This program goes where no program has gone before, exploring new frontiers in C++. The program performs calculations concerning weight on various planets as well as travel time between planets. This will be a multi-file program consisting of Driver.cpp (main) and functions that will be placed in TravelFunctions.cpp and TravelFunctions.h files. You will use structures to hold Planet and Traveler objects. Use Array’s to store the Planet Objects. There will only be a single Traveler. In main, use Arrays to hold Planet Objects (names, distance from the sun, and the specific gravity on each planet). Create a global constant variable for the number of Planets. Place it in a location that both the TravelFunctions.cpp and Driver.cpp files can get access to it. const int NUM_PLANETS{8}; Your program should first declare variables, instantiate your Array of Planets. Display your course header with the program title and a brief introduction using a cout. Load the data into the Array of Planets using GetData. You will use ifstream in GetData to read from a .csv (comma separated value) file. The file name is “PlanetData.csv” and will need to be imported into the program. Start a do while loop as your Play loop. Ask for the user’s first and last name, Earth weight, the speed they want to travel and the planet they wish to visit. Store this in the Traveler object. You will need to provide a menu with the planet names and a way for the user to select the chosen destination. Using the data you have obtained from the user, determine the user’s weight on the destination planet and the travel time in hours. These equations might be useful: Weight on New Planet = Weight on Earth * Relative Surface Gravity of New Planet Travel Time (hours) = Travel Distance (miles) / Speed of Travel(mph) Store all data in the Traveler Object Use the data in this table to load the arrays and Planet Objects: Planet Distance from Sun (millions of miles) Surface Gravity as a Percent of Earth’s Gravity Mercury 36 0.27 Venus 67 0.86 Earth 93 1.00 Mars 141 0.37 Jupiter 483 2.64 Saturn 886 1.17 Uranus 1782 0.92 Neptune 2793 1.44 Display the traveler’s name, weight on Earth, the destination planet and weight on that planet. Also report travel time results: Break down the travel time into years, days and hours. You may use 24 hours in a day and 365 days in a year (ignore leap years). Display the number of years, days and hours required for the trip. Also, display the current date and the date the Traveler will arrive at their destination planet. Use a stringstream to capture the final report. Output the final report to the console and a file named “_.txt” Your program should have only one set of result variables. The code for the calculations and resultant output should be written in only one place in your program. Do not duplicate code and calculation for each planet. Make your program as efficient as possible without losing clarity. Ask the user if they would like to calculate another space trip. Remember to tell the user the choices for an answer. If they say yes, loop up to the top of the Play Loop. When the user is done, present a good-bye message. Set the precision, etc, so you are reporting floating point numbers with 2 decimal places. Use only the following functions and structures. Do not add to or take away from the following functions and structures. Do not add additional functions. Do not add additional structures. Remember to place all prototypes in the .h header file and these functions in the TravelFunctions.cpp file. Remember to place structure in .h (header) and create appropriate objects as necessary. struct Planet { String name{“”}; Int distFromSun{-1}; Float surfGrav {-1.0}; }; struct Traveler { String firstName{“”}; String lastName {“”}; Float weightEarth {-1.0}; Float weightPlanet {-1.0}; Planet destPlanet; Int travelTime[3]; //travel time array index 0 = years, 1 = days, 2 = hours }; Functions Bool GetData(Planet planetData[], string filename); Bool WriteData(string filename, Traveler & r_travelerData); Void GetUserData(Traveler * p_travelerData); Void DisplayPlanets(Planet planetData[]); Int GetDestinationPlanet(); Void CalcTravelTime(Planet destPlanet, Traveler * p_travelerData); Void Header(); String GetInputFilename();
CIS 1275 C++
Using
This program goes where no program has gone before, exploring new frontiers in C++. The program performs calculations concerning weight on various planets as well as travel time between planets.
This will be a multi-file program consisting of Driver.cpp (main) and functions that will be placed in TravelFunctions.cpp and TravelFunctions.h files.
