Do not use static variables to implement recursive methods. USING JAVA: // P6 public static int countSubstrings(String s1, String s2) { } Write a recursive method countSubstrings that counts the number of non-overlapping occurrences of a string s2 in a string s1. Use the method indexOf() from String class. As an example, with s1=”abab” and s2=”ab”, countSubstrings returns 2. With s1=”aaabbaa” and s2=”aa”, countSubstrings returns 2. With s1=”aabbaa” and s2=”AA”, countSubStrings returns 0. Show the output on the following strings: s1 = “Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java applications are typically compiled to bytecode (class file) that can run on any Java Virtual Machine (JVM) regardless of computer architecture. Java is a general-purpose, concurrent, class-based, object-oriented language that is specifically designed to have as few implementation dependencies as possible. Java is currently one of the most popular programming languages in use, particularly for client-server web applications, with a reported 10 million users.” s2= “Java”.
Do not use static variables to implement recursive methods.
USING JAVA:
// P6
public static int countSubstrings(String s1, String s2) {
}
- Write a recursive method countSubstrings that counts the number of non-overlapping occurrences of a string s2 in a string s1. Use the method indexOf() from String class.
- As an example, with s1=”abab” and s2=”ab”, countSubstrings returns 2. With s1=”aaabbaa” and s2=”aa”, countSubstrings returns 2. With s1=”aabbaa” and s2=”AA”, countSubStrings returns 0.
Show the output on the following strings:
- s1 = “Java is a
programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java applications are typically compiled to bytecode (class file) that can run on any Java Virtual Machine (JVM) regardless of computer architecture. Java is a general-purpose, concurrent, class-based, object-oriented language that is specifically designed to have as few implementation dependencies as possible. Java is currently one of the most popular programming languages in use, particularly for client-server web applications, with a reported 10 million users.”
- s2= “Java”.

code : -
public class SubstringCounter {
public static int countSubstrings(String s1, String s2) {
// base case: s1 is empty or s2 is longer than s1
if (s1.isEmpty() || s2.length() > s1.length()) {
return 0;
}
// find the index of the first occurrence of s2 in s1
int index = s1.indexOf(s2);
// if s2 is not found in s1, return 0
if (index == -1) {
return 0;
}
// increment the count by 1 and recursively call countSubstrings
// on the substring of s1 that starts after the first occurrence of s2
// and the same s2 string
return 1 + countSubstrings(s1.substring(index + s2.length()), s2);
}
public static void main(String[] args) {
String s1 = "Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java applications are typically compiled to bytecode (class file) that can run on any Java Virtual Machine (JVM) regardless of computer architecture. Java is a general-purpose, concurrent, class-based, object-oriented language that is specifically designed to have as few implementation dependencies as possible. Java is currently one of the most popular programming languages in use, particularly for client-server web applications, with a reported 10 million users.";
String s2 = "Java";
int count = countSubstrings(s1, s2);
System.out.println("Number of non-overlapping occurrences of \"" + s2 + "\" in \"" + s1 + "\": " + count);
// second test case
s1="abab";
s2="ab";
count = countSubstrings(s1, s2);
System.out.println("\n\n\nNumber of non-overlapping occurrences of \"" + s2 + "\" in \"" + s1 + "\": " + count);
}
}
Step by step
Solved in 2 steps with 1 images

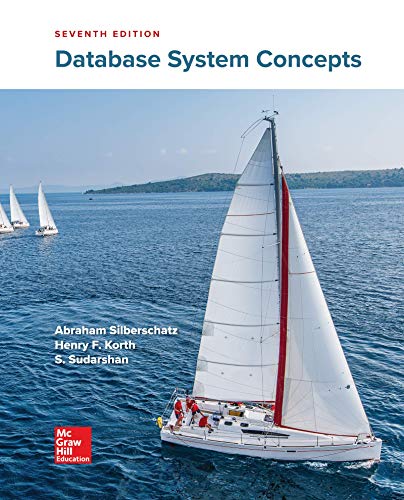
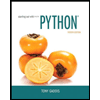
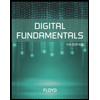
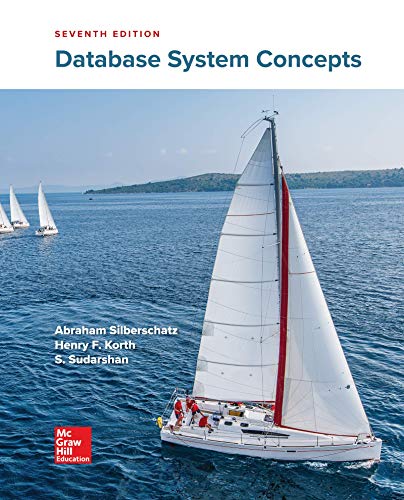
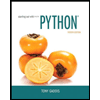
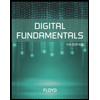
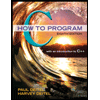
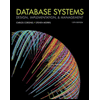
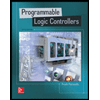