write a code in C++ You work for a streaming analytics company and have been tasked with writing a program that analyzes views and likes on a series of YouTube videos. Code a modular program that uses parallel arrays to store views, likes, and like percentages for a series of videos. The program must do the following: The program must first ask the user for the number of videos that will be selected from YouTube. This must be done using a function that is called. For each video, the program must use random numbers to generate the number of views, likes, and the like percentage and store each value in a series of parallel arrays. These arrays must NOT be declared globally. These arrays also must be populated using a loop that calls a series of functions to generate the views, likes, and like percentage. The following arrays must be declared: views[] - an array of type long to hold the randomly generated views each YouTube video gets likes[] - an array of type long to hold the randomly generated likes each YouTube video gets (based on the number of views) likePercentages[] - an array of type double to hold the calculated percentages of likes of each video (ratio of likes to views) Once all of the arrays are populated, the program must display the stats for all of the videos. This includes displaying the number of views, likes, and the like percentage for each video. This must be done by calling a function that accepts each of the parallel arrays as well as the number of videos entered in step 1. Once the stats for the videos are displayed, the program must calculate and display the following: The video with the most views by calling a function that accepts an array that contains the array of views and the number of videos entered in step 1. The video with the least views by calling a function that accepts an array that contains the array of views and the number of videos entered in step 1. The video with the most likes by calling a function that accepts an array that contains the array of likes and the number of videos entered in step 1. The video with the least likes by calling a function that accepts an array that contains the array of likes and the number of videos entered in step 1. getNumVideos() - function must allow the user to specify the number of YouTube videos to be selected. Validate the user input so that the user must enter between 1 and 9 videos. If the user enters an invalid number of videos, they must be given an unlimited amount of chances to enter a valid number of YouTube videos. The function must return the validated number of YouTube videos. generateViews() - function will generate a random number of views for each video. Many videos on YouTube go viral, with very high view counts, so generate and return an amount of views up to 100,000,000,000. Note: use the long data type to return the number of views and store in the appropriate array. generateLikes() - Likes are generated based on the number of views generated on a YouTube video. Function must accept the views generated from the YouTube video and generate and return an amount of likes based on the the number of views the video has generated. Note: use the long data type to return the number of likes and store in the appropriate array. calculateLikePct() - Function must accept the views and the likes generated from the YouTube video and calculate the like percentage for each video using the following formula: like percentage = likes / views. Calculate and return the like percentage and store in the appropriate array Note: views and likes should be long data type, you will need to use type casting to convert them to a double to ensure proper calculation. displayStats() - Function must accept the parallel arrays that contain the views, likes, and percentages of likes as well as the number of videos and display the video number along with stats for each video formatted with proper spacing on separate lines to matc
write a code in C++ You work for a streaming analytics company and have been tasked with writing a program that analyzes views and likes on a series of YouTube videos. Code a modular program that uses parallel arrays to store views, likes, and like percentages for a series of videos. The program must do the following: The program must first ask the user for the number of videos that will be selected from YouTube. This must be done using a function that is called. For each video, the program must use random numbers to generate the number of views, likes, and the like percentage and store each value in a series of parallel arrays. These arrays must NOT be declared globally. These arrays also must be populated using a loop that calls a series of functions to generate the views, likes, and like percentage. The following arrays must be declared: views[] - an array of type long to hold the randomly generated views each YouTube video gets likes[] - an array of type long to hold the randomly generated likes each YouTube video gets (based on the number of views) likePercentages[] - an array of type double to hold the calculated percentages of likes of each video (ratio of likes to views) Once all of the arrays are populated, the program must display the stats for all of the videos. This includes displaying the number of views, likes, and the like percentage for each video. This must be done by calling a function that accepts each of the parallel arrays as well as the number of videos entered in step 1. Once the stats for the videos are displayed, the program must calculate and display the following: The video with the most views by calling a function that accepts an array that contains the array of views and the number of videos entered in step 1. The video with the least views by calling a function that accepts an array that contains the array of views and the number of videos entered in step 1. The video with the most likes by calling a function that accepts an array that contains the array of likes and the number of videos entered in step 1. The video with the least likes by calling a function that accepts an array that contains the array of likes and the number of videos entered in step 1. getNumVideos() - function must allow the user to specify the number of YouTube videos to be selected. Validate the user input so that the user must enter between 1 and 9 videos. If the user enters an invalid number of videos, they must be given an unlimited amount of chances to enter a valid number of YouTube videos. The function must return the validated number of YouTube videos. generateViews() - function will generate a random number of views for each video. Many videos on YouTube go viral, with very high view counts, so generate and return an amount of views up to 100,000,000,000. Note: use the long data type to return the number of views and store in the appropriate array. generateLikes() - Likes are generated based on the number of views generated on a YouTube video. Function must accept the views generated from the YouTube video and generate and return an amount of likes based on the the number of views the video has generated. Note: use the long data type to return the number of likes and store in the appropriate array. calculateLikePct() - Function must accept the views and the likes generated from the YouTube video and calculate the like percentage for each video using the following formula: like percentage = likes / views. Calculate and return the like percentage and store in the appropriate array Note: views and likes should be long data type, you will need to use type casting to convert them to a double to ensure proper calculation. displayStats() - Function must accept the parallel arrays that contain the views, likes, and percentages of likes as well as the number of videos and display the video number along with stats for each video formatted with proper spacing on separate lines to matc
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter13: Web Site Applications
Section: Chapter Questions
Problem 3E
Related questions
Question
write a code in C++
You work for a streaming analytics company and have been tasked with writing a
Code a modular program that uses parallel arrays to store views, likes, and like percentages for a series of videos.
