2 Extend the PriorityQueue class: 2.1 Write code for the method void clear() which empties the heap and resets the size to 0. 2.2 Write code for the method Boolean isEmpty () that checks whether the heap contains any elements or not. 2.3 Write code for the method Boolean add (Node x) which adds a new node to the heap 2.4 Write code for the method Node remove ( ) which deletes the root, decreases the heap size and reorders the remaining heap. It should involve a call to a method percolate Down (int hole). 3 2.1 Create a file Main.java in which to write your code for this question. 2.2 Write code for a main method to build a Min Heap from the data in flights.txt You will need to write code that performs String pre-processing. This means transform the raw data into the appropriate Node attributes and calculate the arrival time, before creating a Node and adding it to the Heap. The heap should be ordered by arrival time. 2.3 In the main method, print out the order in which incoming flights should land. Sample data: flights.txt registration_no, departure_timestamp, flight duration (hh:mm:ss) ZA7117,14:01:36,3:33:22 ZA3642,18:06:20,5:33:59 ZA5453,23:43:56,4:21:13 ZA0559,16:09:05,8:58:24 ZA6903,13:54:48, 11:59:58 ZA9604,12:29:53,9:09:07 ZA5348,2:59:13, 6:23:48 ZA3835,8:42:41,14:55:44 ZA4949, 12:42:13,3:05:13 ZA2951,23:40:10,8:05:41 ZA4940, 9:11:30, 12:25:47 ZA3121,4:42:50, 7:53:03 ZA6470,22:59:30, 10:03:19 ZA4981, 19:37:53,12:58:38 ZA0647,9:19:03,14:50:22 ZA5632,12:23:53,1:46:22 ZA2321,20:46:09,5:07:25 ZA3408,22:48:49,1:17:55 ZA2851,4:28:12,13:45:53 ZA7759,18:35:41,4:25:57 setting up Study the class definitions below: class Node // attributes String flight_no; // flight number Date arrival time; // time at which flight lands class PriorityQueue // attributes int currentSize; Node root; FlightNode[] array new Node [25]; // methods void clear(); Boolean isEmpty(); Boolean add (Node x); Node remove(); void percolate Down (int hole); .1 Create two files Node.java and PriorityQueue.java .2 Copy the above definitions into the corresponding files .3 Write code for a default and a loaded constructor for Node .4 Write code for a default and a loaded constructor for PriorityQueue .5 Write accessor and mutator methods for the attributes of Node .6 Write accessor and mutator methods for the attributes of PriorityQueue
2 Extend the PriorityQueue class: 2.1 Write code for the method void clear() which empties the heap and resets the size to 0. 2.2 Write code for the method Boolean isEmpty () that checks whether the heap contains any elements or not. 2.3 Write code for the method Boolean add (Node x) which adds a new node to the heap 2.4 Write code for the method Node remove ( ) which deletes the root, decreases the heap size and reorders the remaining heap. It should involve a call to a method percolate Down (int hole). 3 2.1 Create a file Main.java in which to write your code for this question. 2.2 Write code for a main method to build a Min Heap from the data in flights.txt You will need to write code that performs String pre-processing. This means transform the raw data into the appropriate Node attributes and calculate the arrival time, before creating a Node and adding it to the Heap. The heap should be ordered by arrival time. 2.3 In the main method, print out the order in which incoming flights should land. Sample data: flights.txt registration_no, departure_timestamp, flight duration (hh:mm:ss) ZA7117,14:01:36,3:33:22 ZA3642,18:06:20,5:33:59 ZA5453,23:43:56,4:21:13 ZA0559,16:09:05,8:58:24 ZA6903,13:54:48, 11:59:58 ZA9604,12:29:53,9:09:07 ZA5348,2:59:13, 6:23:48 ZA3835,8:42:41,14:55:44 ZA4949, 12:42:13,3:05:13 ZA2951,23:40:10,8:05:41 ZA4940, 9:11:30, 12:25:47 ZA3121,4:42:50, 7:53:03 