The question should be done in C++, please give explanation and running result. 1. Let’s write a function that compares 2 strings and returns true if they were identical, false otherwise. The signature of this function should be: bool word_diff(std::string word1, std::string word2); For example: std::string str1 = "Hello World"; std::string str2 = "hEllO World"; std::string str3 = "World"; std::string str4 = "Hello World"; bool result = word_diff(str1, str2); // False bool result = word_diff(str1, str3); // False bool result = word_diff(str1, str4); // True 2. Now let’s follow a classical approach to compare the content of 2 files. We should open each file, read their content word by word and compare them until a first mismatch occurs. Let’s implement a function called classical_file_diff that takes 2 arguments each of which is a file name and returns a boolean indicating whether the 2 files are identical or not. The signature of this function should be: bool classical_file_diff(std::string file1, std::string file2); This function must use the helper function from Question 1 that compares 2 words. Example: Create a folder within the same directory where your executable resides and name it “txt_folder”. In it create 2 files: file1.txt and file2.txt. In file1.txt copy/paste the following lines: My dear C++ class. I hope that you enjoy this assignment. file2.txt copy/paste the following lines: My dear C++ class. I hope that you like this assignment. The following code should yield false in the variable result: std::string file1 = "./txt_folder/file1.txt"; std::string file2 = "./txt_folder/file2.txt"; bool result = classical_file_diff(file1, file2); // False Please note that you may need to modify the slashes in the path name according to the expectations of your operating system.
The question should be done in C++, please give explanation and running result.
1. Let’s write a function that compares 2 strings and returns true if they were identical, false otherwise. The signature of this function should be:
bool word_diff(std::string word1, std::string word2);
For example:
std::string str1 = "Hello World";
std::string str2 = "hEllO World";
std::string str3 = "World";
std::string str4 = "Hello World";
bool result = word_diff(str1, str2); // False
bool result = word_diff(str1, str3); // False
bool result = word_diff(str1, str4); // True
2. Now let’s follow a classical approach to compare the content of 2 files. We should open each file, read their content word by word and compare them until a first mismatch occurs.
Let’s implement a function called classical_file_diff that takes 2 arguments each of which is a file name and returns a boolean indicating whether the 2 files are identical or not. The signature of this function should be:
bool classical_file_diff(std::string file1, std::string file2);
This function must use the helper function from Question 1 that compares 2 words.
Example: Create a folder within the same directory where your executable resides and name it “txt_folder”. In it create 2 files: file1.txt and file2.txt.
In file1.txt copy/paste the following lines:
My dear C++ class.
I hope that you enjoy this assignment.
file2.txt copy/paste the following lines:
My dear C++ class.
I hope that you like this assignment.
The following code should yield false in the variable result:
std::string file1 = "./txt_folder/file1.txt";
std::string file2 = "./txt_folder/file2.txt";
bool result = classical_file_diff(file1, file2); // False
Please note that you may need to modify the slashes in the path name according to the expectations of your

Step by step
Solved in 5 steps with 2 images

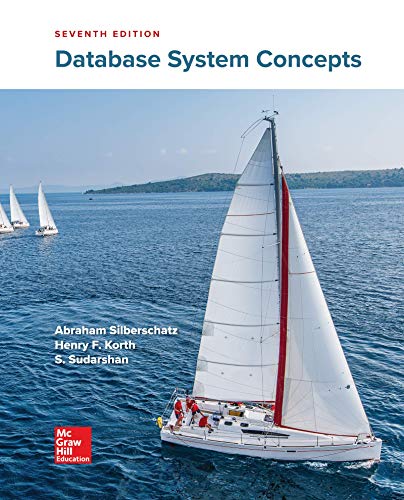
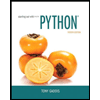
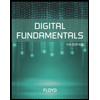
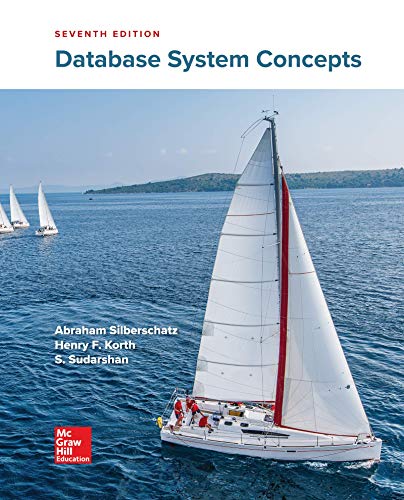
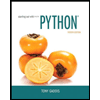
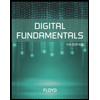
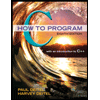
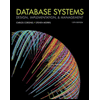
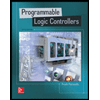