The program must do the following:
- The program must first ask the user for the number of videos that will be selected from YouTube. This must be done using a function that is called.
- For each video, the program must use random numbers to generate the number of views, likes, and the like percentage and store each value in a series of parallel arrays. These arrays must NOT be declared globally. These arrays also must be populated using a loop that calls a series of functions to generate the views, likes, and like percentage.
- The following arrays must be declared:
- views[] - an array of type long to hold the randomly generated views each YouTube video gets
- likes[] - an array of type long to hold the randomly generated likes each YouTube video gets (based on the number of views)
- likePercentages[] - an array of type double to hold the calculated percentages of likes of each video (ratio of likes to views)
- The following arrays must be declared:
- Once all of the arrays are populated, the program must display the stats for all of the videos. This includes displaying the number of views, likes, and the like percentage for each video. This must be done by calling a function that accepts each of the parallel arrays as well as the number of videos entered in step 1.
- Once the stats for the videos are displayed, the program must calculate and display the following:
- The video with the most views by calling a function that accepts an array that contains the array of views and the number of videos entered in step 1.
- The video with the least views by calling a function that accepts an array that contains the array of views and the number of videos entered in step 1.
- The video with the most likes by calling a function that accepts an array that contains the array of likes and the number of videos entered in step 1.
- The video with the least likes by calling a function that accepts an array that contains the array of likes and the number of videos entered in step 1.
- getNumVideos() - function must allow the user to specify the number of YouTube videos to be selected. Validate the user input so that the user must enter between 1 and 9 videos. If the user enters an invalid number of videos, they must be given an unlimited amount of chances to enter a valid number of YouTube videos. The function must return the validated number of YouTube videos.
- generateViews() - function will generate a random number of views for each video. Many videos on YouTube go viral, with very high view counts, so generate and return an amount of views up to 100,000,000,000. Note: use the long data type to return the number of views and store in the appropriate array.
- generateLikes() - Likes are generated based on the number of views generated on a YouTube video. Function must accept the views generated from the YouTube video and generate and return an amount of likes based on the the number of views the video has generated. Note: use the long data type to return the number of likes and store in the appropriate array.
- calculateLikePct() - Function must accept the views and the likes generated from the YouTube video and calculate the like percentage for each video using the following formula: like percentage = likes / views. Calculate and return the like percentage and store in the appropriate array Note: views and likes should be long data type, you will need to use type casting to convert them to a double to ensure proper calculation.
- displayStats() - Function must accept the parallel arrays that contain the views, likes, and percentages of likes as well as the number of videos and display the video number along with stats for each video formatted with proper spacing on separate lines to match the sample output. Format the percentages of the likes to show numbers with 2 decimal places.
- maxViews() - Function must accept the array that contains the number of views as well as the number of videos entered and display the video that has the most views.
- minViews() - Function must accept the array that contains the number of views as well as the number of videos entered and display the video that has the least views.
- maxLikes() - Function must accept the array that contains the number of likes as well as the number of videos entered and display the video that has the most likes.
- minLikes() - Function must accept the array that contains the number of likes as well as the number of videos entered and display the video that has the least likes.
Other Notes:
Program Output and Prompts MUST MATCH the formatting of the sample runs EXACTLY.
output below

Transcribed Image Text:Enter the amount of videos that will be selected from YouTube (min: 1 - max: 9): −1
ERROR: ENTER BETWEEN 1 AND 9 VIDEOS
Enter the amount of videos that will be selected from YouTube: 99
ERROR: ENTER BETWEEN 1 AND 9 VIDEOS
Enter the amount of videos that will be selected from YouTube: 5
Video #
Video #1
Video #2
Video #3
Video #4
Video #5
Most Viewed Video
Video #3
Least Viewed Video
Video #4
Most Liked Video
Video #3
Least Liked Video
Video #5
Views
25413
17368
29195
7662
25636
29195 views
7662 views
26785 likes
1339 likes
Likes
1474
11340
26785
3374
1339
Like %
5.80
65.29
91.75
44.04
5.22

Transcribed Image Text:Enter the amount of videos that will be selected from YouTube (min: 1 - max: 9): 3
Video #
Video #1
Video #2
Video #3
Most Viewed Video
Video #3
Least Viewed Video
Video #2
Most Liked Video
Video #3
Least Liked Video
Video #2
Views
19189
14209
30416
30416 views
14209 views
13542 likes
6464 likes
Likes
8591
6464
13542
Like %
44.77
45.49
44.52
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
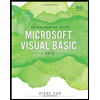
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
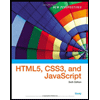
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
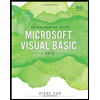
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
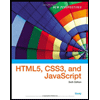
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage