TOPIC: Inheritance In Python, modify the given code based on the given specifications below. Create another class, “Food.” For the Food class, the specifications are: The object will have the following attributes on initialization: weight (weight of the food in kg) energy_add (amount of energy it will add once consumed) happiness_add (amount of happiness it will add once consumed) Modify the Child and the Friends classes such that: Instead of accepting a string (as food) in the eat method, the method should accept a Foodobject Once a Child/Friendsobject eats a Food object: The added weight should be the Food object’s weight The added energy should be the Food object’s energy_add The added happiness should be the Food object’s happiness_add The given code (can also be found on the picture): class Child: def __init__(self, child_number: int, name: str, weight: float): self.child_number = child_number self.name = name self.weight = weight def eat(self, food: str): self.weight = self.weight + 0.50 def exercise(self, exercise: str): self.weight = self.weight - 0.50 def weight_in_grams(self): return self.weight * 1000 class Friends(Child): energy = 50 happiness = 50 def eat(self, food: str): self.energy = self.energy + 10 self.happiness = self.happiness + 10 return Child.eat(self, food) and self.energy and self.happiness def exercise(self, food: str): self.energy = self.energy - 10 self.happiness = self.happiness + 10 return Child.exercise(self, food) and self.energy and self.happiness
TOPIC: Inheritance In Python, modify the given code based on the given specifications below. Create another class, “Food.” For the Food class, the specifications are: The object will have the following attributes on initialization: weight (weight of the food in kg) energy_add (amount of energy it will add once consumed) happiness_add (amount of happiness it will add once consumed) Modify the Child and the Friends classes such that: Instead of accepting a string (as food) in the eat method, the method should accept a Foodobject Once a Child/Friendsobject eats a Food object: The added weight should be the Food object’s weight The added energy should be the Food object’s energy_add The added happiness should be the Food object’s happiness_add The given code (can also be found on the picture): class Child: def __init__(self, child_number: int, name: str, weight: float): self.child_number = child_number self.name = name self.weight = weight def eat(self, food: str): self.weight = self.weight + 0.50 def exercise(self, exercise: str): self.weight = self.weight - 0.50 def weight_in_grams(self): return self.weight * 1000 class Friends(Child): energy = 50 happiness = 50 def eat(self, food: str): self.energy = self.energy + 10 self.happiness = self.happiness + 10 return Child.eat(self, food) and self.energy and self.happiness def exercise(self, food: str): self.energy = self.energy - 10 self.happiness = self.happiness + 10 return Child.exercise(self, food) and self.energy and self.happiness
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
TOPIC: Inheritance
In Python, modify the given code based on the given specifications below. Create another class, “Food.”
For the Food class, the specifications are:
- The object will have the following attributes on initialization:
- weight (weight of the food in kg)
- energy_add (amount of energy it will add once consumed)
- happiness_add (amount of happiness it will add once consumed)
Modify the Child and the Friends classes such that:
- Instead of accepting a string (as food) in the eat method, the method should accept a Foodobject
- Once a Child/Friendsobject eats a Food object:
- The added weight should be the Food object’s weight
- The added energy should be the Food object’s energy_add
- The added happiness should be the Food object’s happiness_add
The given code (can also be found on the picture):
class Child:
def __init__(self, child_number: int, name: str, weight: float):
self.child_number = child_number
self.name = name
self.weight = weight
def eat(self, food: str):
self.weight = self.weight + 0.50
def exercise(self, exercise: str):
self.weight = self.weight - 0.50
def weight_in_grams(self):
return self.weight * 1000
class Friends(Child):
energy = 50
happiness = 50
def eat(self, food: str):
self.energy = self.energy + 10
self.happiness = self.happiness + 10
return Child.eat(self, food) and self.energy and self.happiness
def exercise(self, food: str):
self.energy = self.energy - 10
self.happiness = self.happiness + 10
return Child.exercise(self, food) and self.energy and self.happiness

Transcribed Image Text:class Child:
def _init_(self, child_number: int, name: str, weight: float):
self.child_number = child_number
%3D
self.name = name
self.weight = weight
def eat(self, food: str):
self.weight = self.weight + 0.50
def exercise(self, exercise: str):
self.weight = self.weight - 0.50
def weight_in_grams(self):
return self.weight * 1000
class Friends(Child):
energy = 50
happiness = 50
%3D
def eat(self, food: str):
self.energy = self.energy + 10
self.happiness = self.happiness + 10
return Child.eat(self, food) and self.energy and self.happiness
%3D
def exercise(self, food: str):
self.energy = self.energy - 10
self.happiness = self.happiness + 10
return Child.exercise(self, food) and self.energy and self.happiness
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
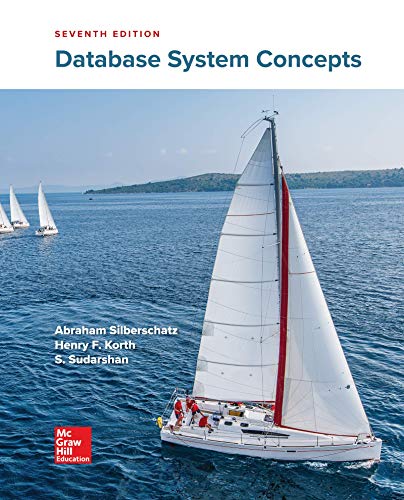
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
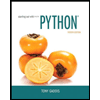
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
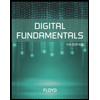
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
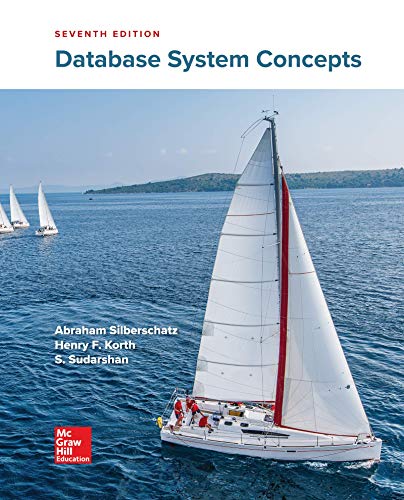
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
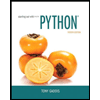
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
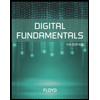
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
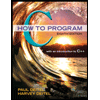
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
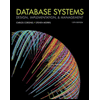
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
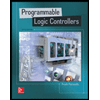
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education