* This is the code for the Word Jumble game from Chapter 3. Improve the Word Jumble game by adding a scoring system. Make the point valuefor a word based on its length. Deduct points if the player asks for a hint. */ // Word Jumble // The classic word jumble game where the player can ask for a hint #include #include #include #include using namespace std; int main() { enum fields {WORD, HINT, NUM_FIELDS}; const int NUM_WORDS = 5; const string WORDS[NUM_WORDS][NUM_FIELDS] = { {"wall", "Do you feel you're banging your head against something?"}, {"glasses", "These might help you see the answer."}, {"labored", "Going slowly, is it?"}, {"persistent", "Keep at it."}, {"jumble", "It's what the game is all about."} }; srand(static_cast(time(0))); int choice = (rand() % NUM_WORDS); string theWord = WORDS[choice][WORD]; //word to guess string theHint = WORDS[choice][HINT]; //hint for the word string jumble = theWord; //jumbled version of the world int length = jumble.size(); for (int i = 0; i < length; i++) { int index1 = (rand() % length); int index2 = (rand() % length); char temp = jumble[index1]; jumble[index1] = jumble[index2]; jumble[index2] = temp; } cout << "\t\t\tWelcome to Word Jumble!\n\n"; cout << "Unscramble the letters to make a word.\n"; cout << "Enter 'hint' for a hint\n"; cout << "Enter 'quit' to quit the game.\n\n"; cout << "The jumble is: " << jumble; string guess; cout << "\n\nYour guess: "; cin >> guess; while ((guess != theWord) && (guess != "quit")) { if (guess == "hint") { cout << theHint; } else { cout << "Sorry, that's not it."; } cout << "\n\nYour guess: "; cin >> guess; } if (guess == theWord) { cout << "\nThat's it! You guessed it!\n"; } cout << "\nThanks for playing.\n"; return 0; }
/*
This is the code for the Word Jumble game from Chapter 3.
Improve the Word Jumble game by adding a scoring system. Make the point valuefor a word based on its length. Deduct points if the player asks for a hint.
*/
// Word Jumble
// The classic word jumble game where the player can ask for a hint
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
int main()
{
enum fields {WORD, HINT, NUM_FIELDS};
const int NUM_WORDS = 5;
const string WORDS[NUM_WORDS][NUM_FIELDS] =
{
{"wall", "Do you feel you're banging your head against something?"},
{"glasses", "These might help you see the answer."},
{"labored", "Going slowly, is it?"},
{"persistent", "Keep at it."},
{"jumble", "It's what the game is all about."}
};
srand(static_cast<unsigned int>(time(0)));
int choice = (rand() % NUM_WORDS);
string theWord = WORDS[choice][WORD]; //word to guess
string theHint = WORDS[choice][HINT]; //hint for the word
string jumble = theWord; //jumbled version of the world
int length = jumble.size();
for (int i = 0; i < length; i++)
{
int index1 = (rand() % length);
int index2 = (rand() % length);
char temp = jumble[index1];
jumble[index1] = jumble[index2];
jumble[index2] = temp;
}
cout << "\t\t\tWelcome to Word Jumble!\n\n";
cout << "Unscramble the letters to make a word.\n";
cout << "Enter 'hint' for a hint\n";
cout << "Enter 'quit' to quit the game.\n\n";
cout << "The jumble is: " << jumble;
string guess;
cout << "\n\nYour guess: ";
cin >> guess;
while ((guess != theWord) && (guess != "quit"))
{
if (guess == "hint")
{
cout << theHint;
} else
{
cout << "Sorry, that's not it.";
}
cout << "\n\nYour guess: ";
cin >> guess;
}
if (guess == theWord)
{
cout << "\nThat's it! You guessed it!\n";
}
cout << "\nThanks for playing.\n";
return 0;
}

- Added an integer variable points to keep track of the player's score.
- Initialized points to the length of the word to be guessed.
- Added a boolean flag hintTaken to keep track of whether the player has taken a hint or not.
- Added points-- after each incorrect guess or hint taken to deduct a point from the player's score.
- Added an if statement to check if the player guessed the word without taking a hint, and added two bonus points to the player's score if this is the case.
- Displayed the player
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

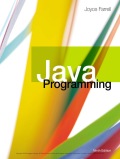
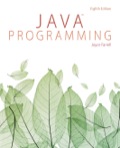
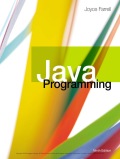
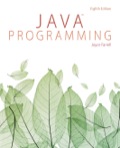