THIS NEEDS TO BE DONE IN C#!! The Tourtise and The Hair In this lab, we will be simulating the classic race of the tourtise and the hare. The race will take place on two paths of 70 tiles, which spans from left to right. The path will have two lanes, one for each animal. The path will be represented as an array of 70 characters, where each character will be the following: · A dash(i.e, “-“), which represents an empty tile. · An “h”, which represents the hare on the hare lane. · A “t”, which represents the tourtise on the tortise lane. All tiles will be “empty” (i.e. set to the dash) with the exception of the tiles that are occupied by the animals. As the animals move, the previous tile the animal was on will be set to empty, and the new tile will be changed to either “h” or “t”. Tile 70 will be the finishing tile, and the first animal to that tile will be declared the winner of the race. We will use randomization to determine how far each animal will move. Since this race will be hilly, there is a chance that both animals slip, meaning that they will be pushed backwards on the race. The following table describes how each animal moves: To generate the move made, the program will generate a random number from 1 to 10. The number generated will determine the move made. Take the torotise for example. If the number is 1 – 5(50% of the possible range), then the torotise will perform a “dash” move, 6 and 7 will perform a slip, and 8 – 10 will perform a walk. The hare’s movements will be determined in a similar fashion. Additionally, to see the moves in real time, the program will pause for 1 second between each move. To do so, use C#’s sleep function, which is typed as “Thread.Sleep(1000)”. Additionally, the console window can be cleared using “Console.Clear()” so that the previous positions are erased from the screen(giving the impression of the race being “animated”). To design your program, you are going to apply inheritance and polymorphic techniques. For the animals, you are going to have three classes: a RaceAnimal class which serves as a base class, and two additional classes, one for each animal, that serve as derived classes. The RaceAnimal class will be abstract and define attributes/methods for managing the track. The RaceAnimal class will have an abstract method called Move, which must be overridden in the derived classes and defined how that specific animal moves. Additionally, you will also define a Race class, which simulates the overall race. The main function will then call race.simulate() to start the race. RaceAnimal Attribute Descriptions: · track – stores the current track. The symbol is printed in the element for the current position of the animal. Every other element should have a dash(‘-‘). · symbol – stores the symbol used to represent the animal on the track. · position – stores the current position of the animal on the track. Is the index of the element in the track array. Must only be a number between 0 and 69. · name – stores the name of the animal. RaceAnimal Method Descriptions: · constructor – sets the track up by printing the dashes and animal symbol. The name and symbol of the animal should be passed in as parameters. The derived classes will pass in the name and symbol to the based class using the example listed in Appendix A. · ToString – Overrides the ToString method in C#. When called, should output the animals track in the Race. Will be used in the Race class for output. Returns a string built from the track array. · ChangePos – A utility method that takes in the number of steps to move and applies it to the track. Can move the animal backwards and forwards. This should check the bounds of the track to ensure the move is in bounds. · Move – An abstrat method that MUST ONLY be defined in the derived classes. When called, moves the animal along the track. Will be overridden for each of the animals. UML Tables for Hare and Tortoise Class class Hare: RaceAnimal class Tortoise: RaceAnimal URL Legend Plus(“+”) : public access Dash(“-“): private access Hashtag(“#”): protected access +constructor() overridden Move() +constructor() overridden Move() Hare and Tourtise Method Descriptions: · constructor – Calls the RaceAnimal method and passes the animal name and symbol to the base class constructor (see Appendix A for an example of how to do this.) Does nothing else…. · Move – Overrides the Move method in RaceAnimal class. Will update the position of the animal based on the charts provided above for each animal type. Calls ChangePos once the move type is determined to change the position of the animal. Note: These are two separate class definitions, which each inherit from RaceAnimal. Each class’s Move method will be coded based on how the type of animal moves.
THIS NEEDS TO BE DONE IN C#!!
