You have become the master of making peach cobbler. The recipe you follow is simple: 22 ounces of peaches in heavy syrup 1 regular yellow cake mix 3/8 cup melted butter The biggest problem is making sure you have enough ingredients. The above recipe for one batch serves 9. The cobbler is always made in the same batch size. There are no partial batches. Having some left over is ok, but being short is not. It's also ok to have some peaches and some butter left over. Create a program that will ask the user to enter the number of servings desired. Then generate the shopping list for all ingredients. Also calculate the leftover ingredients and the leftover cobbler Peaches come in cans of 29 ounces and 15 ounces Butter comes in packages of 1 pound (2 cups). Use a separate method to calculate and return the number of cans of peaches to buy. Use a separate method to calculate and return the boxes of butter. Output should look similar to the following. Spacing may vary, but make things line up! Fill in the numbers for the hashtags. USE VARIABLES. GROCERY LIST FOR <#> BATCHES OF COBBLER 29 ounce cans peaches 15 ounce cans peaches # # # # # # Yellow cake mixes # # pounds of butter QUANTITIES USED # # ounces of peaches used # # left over ##. # cups of butter used # #.# left over LEFTOVER COBBLER There should be <##> servings of cobbler left over, but don't count on it!


import java.util.Scanner;
public class MasterChef {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int servings = sc.nextInt(), servingsPerBatch = 9;
int batches = (int) Math.ceil((double) servings / servingsPerBatch);
int noOfYellowCakes = batches;
int noOfBoxesOfButter = boxesOfButter(batches);
int noOf29OuncesPeachBoxes = noOf29OuncesCansOfPeaches(batches);
int noOf15OuncesPeachBoxes = noOf15OuncesCansOfPeaches(batches);
shoppingList(batches, noOfYellowCakes, noOf29OuncesPeachBoxes, noOf15OuncesPeachBoxes, noOfBoxesOfButter);
quantitiesUsed(batches, noOfYellowCakes, noOf29OuncesPeachBoxes, noOf15OuncesPeachBoxes, noOfBoxesOfButter);
cobberLeftOver(batches, servings, servingsPerBatch);
}
private static void shoppingList(int batch, int noOfCakes, int noOf29Ounces, int noOf15Ounces, int noOfButter) {
System.out.println(String.format("GROCERY LIST FOR <%d> BATCHES OF COBBLER\n", batch));
System.out.println(String.format("%d\t29 ounce cans peaches", noOf29Ounces));
System.out.println(String.format("%d\t15 ounce cans peaches", noOf15Ounces));
System.out.println(String.format("%d\tYellow cake mixes", noOfCakes));
System.out.println(String.format("%d\tpounds of butter\n", noOfButter));
}
private static void quantitiesUsed(int batch, int noOfCakes, int noOf29Ounces, int noOf15Ounces, int noOfButter) {
System.out.println("QUANTITIES USED\n");
System.out.println(String.format("%d\tounces of peaches used\t\t%d\tleft over", batch * 22,
noOf29Ounces * 29 + noOf15Ounces * 15 - batch * 22));
System.out.println(String.format("%.1f\tcups of butter used\t\t%.1f\tleft over\n", batch * 3.0 / 8,
noOfButter * 2 - batch * 3.0 / 8));
}
private static void cobberLeftOver(int batch, int servings, int perServing) {
System.out.println("LEFTOVER COBBLER\n");
System.out.println(String.format("There should be <%d> servings of cobbler left over, but don't count on it!",
(batch * perServing - servings) % perServing));
}
private static int noOf29OuncesCansOfPeaches(int batches) {
if (batches == 0)
return 0;
int ouncesOfPeachesNeededForCalculatedBatchSize = 22 * batches;
int noOf15OuncesCansOfPeaches = 0,
noOf29OuncesCansOfPeaches = (int) Math.ceil(ouncesOfPeachesNeededForCalculatedBatchSize / 29.0);
int bestCase = noOf29OuncesCansOfPeaches,
wastage = noOf29OuncesCansOfPeaches * 29 - ouncesOfPeachesNeededForCalculatedBatchSize;
int available;
while (--noOf29OuncesCansOfPeaches != 0) {
noOf15OuncesCansOfPeaches = (int) Math
.ceil((ouncesOfPeachesNeededForCalculatedBatchSize - noOf29OuncesCansOfPeaches * 29) / 15.0);
available = noOf15OuncesCansOfPeaches * 15 + noOf29OuncesCansOfPeaches * 29;
if (available - ouncesOfPeachesNeededForCalculatedBatchSize < wastage) {
wastage = available - ouncesOfPeachesNeededForCalculatedBatchSize;
bestCase = noOf29OuncesCansOfPeaches;
}
}
noOf15OuncesCansOfPeaches = (int) Math.ceil(ouncesOfPeachesNeededForCalculatedBatchSize / 15.0);
if (noOf15OuncesCansOfPeaches * 15 - ouncesOfPeachesNeededForCalculatedBatchSize < wastage)
return 0;
else
return bestCase;
}
private static int noOf15OuncesCansOfPeaches(int batches) {
if (batches == 0)
return 0;
int ouncesOfPeachesNeededForCalculatedBatchSize = 22 * batches;
int noOf29OuncesCansOfPeaches = 0,
noOf15OuncesCansOfPeaches = (int) Math.ceil(ouncesOfPeachesNeededForCalculatedBatchSize / 15.0);
int bestCase = noOf15OuncesCansOfPeaches,
wastage = noOf15OuncesCansOfPeaches * 15 - ouncesOfPeachesNeededForCalculatedBatchSize;
int available;
while (--noOf15OuncesCansOfPeaches != 0) {
noOf29OuncesCansOfPeaches = (int) Math
.ceil((ouncesOfPeachesNeededForCalculatedBatchSize - noOf15OuncesCansOfPeaches * 15) / 29.0);
available = noOf15OuncesCansOfPeaches * 15 + noOf29OuncesCansOfPeaches * 29;
if (available - ouncesOfPeachesNeededForCalculatedBatchSize < wastage) {
wastage = available - ouncesOfPeachesNeededForCalculatedBatchSize;
bestCase = noOf15OuncesCansOfPeaches;
}
}
noOf29OuncesCansOfPeaches = (int) Math.ceil(ouncesOfPeachesNeededForCalculatedBatchSize / 29.0);
if (noOf29OuncesCansOfPeaches * 29 - ouncesOfPeachesNeededForCalculatedBatchSize < wastage)
return 0;
else
return bestCase;
}
private static int boxesOfButter(int batches) {
if (batches == 0)
return 0;
double amountOfButterNeededForCalculatedBatchSize = 0.1875 * batches; // 2 cups = 1 pound, therefore 3/8 cups =
// // 0.1875 pounds
return (int) Math.ceil(amountOfButterNeededForCalculatedBatchSize);
}
}
Step by step
Solved in 4 steps with 1 images

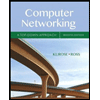
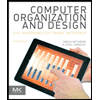
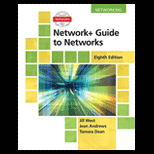
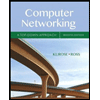
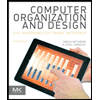
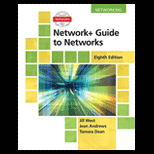
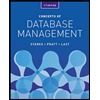
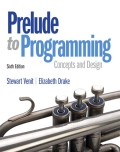
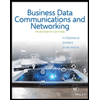