sorting the elements in descending order (highest to lowest). Then write a main program that reads a list of integers from input, stores the integers in a vector, calls SortVector(), and outputs the sorted vector. The first input integer indicates how many numbers are in the list. Ex: If the input is: 5 10 4 39 12 2 the output is: 39,12,10,4,2, For coding simplicity, follow every output value by a comma, including the last one. Your program must define and call the following function: void SortVector (vector & myVec) Hint: Sorting a vector can be done in many ways. You are welcome to look up and use any existing algorithm. Some believe the simplest to code is bubble sort: https://en.wikipedia.org/wiki/Bubble sort. But you are welcome to try others: https://en.wikipedia.org/wiki/Sorting algorithm. 555062.2603552.qx3zqy7 LAB ACTIVITY 4.11.1: LAB: Sort a vector 3/10 main.cpp Load default template... 1| 2 #include 3 #include 4 using namespace std; 5 #include 6 7 /* Define your function here */ 8 void SortVector (vector& myVec) { 9 sort (myVec.begin(), myVec.end(), greater()); 10 } 11 12 int main() { 13 /* Type your code here */ 14 int n;
sorting the elements in descending order (highest to lowest). Then write a main program that reads a list of integers from input, stores the integers in a vector, calls SortVector(), and outputs the sorted vector. The first input integer indicates how many numbers are in the list. Ex: If the input is: 5 10 4 39 12 2 the output is: 39,12,10,4,2, For coding simplicity, follow every output value by a comma, including the last one. Your program must define and call the following function: void SortVector (vector & myVec) Hint: Sorting a vector can be done in many ways. You are welcome to look up and use any existing algorithm. Some believe the simplest to code is bubble sort: https://en.wikipedia.org/wiki/Bubble sort. But you are welcome to try others: https://en.wikipedia.org/wiki/Sorting algorithm. 555062.2603552.qx3zqy7 LAB ACTIVITY 4.11.1: LAB: Sort a vector 3/10 main.cpp Load default template... 1| 2 #include 3 #include 4 using namespace std; 5 #include 6 7 /* Define your function here */ 8 void SortVector (vector& myVec) { 9 sort (myVec.begin(), myVec.end(), greater()); 10 } 11 12 int main() { 13 /* Type your code here */ 14 int n;
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I am not sure how to get my code to work. Please help
This is my code I am using :
#include <iostream>
#include <
using namespace std;
#include <algorithm>
/* Define your function here */
void SortVector(vector<int>& myVec) {
sort(myVec.begin(), myVec.end(), greater<int>());
}
int main() {
/* Type your code here */
int n;
cin >> n;
vector<int>vec(n);
for(int i=0; i<n; i++) {
cin >> vec[i];
}
SortVector(vec);
for(int i=0; i<n; i++) {
cout << vec[i] << ",";
}
return 0;
}

Transcribed Image Text:Define a function named SortVector that takes a vector of integers as a parameter. Function SortVector() modifies the vector parameter by
sorting the elements in descending order (highest to lowest). Then write a main program that reads a list of integers from input, stores the
integers in a vector, calls SortVector(), and outputs the sorted vector. The first input integer indicates how many numbers are in the list.
Ex: If the input is:
5 10 4 39 12 2
the output is:
39,12,10,4,2,
For coding simplicity, follow every output value by a comma, including the last one.
Your program must define and call the following function:
void SortVector (vector<int> & myVec)
Hint: Sorting a vector can be done in many ways. You are welcome to look up and use any existing algorithm. Some believe the simplest to
code is bubble sort: https://en.wikipedia.org/wiki/Bubblesort. But you are welcome to try others:
https://en.wikipedia.org/wiki/Sorting_algorithm.
555062.2603552.qx3zqy?
LAB
ACTIVITY
4.11.1: LAB: Sort a vector
3/10
main.cpp
Load default template...
1|
2 #include <iostream>
3 #include <vector>
4 using namespace std;
5 #include <algorithm>
6
7 /* Define your function here */
8 void SortVector (vector<int> & myVec) {
9 sort (myVec.begin(), myVec.end(), greater<int>());
10 }
11
12 int main() {
13
14
/* Type your code here */
int n;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
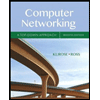
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
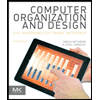
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
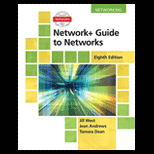
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
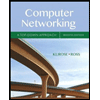
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
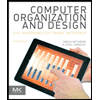
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
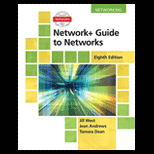
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
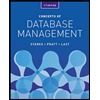
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
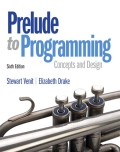
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
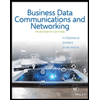
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY