struct Node node;node *head,*newNode;Write a C program that contains the following functions to manipulate this linked list :1. First function: Adding the odd numbers to the beginning of the list and even numbers to theend of the list until -1 is entered from keyboard.2. Second function using given prototype below. This function cuts the last node of the list andadds it to the beginning as first node. It takes beginning address of the list as a parameter andreturns the updated list.node* cutlastaddhead(node* head);3. 3rd function deletes the element in the middle of the list (free this memory location) (if the listhas 100 or 101 elements, it will delete the 50th element). The function will take a list as aparameter and return the updated list.4. 4th function named changeFirstAndLast that swaps the node at the end of the list and thenode at the beginning of…arrow_forwardWhen a new node is inserted into an array based list at an index, which of the following is FALSE? Select one: A.All elements from the index to the end of the list will be shifted to one higher value B.The size of the array will be increased to accommodate the new element C.The new element will be placed in the array at the next position after the specified index D.The element at the index position will be replaced with the newly inserted elementarrow_forwardData Structures , Code C++ Suppose that p, q, and r are all pointers to nodes in a linked list with 15 nodes. The pointer p points to the first node, q points to the 8th node, and r points to the last node. Write a few lines of code that will make a new copy of the list. You code should set THREE new pointers called x, y, and z so that: x points to the first node of the copy, y points to the 8th node of the copy, and z points to the last node of the copy.arrow_forward
- head = (node *)malloc(sizeof(node)); which returns a pointer to a structure of type node that has been type defined earlier. The linked list is then created by the function create. The function requests for the number to be placed in the current node that has been created. If the value assigned to the current node is -999, then null is assigned to the pointer variable next and the list ends. Otherwise, memory space is allocated to the next node using again the malloc function and the next value is placed into it. Not that the function create calls itself recursively and the process will continue until we enter the number -999. The items stored in the linked list are printed using the function print which accept a pointer to the current node as an argument. It is a recursive function and stops when it receives a NULL pointer. Printing algorithm is as follows; 1. Start with the first node. 2. While there are valid nodes left to print a) print the current item and b) advance to next node…arrow_forwardIn C, members (fields) of different structures can have the same name. True Falsearrow_forwardDATA STRUCTURES C++ C++ PROGRAM Create a doubly circular linked list using circular link list implementation. Write a function in doubly Linked List class to find the minimum value in list. Swap values of any two nodes given by user from doubly linked list and show the result. You have to paste code and screenshots of all tasks.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
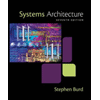
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
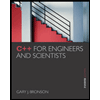
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
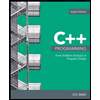
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning