Write the following generic method using selection sort and a comparator: public static void selectionSort(E[] list, Comparator comparator) Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator introduced in Listing 20.5 to sort the elements. Display the sorted elements. Use the following statement to create the array: GeometricObject[] list1 = {new Circle(5), new Rectangle(4, 5), new Circle(5.5), new Rectangle(2.4, 5), new Circle(0.5), new Rectangle(4, 65), new Circle(4.5), new Rectangle(4.4, 1), new Circle(6.5), new Rectangle(4, 5)}; Also in the same program, write the code that sorts six strings by their last character. Use the following statement to create the array: String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"}; GeometricObjects import java.util.Comparator; public class GeometricObjectComparator implements Comparator, java.io.Serializable { public int compare(GeometricObject o1, GeometricObject o2) { return o1.getArea() > o2.getArea() ? 1 : o1.getArea() == o2.getArea() ? 0 : -1; } } GeometricObjectComparator public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } @Override public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); } I'm getting an error on my program.
Write the following generic method using selection sort and a comparator: public static void selectionSort(E[] list, Comparator comparator) Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator introduced in Listing 20.5 to sort the elements. Display the sorted elements. Use the following statement to create the array: GeometricObject[] list1 = {new Circle(5), new Rectangle(4, 5), new Circle(5.5), new Rectangle(2.4, 5), new Circle(0.5), new Rectangle(4, 65), new Circle(4.5), new Rectangle(4.4, 1), new Circle(6.5), new Rectangle(4, 5)}; Also in the same program, write the code that sorts six strings by their last character. Use the following statement to create the array: String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"}; GeometricObjects import java.util.Comparator; public class GeometricObjectComparator implements Comparator, java.io.Serializable { public int compare(GeometricObject o1, GeometricObject o2) { return o1.getArea() > o2.getArea() ? 1 : o1.getArea() == o2.getArea() ? 0 : -1; } } GeometricObjectComparator public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } @Override public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); } I'm getting an error on my program.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the following generic method using selection sort and a comparator:
public static <E> void selectionSort(E[] list, Comparator<? super E> comparator)
Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator introduced in Listing 20.5 to sort the elements.
Display the sorted elements. Use the following statement to create the array:
GeometricObject[] list1 = {new Circle(5), new Rectangle(4, 5),
new Circle(5.5), new Rectangle(2.4, 5), new Circle(0.5),
new Rectangle(4, 65), new Circle(4.5), new Rectangle(4.4, 1),
new Circle(6.5), new Rectangle(4, 5)};
new Circle(5.5), new Rectangle(2.4, 5), new Circle(0.5),
new Rectangle(4, 65), new Circle(4.5), new Rectangle(4.4, 1),
new Circle(6.5), new Rectangle(4, 5)};
Also in the same program, write the code that sorts six strings by their last character. Use the following statement to create the array:
String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"};
GeometricObjects
import java.util.Comparator; public class GeometricObjectComparator implements Comparator<GeometricObject>, java.io.Serializable { public int compare(GeometricObject o1, GeometricObject o2) { return o1.getArea() > o2.getArea() ? 1 : o1.getArea() == o2.getArea() ? 0 : -1; } }
GeometricObjectComparator
public abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated; /** Construct a default geometric object */ protected GeometricObject() { dateCreated = new java.util.Date(); } /** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; } /** Return color */ public String getColor() { return color; } /** Set a new color */ public void setColor(String color) { this.color = color; } /** Return filled. Since filled is boolean, * the get method is named isFilled */ public boolean isFilled() { return filled; } /** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; } /** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; } @Override public String toString() { return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled; } /** Abstract method getArea */ public abstract double getArea(); /** Abstract method getPerimeter */ public abstract double getPerimeter(); }
I'm getting an error on my program.
![1 package. GeometricObject; g
2 x
30 import java.util.Arrays;
4 import java.util.Comparator;
5 ¤¶
6 import application. GeometricObject;
7 import javafx.scene.shape.Circle;
8 import javafx.scene.shape. Rectangle;
9 import javafx.scene.paint.Color;
10 import javafx.scene.text. Font;
11 import javafx.scene.text.Text;
12 import javafx.application. Application;
13 import javafx.scene. Scene;
14 import javafx.scene.layout.Pane;
15 import javafx.stage.Stage;
16 ¤¶
17 ¤¶
18 public class Test Program {9
190
20
21
22
23
24
25
26
27
28
29
x30
31
32 ¤¶
33
34
35 ¤
36
37
38
39
40 ¤¶
41
42
public static void main(String[] args) {
GeometricObject[] list1= {9
new Circle(5), J
new Rectangle(45), ¤¶
new Circle(5.5),
new Rectangle(2.4.5),
new Circle(0.5),
new Rectangle(465), ¤¶
new Circle(4.5),
new Rectangle(441),
new Circle(6.5), ¤¶
new Rectangle(4, 5).
