This is the question I am stuck on - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons. Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return saleAmount; } public int getInvoiceNumber() { return invoiceNumber; } public void display() { System.out.println("Invoice #" + invoiceNumber + " Amount of sale: $" + saleAmount + " Tax: $" + tax); } } -------------------------------------------------------------------------- import java.util.Scanner; public class SortPurchasesArray { public static void main(String[] args) { // Write code here } public static void sortBySaleAmount(Purchase[] array) { // Write code here } public static void sortByInvoice(Purchase[] array) { // Write code here } public static void display(Purchase[] p, String msg) { // Write code here } }
This is the question I am stuck on - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons. Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return saleAmount; } public int getInvoiceNumber() { return invoiceNumber; } public void display() { System.out.println("Invoice #" + invoiceNumber + " Amount of sale: $" + saleAmount + " Tax: $" + tax); } } -------------------------------------------------------------------------- import java.util.Scanner; public class SortPurchasesArray { public static void main(String[] args) { // Write code here } public static void sortBySaleAmount(Purchase[] array) { // Write code here } public static void sortByInvoice(Purchase[] array) { // Write code here } public static void display(Purchase[] p, String msg) { // Write code here } }
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 7PE
Related questions
Question
This is the question I am stuck on -
In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons.
Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order.
This is the code I have -
public class Purchase
{
private int invoiceNumber;
private double saleAmount;
private double tax;
private static final double RATE = 0.05;
public void setInvoiceNumber(int num)
{
invoiceNumber = num;
}
public void setSaleAmount(double amt)
{
saleAmount = amt;
tax = saleAmount * RATE;
}
public double getSaleAmount()
{
return saleAmount;
}
public int getInvoiceNumber()
{
return invoiceNumber;
}
public void display()
{
System.out.println("Invoice #" + invoiceNumber +
" Amount of sale: $" + saleAmount + " Tax: $" + tax);
}
}
--------------------------------------------------------------------------
import java.util.Scanner;
public class SortPurchasesArray {
public static void main(String[] args) {
// Write code here
}
public static void sortBySaleAmount(Purchase[] array) {
// Write code here
}
public static void sortByInvoice(Purchase[] array) {
// Write code here
}
public static void display(Purchase[] p, String msg) {
// Write code here
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
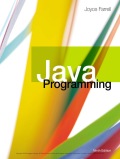
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
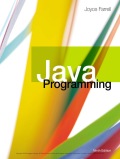
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage