Write a class named Patient that has attributes for the following data: First name, middle name, and last name Address, city, state, and ZIP code Phone number Name and phone number of emergency contact The Patient class’s _ _init_ _ method should accept an argument for each attribute. The Patient class should also have accessor and mutator methods for each attribute. In other words, write methods so you can set the attribute values or get their values. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: Name of the procedure Date of the procedure Name of the practitioner who performed the procedure Charges for the procedure The Procedure class’s _ _init_ _ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute. Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three instances of the Procedure class, initialized with the following data: Procedure #1: Procedure name: Physical Exam Date: Today’s date Practitioner: Dr. Irvine Charge: $250.00 Procedure #2: Procedure name: X-ray Date: Today’s date Practitioner: Dr. Jamison Charge: $500.00 Procedure #3: Procedure name: Blood test Date: Today’s date Practitioner: Dr. Smith Charge: $200.00 The program should display the patient’s information, information about all three of the procedures, and the total charges of the three procedures.
Write a class named Patient that has attributes for the following data:
- First name, middle name, and last name
- Address, city, state, and ZIP code
- Phone number
- Name and phone number of emergency contact
The Patient class’s _ _init_ _ method should accept an argument for each attribute. The Patient class should also have accessor and mutator methods for each attribute. In other words, write methods so you can set the attribute values or get their values.
Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data:
- Name of the procedure
- Date of the procedure
- Name of the practitioner who performed the procedure
- Charges for the procedure
The Procedure class’s _ _init_ _ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute. Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three instances of the Procedure class, initialized with the following data:
Procedure #1:
Procedure name: Physical Exam
Date: Today’s date
Practitioner: Dr. Irvine
Charge: $250.00
Procedure #2:
Procedure name: X-ray
Date: Today’s date
Practitioner: Dr. Jamison
Charge: $500.00
Procedure #3:
Procedure name: Blood test
Date: Today’s date
Practitioner: Dr. Smith
Charge: $200.00
The program should display the patient’s information, information about all three of the procedures, and the total charges of the three procedures.

class Patient:
first_name = ""
middle_name = ""
last_name = ""
address = ""
city = ""
state = ""
zip = ""
phone_number = ""
emergency_name = ""
emergency_contact = ""
def __init__(self, f, m, l, a, c, s, z, p, e_n, e_c):
self.first_name = f
self.middle_name = m
self.last_name = l
self.address = a
self.city = c
self.state = s
self.zip = z
self.phone_number = p
self.emergency_name = e_n
self.emergency_contact = e_c
def setfirstname(self, f):
self.first_name = f
def setmiddlename(self, m):
self.middle_name = m
def setlastname(self, l):
self.last_name = l
def setaddress(self, a):
self.address= a
def setcity(self, c):
self.city = c
def setstate(self, s):
self.state = s
def setzip(self, z):
self.zip = z
def setphonenumber(self, p):
self.phone_number = p
def setemergencyname(self, e_n):
self.emergency_name = e_n
def setemergencycontact(self, e_c):
self.emergency_contact = e_c
def getfirstname(self):
return self.first_name
def getmiddlename(self):
return self.middle_name
def getlastname(self):
return self.last_name
def getaddress(self):
return self.address
def getcity(self):
return self.city
def getstate(self):
return self.state
def getzip(self):
return self.zip
def getphonenumber(self):
return self.phone_number
def getemergencyname(self):
return self.emergency_name
def getemergencycontact(self):
return self.emergency_contact
class Procedure:
name = ""
date = ""
practitioner = ""
charges = 0
def __init__(self, n, d, p ,c):
self.name = n
self.date = d
self.practitioner = p
self.charges = c
def setname(self, n):
self.name = n
def setdate(self, d):
self.date = d
def setpractioner(self, p):
self.practitioner = p
def setcharges(self, c):
self.charges = c
def getname(self):
return self.name
def getdate(self):
return self.date
def getpractioner(self):
return self.practitioner
def getcharges(self):
return self.charges
patient1 = Patient("X", "Y", "Z", "ABC", "M","N", "123456", "09876543210", "ABC", "9876501234")
proc1 = Procedure("Physical Exam", "22-02-2021", "Dr. Irvine", 250.00)
proc2 = Procedure("X-ray","22-02-2021", "Dr.Jamison", 500.00)
proc3 = Procedure("Blood Test", "22-02-2021","Dr. Smith", 200.00)
print("Patient's Information: ")
print("First Name:", patient1.getfirstname())
print("Last Name:", patient1.getlastname())
print("Middle Name:", patient1.getmiddlename())
print("Address:", patient1.getaddress())
print("City:", patient1.getcity())
print("State:", patient1.getstate())
print("Zip:", patient1.getzip())
print("Phone Number:", patient1.getphonenumber())
print("Emergency contact name: ", patient1.getemergencyname())
print("Emergency contact number: ", patient1.getemergencycontact())
print("Procedure 1 details: ")
print("Procedure 1 name:", proc1.getname())
print("Procedure 1 date:", proc1.getdate())
print("Procedure 1 practitioner:", proc1.getpractioner())
print("Procedure 1 charges: $", proc1.getcharges())
print("Procedure 2 details: ")
print("Procedure 2 name:", proc2.getname())
print("Procedure 2 date:", proc2.getdate())
print("Procedure 2 practitioner:", proc2.getpractioner())
print("Procedure 2 charges: $", proc2.getcharges())
print("Procedure 3 details: ")
print("Procedure 3 name:", proc3.getname())
print("Procedure 3 date:", proc3.getdate())
print("Procedure 3 practitioner:", proc3.getpractioner())
print("Procedure 3 charges: $", proc3.getcharges())
print("Total charges: $", proc1.getcharges()+proc2.getcharges()+proc3.getcharges())
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

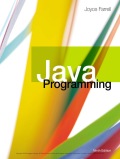
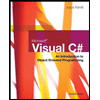
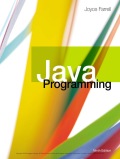
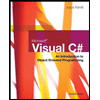