### TODO - load the data from csv file ### use csv.reader to append rows of filename to data
IN PYTHON - please help with lines beginning with ###TODO
import csv
import random as rand
import sys
# define functions
def load_csv_data(filename):
"""
load the data from csv file
returns a tuple containing two lists:
col_names: first row of csv file (list of strings)
row_data: all other rows of csv file (list of list of strings)
if error is encountered reading filename, returns -1
"""
try:
data = []
### TODO - load the data from csv file
### use csv.reader to append rows of filename to data
except:
print("Error loading data.")
return -1
# if successful, return tuple of column names and row data
col_names = data[0]
row_data = data[1:]
return (col_names, row_data)
def display_menu():
### TODO - print the main menu
print("Main Menu")
print("1 - Get Clean Sample")
print("2 - Calculate Total Profit in Sample")
print("Q - Quit")
def get_valid_menu_option():
### TODO - get valid menu option from the user
### use prompt ">>> choose menu option: "
### if not valid selection, print message "invalid input" and get again
### repeat until valid and return that result
user_input = (">>> choose menu option: ")
if user_input != '1':
print("invalid input")
elif user_input != '2':
print("invalid input")
elif user_input != 'Q':
print("invalid input")
else:
print(">>> choose menu option: ")
return user_input
def get_clean_sample(data, n=20):
# get n rows randomly sampled w/o replacement from the data
sample = rand.sample(data, n)
### TODO - convert string types in all rows of sample to int / float type
### positions 0 and 8 should be integers
### positions 9 and 10 should be floats
### return the updated sample
def print_sample_summary(sample):
"""print summary data for a given sample, returns nothing"""
print(f"\nSample size {len(sample)} rows x {len(sample[0])} cols.")
print("First order number:\t", sample[0][0])
print("Last order number:\t", sample[-1][0])
tot = 0
for row in sample:
tot += row[8]
avg = tot / len(sample)
print("Average units per order:", avg)
# check if run as module or script
if __name__ == "__main__":
# set random seed
seed = input("*** Enter random seed (integer) or ENTER for none: ")
if seed:
rand.seed(int(seed))
print("\nRandom seed set to", seed)
else:
print("\nNo random seed set")
# load csv data file
# this file must be in the same directory as your .py file
file = "5000 Sales Records.csv"
data = load_csv_data(file)
if data == -1:
print("Ending.")
sys.exit()
else:
# unpack the data tuple into header and rows; print result
col_names, row_data = data
print(f"\nLoaded '{file}', {len(row_data)} rows x {len(row_data[0])} cols.")
### TODO - implement main menu loop per specification

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

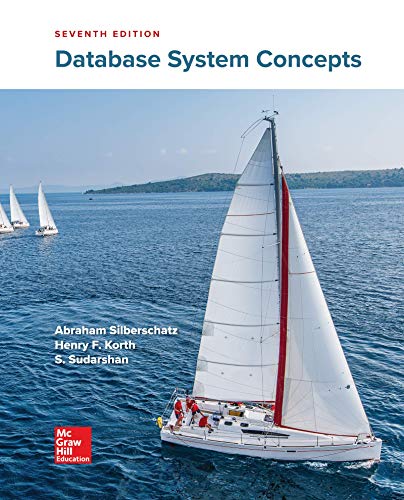
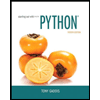
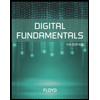
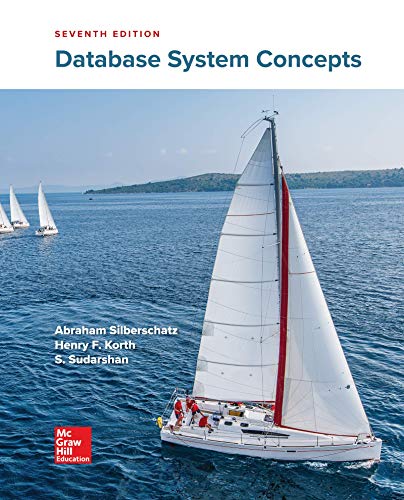
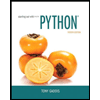
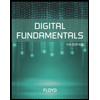
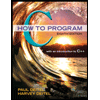
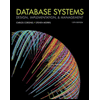
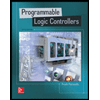