·};*9
.//.Sort the array using selection sort and the GeometricObjectComparator*g
selectionSort(list1, new GeometricObject());
.// Display the sorted elements
for (GeometricObject obj: list1). {9
System.out.println(obj);
}¤¶
String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"};](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc4b4111f-0cb3-46a3-bff1-74a667fd5dd6%2Fc0cacdaa-3f9b-46af-a025-2c43000c3f37%2Frrrgt7ol_processed.png&w=3840&q=75)
Transcribed Image Text:1 package. GeometricObject; g
2 x
30 import java.util.Arrays;
4 import java.util.Comparator;
5 ¤¶
6 import application. GeometricObject;
7 import javafx.scene.shape.Circle;
8 import javafx.scene.shape. Rectangle;
9 import javafx.scene.paint.Color;
10 import javafx.scene.text. Font;
11 import javafx.scene.text.Text;
12 import javafx.application. Application;
13 import javafx.scene. Scene;
14 import javafx.scene.layout.Pane;
15 import javafx.stage.Stage;
16 ¤¶
17 ¤¶
18 public class Test Program {9
190
20
21
22
23
24
25
26
27
28
29
x30
31
32 ¤¶
33
34
35 ¤
36
37
38
39
40 ¤¶
41
42
public static void main(String[] args) {
GeometricObject[] list1= {9
new Circle(5), J
new Rectangle(45), ¤¶
new Circle(5.5),
new Rectangle(2.4.5),
new Circle(0.5),
new Rectangle(465), ¤¶
new Circle(4.5),
new Rectangle(441),
new Circle(6.5), ¤¶
new Rectangle(4, 5).
·};*9
.//.Sort the array using selection sort and the GeometricObjectComparator*g
selectionSort(list1, new GeometricObject());
.// Display the sorted elements
for (GeometricObject obj: list1). {9
System.out.println(obj);
}¤¶
String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"};
![Write the following generic method using selection sort and a comparator:
public static <E> void selectionSort (E[] list, Comparator<? super E> comparator)
Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator introduced in Listing 20.5 to sort the elements. Display the sorted elements. Use
the array:
GeometricObject[] list1 = {new Circle (5), new Rectangle(4, 5),
new Circle (5.5), new Rectangle (2.4, 5), new Circle(0.5),
new Rectangle(4, 65), new Circle(4.5), new Rectangle (4.4, 1),
new Circle(6.5), new Rectangle(4, 5)};
Also in the same program, write the code that sorts six strings by their last character. Use the following statement to create the array:
String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"};
following statement to create](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc4b4111f-0cb3-46a3-bff1-74a667fd5dd6%2Fc0cacdaa-3f9b-46af-a025-2c43000c3f37%2Fvpdqwoo_processed.png&w=3840&q=75)
Transcribed Image Text:Write the following generic method using selection sort and a comparator:
public static <E> void selectionSort (E[] list, Comparator<? super E> comparator)
Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator introduced in Listing 20.5 to sort the elements. Display the sorted elements. Use
the array:
GeometricObject[] list1 = {new Circle (5), new Rectangle(4, 5),
new Circle (5.5), new Rectangle (2.4, 5), new Circle(0.5),
new Rectangle(4, 65), new Circle(4.5), new Rectangle (4.4, 1),
new Circle(6.5), new Rectangle(4, 5)};
Also in the same program, write the code that sorts six strings by their last character. Use the following statement to create the array:
String[] list2 = {"red", "blue", "green", "yellow", "orange", "pink"};
following statement to create
Expert Solution

Step 1
Write the following generic method using selection sort and a comparator:
public static <E> void selectionSort(E[] list, Comparator<? super E> comparator)
Write a test program that creates an array of 10 GeometricObjects and invokes this method using the GeometricObjectComparator introduced in Listing 20.5 to sort the elements.
Display the sorted elements.
I have provided a solution in step 2 with a proper explanation.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
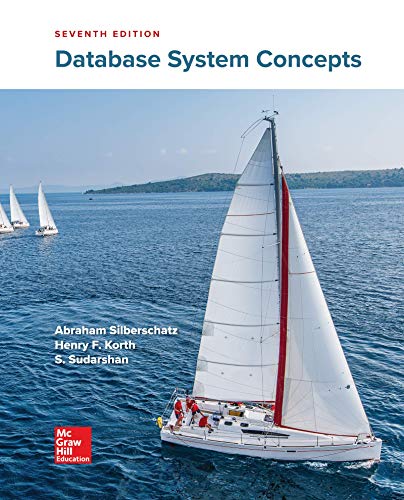
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
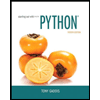
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
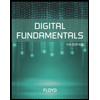
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
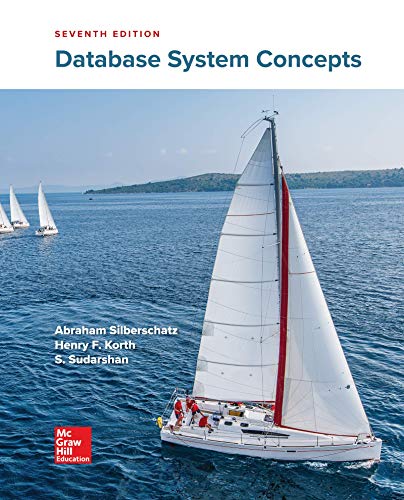
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
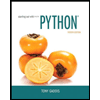
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
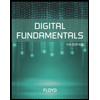
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
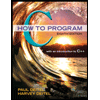
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
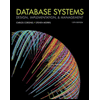
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
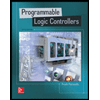
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education