use commands move and mvprintw in c language to code this binary clock that sleeps for one second and changes display. time in the format 'h:m:s:" will be provided by user input. i have a clock.sh that displays the time in "h:m:s" format every second. This is the code in clock.sh. while true; do current_time=$(date +"%T") # Get the current time in HH:MM:SS format echo "$current_time" sleep 1 done Now, i need a display that looks like the below picture all this code will be put in a GitLab file named display-nopi.c. Here is my current code for this file. #include #include "display-nopi.h" static int is_display_open = 0; int open_display(void) { if (!is_display_open) { initscr(); start_color(); init_pair(1, COLOR_RED, COLOR_BLACK); init_pair(2, COLOR_GREEN, COLOR_BLACK); init_pair(3, COLOR_BLUE, COLOR_BLACK); is_display_open = 1; return 1; } return 0; } void display_time(int hours, int minutes, int seconds) { if (is_display_open) { clear(); display_hours(hours); display_colons(); display_minutes(minutes); display_colons(); display_seconds(seconds); refresh(); } } void display_colons(void) { attron(COLOR_PAIR(1)); mvaddch(3, 16, ':'); mvaddch(3, 20, ':'); attroff(COLOR_PAIR(1)); } void display_hours(int hours) { mvprintw(3, 14, "%02d", hours); } void display_minutes(int minutes) { attron(COLOR_PAIR(2)); mvprintw(3, 17, "%02d", minutes); attroff(COLOR_PAIR(2)); } void display_seconds(int seconds) { attron(COLOR_PAIR(3)); mvprintw(3, 21, "%02d", seconds); attroff(COLOR_PAIR(3)); } void close_display(void) { if (is_display_open) { endwin(); is_display_open = 0; } }
use commands move and mvprintw in c language to code this binary clock that sleeps for one second and changes display. time in the format 'h:m:s:" will be provided by user input. i have a clock.sh that displays the time in "h:m:s" format every second. This is the code in clock.sh.
while true; do
current_time=$(date +"%T") # Get the current time in HH:MM:SS format
echo "$current_time"
sleep 1
done
Now, i need a display that looks like the below picture
all this code will be put in a GitLab file named display-nopi.c. Here is my current code for this file.
#include <ncurses.h>
#include "display-nopi.h"
static int is_display_open = 0;
int open_display(void) {
if (!is_display_open) {
initscr();
start_color();
init_pair(1, COLOR_RED, COLOR_BLACK);
init_pair(2, COLOR_GREEN, COLOR_BLACK);
init_pair(3, COLOR_BLUE, COLOR_BLACK);
is_display_open = 1;
return 1;
}
return 0;
}
void display_time(int hours, int minutes, int seconds) {
if (is_display_open) {
clear();
display_hours(hours);
display_colons();
display_minutes(minutes);
display_colons();
display_seconds(seconds);
refresh();
}
}
void display_colons(void) {
attron(COLOR_PAIR(1));
mvaddch(3, 16, ':');
mvaddch(3, 20, ':');
attroff(COLOR_PAIR(1));
}
void display_hours(int hours) {
mvprintw(3, 14, "%02d", hours);
}
void display_minutes(int minutes) {
attron(COLOR_PAIR(2));
mvprintw(3, 17, "%02d", minutes);
attroff(COLOR_PAIR(2));
}
void display_seconds(int seconds) {
attron(COLOR_PAIR(3));
mvprintw(3, 21, "%02d", seconds);
attroff(COLOR_PAIR(3));
}
void close_display(void) {
if (is_display_open) {
endwin();
is_display_open = 0;
}
}


Step by step
Solved in 4 steps

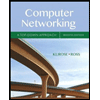
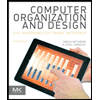
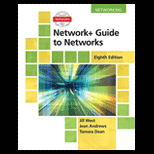
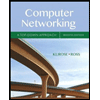
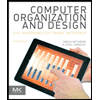
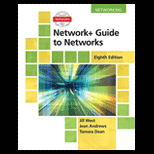
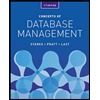
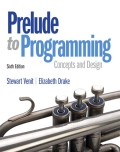
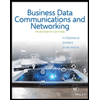