The Tourtise and The Hair
In this lab, we will be simulating the classic race of the tourtise and the hare. The race will take place on two paths of 70 tiles, which spans from left to right. The path will have two lanes, one for each animal. The path will be represented as an array of 70 characters, where each character will be the following:
· A dash(i.e, “-“), which represents an empty tile.
· An “h”, which represents the hare on the hare lane.
· A “t”, which represents the tourtise on the tortise lane.
All tiles will be “empty” (i.e. set to the dash) with the exception of the tiles that are occupied by the animals. As the animals move, the previous tile the animal was on will be set to empty, and the new tile will be changed to either “h” or “t”. Tile 70 will be the finishing tile, and the first animal to that tile will be declared the winner of the race.
We will use randomization to determine how far each animal will move. Since this race will be hilly, there is a chance that both animals slip, meaning that they will be pushed backwards on the race. The following table describes how each animal moves:
To generate the move made, the program will generate a random number from 1 to 10. The number generated will determine the move made. Take the torotise for example. If the number is 1 – 5(50% of the possible range), then the torotise will perform a “dash” move, 6 and 7 will perform a slip, and 8 – 10 will perform a walk. The hare’s movements will be determined in a similar fashion. Additionally, to see the moves in real time, the program will pause for 1 second between each move. To do so, use C#’s sleep function, which is typed as “Thread.Sleep(1000)”. Additionally, the console window can be cleared using “Console.Clear()” so that the previous positions are erased from the screen(giving the impression of the race being “animated”).
To design your program, you are going to apply inheritance and polymorphic techniques. For the animals, you are going to have three classes: a RaceAnimal class which serves as a base class, and two additional classes, one for each animal, that serve as derived classes. The RaceAnimal class will be abstract and define attributes/methods for managing the track. The RaceAnimal class will have an abstract method called Move, which must be overridden in the derived classes and defined how that specific animal moves. Additionally, you will also define a Race class, which simulates the overall race. The main function will then call race.simulate() to start the race.
RaceAnimal Attribute Descriptions:
· track – stores the current track. The symbol is printed in the element for the current position of the animal. Every other element should have a dash(‘-‘).
· symbol – stores the symbol used to represent the animal on the track.
· position – stores the current position of the animal on the track. Is the index of the element in the track array. Must only be a number between 0 and 69.
· name – stores the name of the animal.
RaceAnimal Method Descriptions:
· constructor – sets the track up by printing the dashes and animal symbol. The name and symbol of the animal should be passed in as parameters. The derived classes will pass in the name and symbol to the based class using the example listed in Appendix A.
· ToString – Overrides the ToString method in C#. When called, should output the animals track in the Race. Will be used in the Race class for output. Returns a string built from the track array.
· ChangePos – A utility method that takes in the number of steps to move and applies it to the track. Can move the animal backwards and forwards. This should check the bounds of the track to ensure the move is in bounds.
· Move – An abstrat method that MUST ONLY be defined in the derived classes. When called, moves the animal along the track. Will be overridden for each of the animals.
UML Tables for Hare and Tortoise Class
class Hare: RaceAnimal class Tortoise: RaceAnimal URL Legend
<none> <none> Plus(“+”) : public access Dash(“-“): private access Hashtag(“#”): protected access
+constructor()
overridden Move() +constructor() overridden Move()
Hare and Tourtise Method Descriptions:
· constructor – Calls the RaceAnimal method and passes the animal name and symbol to the base class constructor (see Appendix A for an example of how to do this.) Does nothing else….
· Move – Overrides the Move method in RaceAnimal class. Will update the position of the animal based on the charts provided above for each animal type. Calls ChangePos once the move type is determined to change the position of the animal.
Note: These are two separate class definitions, which each inherit from RaceAnimal. Each class’s Move method will be coded based on how the type of animal moves.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

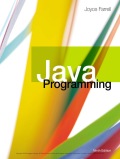
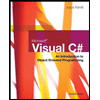
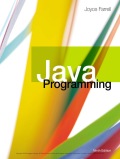
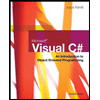