C++ Code Step 1: Preparation For the moment, "comment out" the following under-construction code: In dynamicarray.h: All function prototypes except the constructors and destructor. Keep the member variables (but we will be replacing them shortly). In dynamicarray.cpp: All function implementations except the constructors and destructor. You should also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away. In main: Comment out all the code inside the RunPart1Tests function between the lines bool pass = true; and return pass; • Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return 0;" line. Step 2: Replacing member data and the two constructors You're going to replace the current member data (arr, len, and capacity) with a single vector of integers. Remember that vectors keep track of their own size and capacity, so your new vector object will contain all of that information. At the top of your.h, include the library. This is the only place you should need to include it. Also in your h, remove your current member data, and replace it with a vector of ints. I recommend naming it "arr" so you can keep as much of your current code as possible. Next, you need to change the constructor and destructor. And it's going to be awesome: If you look at the code in your default constructor, there is no need to do any of that anymore. The default constructor for the STL vector class is already doing that for us. So your implementation is now {}. Technically, you could remove the default constructor from the class altogether, but you might as well be explicit about the fact that you don't need to do anything special. For the copy constructor, we need to copy the vector from the other object. One way to do so would be to do an element-by-element copy. But this poses a problem: how can we do this copying if the vector we are trying to copy to is not even constructed (is empty). Well, one answer is that we can resize the vector to be the same size as the other object and then do the element-by-element copy. The other is that we can use the copy constructor that's defined for the STL vector class through what is called a constructor initialization list (zyBooks section 9.12). Here's your new copy constructor in all its glory: DynamicArray: :DynamicArray(const DynamicArray& other) : arr(other.arr) { } The ": arr(other)" is the initialization list. It specifies how to construct each of the class's member variables and uses the copy constructor of the STL vector class. For the destructor, we have to deallocate any memory we dynamically allocated. We're not doing that anymore! When our member variables get deallocated (which happens automatically), the STL vector class's destructor will do all of that for us too. So the destructor is also now { }. Step 3: The one-liners plus output Next, you'll tackle four member functions (cap(), at(), append() and operator=) that translate very well to functions that the vector class provides for you. You'll also tackle print, since you'll need it to check your results. This reference page for the vector::capacity.) E function actually has an example covering most of we need. The remainder is in the list of member functions at the bottom of this page E; you can click on each one to get examples. Here's what to do: Uncomment the declarations and definitions of these functions from the .h and .cpp files, respectively. Replace your cap() function (defined in the h) with a single-line function that uses a member function of the vector class. Do the same for your at() function. Do NOT return -11111 when out-of-bounds. It's OK if your code throws an exception (which will cause a crash) for out-of-bounds errors. Do the same for your append() function. Repeat for the = operator. This will be two lines: one to actually copy the vector, and then the obligatory return * this; At this point, you should check that your code is free of compiler errors (which is the option Build->Build Project from the menu). Next, it's time to look at the output function: print(. It makes reference to two member variables: arrli] and len. Replace these references with the appropriate ways to access them using your new vector. When you're ready to test your code, go to the RunPart1Tests function in main.cpp. Put everything back in until the line labeled "Test sum." Two bad things are going to happen: You're going to fail two tests. You can comment out the sections that test the capacity THIS CODE SHOULD CRASH, with an error message including: terminate called after throwing an instance of 'std::out_of_range: vector'
C++ Code
Step 1: Preparation
For the moment, "comment out" the following under-construction code:
- In dynamicarray.h: All function prototypes except the constructors and destructor. Keep the member variables (but we will be replacing them shortly).
- In dynamicarray.cpp: All function implementations except the constructors and destructor. You should also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away.
- In main: Comment out all the code inside the RunPart1Tests function between the lines
bool pass = true;
and
return pass;
• Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return 0;" line.
Step 2: Replacing member data and the two constructors
You're going to replace the current member data (arr, len, and capacity) with a single vector of integers. Remember that
- At the top of your.h, include the <vector> library. This is the only place you should need to include it.
- Also in your h, remove your current member data, and replace it with a vector of ints. I recommend naming it "arr" so you can keep as much of your current code as possible.
Next, you need to change the constructor and destructor. And it's going to be awesome:
If you look at the code in your default constructor, there is no need to do any of that anymore. The default constructor for the STL vector class is already doing that for us. So your implementation is now {}. Technically, you could remove the default
constructor from the class altogether, but you might as well be explicit about the fact that you don't need to do anything special.
- For the copy constructor, we need to copy the vector from the other object. One way to do so would be to do an element-by-element copy. But this poses a problem: how can we do this copying if the vector we are trying to copy to is not even constructed (is empty). Well, one answer is that we can resize the vector to be the same size as the other object and then do the element-by-element copy. The other is that we can use the copy constructor that's defined for the STL vector class through what is called a constructor initialization list (zyBooks section 9.12). Here's your new copy constructor in all its glory:
DynamicArray: :DynamicArray(const DynamicArray& other) : arr(other.arr) { }
The ": arr(other)" is the initialization list. It specifies how to construct each of the class's member variables and uses the copy constructor of the STL vector class. - For the destructor, we have to deallocate any memory we dynamically allocated. We're not doing that anymore! When our member variables get deallocated (which happens automatically), the STL vector class's destructor will do all of that for us too. So the destructor is also now { }.
Step 3: The one-liners plus output
Next, you'll tackle four member functions (cap(), at(), append() and operator=) that translate very well to functions that the vector class provides for you. You'll also tackle print, since you'll need it to check your results.
This reference page for the vector::capacity.) E function actually has an example covering most of we need. The remainder is in the list of member functions at the bottom of this page E; you can click on each one to get examples.
Here's what to do:
- Uncomment the declarations and definitions of these functions from the .h and .cpp files, respectively.
- Replace your cap() function (defined in the h) with a single-line function that uses a member function of the vector class.
- Do the same for your at() function. Do NOT return -11111 when out-of-bounds. It's OK if your code throws an exception (which will cause a crash) for out-of-bounds errors.
- Do the same for your append() function.
- Repeat for the = operator. This will be two lines: one to actually copy the vector, and then the obligatory return * this;
At this point, you should check that your code is free of compiler errors (which is the option Build->Build Project from the menu).
Next, it's time to look at the output function: print(. It makes reference to two member variables: arrli] and len. Replace these references with the appropriate ways to access them using your new vector.
When you're ready to test your code, go to the RunPart1Tests function in main.cpp. Put everything back in until the line labeled "Test sum."
Two bad things are going to happen:
- You're going to fail two tests. You can comment out the sections that test the capacity
- THIS CODE SHOULD CRASH, with an error message including:
terminate called after throwing an instance of 'std::out_of_range: vector'

Step by step
Solved in 4 steps with 5 images

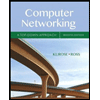
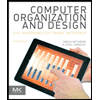
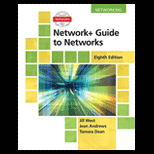
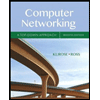
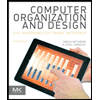
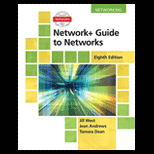
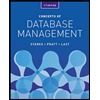
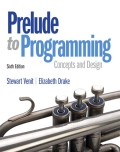
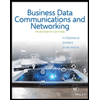