Write a function print_numbered_items (items) that takes a list of items and prints them one per line, each preceded by its index in the list, a colon and a space, as shown in the example. For this question you are required to use the len and range functions. You must use a for loop. Hint: This is the pattern explained in the info panel 'Iterating Over Lists Revisited'.
Write a function print_numbered_items (items) that takes a list of items and prints them one per line, each preceded by its index in the list, a colon and a space, as shown in the example. For this question you are required to use the len and range functions. You must use a for loop. Hint: This is the pattern explained in the info panel 'Iterating Over Lists Revisited'.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Loops summary: for vs. while
We have now seen that Python has two different loop constructs: the for loop and the while loop.
The while loop is more powerful than the for loop, since it can be used in situations where the number of iterations isn't known in advance. For
example, the following while loop answers the question: "If a population of bacteria increases in size by 21% every minute, how long does it take for
the population size to double?"
minutes = 0
population = 1000
growth_rate = 0.21
while population < 2000:
population *= (1 + growth_rate)
minutes += 1
print (f"{minutes} minutes required.")
print (f"Population = {int(population)}")
This would not be well suited to a for loop - we are trying to find out how many times the loop will run, and a for loop expects to know how many
times it will run before it starts.
In contrast, the for loop is a special case of the more general while loop, meaning that any program written with for loops can be rewritten to use
while loops. However, for loops are preferable when you are iterating over collections of data like lists. They are more compact, more readable, and
less error-prone than while loops.
To illustrate this point, the following questions ask you to write the same function in three different ways. These questions will force you to use - or
not use - specific functions and constructs, so make sure you precheck your answers to avoid penalties!
Write a function print_numbered_items (items) that takes a list of items and prints them one per line, each preceded by its index in the list, a colon
and a space, as shown in the example.
For this question you are required to use the len and range functions. You must use a for loop.
Hint: This is the pattern explained in the info panel 'Iterating Over Lists Revisited'.
For example:
Test
Result
names = ["Bob", "Tanya", "Wiremu", "ChinMay"] 0: Bob
print_numbered_items (names)
1: Tanya
2: Wiremu
3: ChinMay](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe391c883-e9e1-47fd-977c-8281e3a3314e%2F25019736-7733-466f-9469-8deca96fb2a4%2Fioachhu_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Loops summary: for vs. while
We have now seen that Python has two different loop constructs: the for loop and the while loop.
The while loop is more powerful than the for loop, since it can be used in situations where the number of iterations isn't known in advance. For
example, the following while loop answers the question: "If a population of bacteria increases in size by 21% every minute, how long does it take for
the population size to double?"
minutes = 0
population = 1000
growth_rate = 0.21
while population < 2000:
population *= (1 + growth_rate)
minutes += 1
print (f"{minutes} minutes required.")
print (f"Population = {int(population)}")
This would not be well suited to a for loop - we are trying to find out how many times the loop will run, and a for loop expects to know how many
times it will run before it starts.
In contrast, the for loop is a special case of the more general while loop, meaning that any program written with for loops can be rewritten to use
while loops. However, for loops are preferable when you are iterating over collections of data like lists. They are more compact, more readable, and
less error-prone than while loops.
To illustrate this point, the following questions ask you to write the same function in three different ways. These questions will force you to use - or
not use - specific functions and constructs, so make sure you precheck your answers to avoid penalties!
Write a function print_numbered_items (items) that takes a list of items and prints them one per line, each preceded by its index in the list, a colon
and a space, as shown in the example.
For this question you are required to use the len and range functions. You must use a for loop.
Hint: This is the pattern explained in the info panel 'Iterating Over Lists Revisited'.
For example:
Test
Result
names = ["Bob", "Tanya", "Wiremu", "ChinMay"] 0: Bob
print_numbered_items (names)
1: Tanya
2: Wiremu
3: ChinMay
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
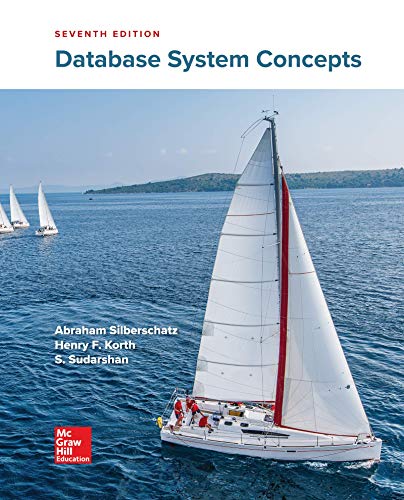
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
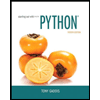
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
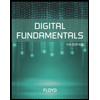
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
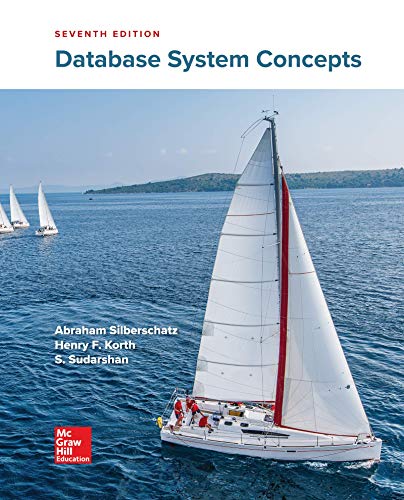
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
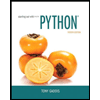
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
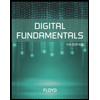
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
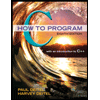
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
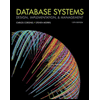
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
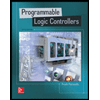
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education