You will use structures to hold Planet and Traveler objects. Use Array’s to store the Planet Objects. There will only be a single Traveler.
In main, use Arrays to hold Planet Objects (names, distance from the sun, and the specific gravity on each planet). Create a global constant variable for the number of Planets. Place it in a location that both the TravelFunctions.cpp and Driver.cpp files can get access to it.
const int NUM_PLANETS{8};
Your program should first declare variables, instantiate your Array of Planets.
Display your course header with the program title and a brief introduction using a cout. Load the data into the Array of Planets using GetData. You will use ifstream in GetData to read from a .csv (comma separated value) file. The file name is “PlanetData.csv” and will need to be imported into the program. Start a do while loop as your Play loop.
Ask for the user’s first and last name, Earth weight, the speed they want to travel and the planet they wish to visit. Store this in the Traveler object. You will need to provide a menu with the planet names and a way for the user to select the chosen destination.
Using the data you have obtained from the user, determine the user’s weight on the destination planet and the travel time in hours.
These equations might be useful:
Weight on New Planet = Weight on Earth * Relative Surface Gravity of New Planet
Travel Time (hours) = Travel Distance (miles) / Speed of Travel(mph)
Store all data in the Traveler Object
Use the data in this table to load the arrays and Planet Objects:
Planet |
Distance from Sun (millions of miles) |
Surface Gravity as a Percent of Earth’s Gravity |
Mercury |
36 |
0.27 |
Venus |
67 |
0.86 |
Earth |
93 |
1.00 |
Mars |
141 |
0.37 |
Jupiter |
483 |
2.64 |
Saturn |
886 |
1.17 |
Uranus |
1782 |
0.92 |
Neptune |
2793 |
1.44 |
Display the traveler’s name, weight on Earth, the destination planet and weight on that planet. Also report travel time results: Break down the travel time into years, days and hours. You may use 24 hours in a day and 365 days in a year (ignore leap years). Display the number of years, days and hours required for the trip. Also, display the current date and the date the Traveler will arrive at their destination planet.
Use a stringstream to capture the final report. Output the final report to the console and a file named “<Traveler Last Name>_<Destination planet>.txt”
Your program should have only one set of result variables. The code for the calculations and resultant output should be written in only one place in your program. Do not duplicate code and calculation for each planet. Make your program as efficient as possible without losing clarity.
Ask the user if they would like to calculate another space trip. Remember to tell the user the choices for an answer. If they say yes, loop up to the top of the Play Loop. When the user is done, present a good-bye message.
Set the precision, etc, so you are reporting floating point numbers with 2 decimal places.
Use only the following functions and structures. Do not add to or take away from the following functions and structures. Do not add additional functions. Do not add additional structures. Remember to place all prototypes in the .h header file and these functions in the TravelFunctions.cpp file. Remember to place structure in .h (header) and create appropriate objects as necessary.
struct Planet
{
String name{“”};
Int distFromSun{-1};
Float surfGrav {-1.0};
};
struct Traveler
{
String firstName{“”};
String lastName {“”};
Float weightEarth {-1.0};
Float weightPlanet {-1.0};
Planet destPlanet;
Int travelTime[3]; //travel time array index 0 = years, 1 = days, 2 = hours
};
Functions
Bool GetData(Planet planetData[], string filename);
Bool WriteData(string filename, Traveler & r_travelerData);
Void GetUserData(Traveler * p_travelerData);
Void DisplayPlanets(Planet planetData[]);
Int GetDestinationPlanet();
Void CalcTravelTime(Planet destPlanet, Traveler * p_travelerData);
Void Header();
String GetInputFilename();

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 12 images

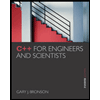
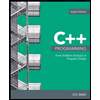
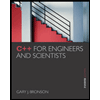
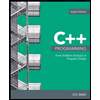