ZA6470,22:59:30, 10:03:19 ZA4981, 19:37:53,12:58:38 ZA0647,9:19:03,14:50:22 ZA5632,12:23:53,1:46:22 ZA2321,20:46:09,5:07:25 ZA3408,22:48:49,1:17:55 ZA2851,4:28:12,13:45:53 ZA7759,18:35:41,4:25:57 setting up Study the class definitions below: class Node // attributes String flight_no; // flight number Date arrival time; // time at which flight lands class PriorityQueue // attributes int currentSize; Node root; FlightNode[] array new Node [25]; // methods void clear(); Boolean isEmpty(); Boolean add (Node x); Node remove(); void percolate Down (int hole); .1 Create two files Node.java and PriorityQueue.java .2 Copy the above definitions into the corresponding files .3 Write code for a default and a loaded constructor for Node .4 Write code for a default and a loaded constructor for PriorityQueue .5 Write accessor and mutator methods for the attributes of Node .6 Write accessor and mutator methods for the attributes of PriorityQueue
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please assist with this practice 3 questions for java coding. Please provide all the necessary details/answers.(This is not a graded test/assignment)

Transcribed Image Text:2
Extend the PriorityQueue class:
2.1 Write code for the method void clear() which empties the heap and resets the
size to 0.
2.2 Write code for the method Boolean isEmpty () that checks whether the heap
contains any elements or not.
2.3 Write code for the method Boolean add (Node x) which adds a new node to
the heap
2.4 Write code for the method Node remove ( ) which deletes the root, decreases the
heap size and reorders the remaining heap. It should involve a call to a method
percolate Down (int hole).
3
2.1 Create a file Main.java in which to write your code for this question.
2.2 Write code for a main method to build a Min Heap from the data in flights.txt
You will need to write code that performs String pre-processing. This means transform
the raw data into the appropriate Node attributes and calculate the arrival time, before
creating a Node and adding it to the Heap. The heap should be ordered by arrival time.
2.3 In the main method, print out the order in which incoming flights should land.
Sample data: flights.txt
registration_no, departure_timestamp, flight duration (hh:mm:ss)
ZA7117,14:01:36,3:33:22
ZA3642,18:06:20,5:33:59
ZA5453,23:43:56,4:21:13
ZA0559,16:09:05,8:58:24
ZA6903,13:54:48, 11:59:58
ZA9604,12:29:53,9:09:07
ZA5348,2:59:13, 6:23:48
ZA3835,8:42:41,14:55:44
ZA4949, 12:42:13,3:05:13
ZA2951,23:40:10,8:05:41
ZA4940, 9:11:30, 12:25:47
ZA3121,4:42:50, 7:53:03
ZA6470,22:59:30, 10:03:19
ZA4981, 19:37:53,12:58:38
ZA0647,9:19:03,14:50:22
ZA5632,12:23:53,1:46:22
ZA2321,20:46:09,5:07:25
ZA3408,22:48:49,1:17:55
ZA2851,4:28:12,13:45:53
ZA7759,18:35:41,4:25:57
![setting up
Study the class definitions below:
class Node
// attributes
String flight_no; // flight number
Date arrival time; // time at which flight lands
class PriorityQueue
// attributes
int currentSize;
Node root;
FlightNode[] array new Node [25];
// methods
void clear();
Boolean isEmpty();
Boolean add (Node x);
Node remove();
void percolate Down (int hole);
.1 Create two files Node.java and PriorityQueue.java
.2 Copy the above definitions into the corresponding files
.3 Write code for a default and a loaded constructor for Node
.4 Write code for a default and a loaded constructor for PriorityQueue
.5 Write accessor and mutator methods for the attributes of Node
.6 Write accessor and mutator methods for the attributes of PriorityQueue](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F92865db7-12e1-4656-9453-9946f7baa49c%2F5ecbeb06-bf3c-41a3-9ded-6137b69f0d2f%2F91f9mnre_processed.jpeg&w=3840&q=75)
Transcribed Image Text:setting up
Study the class definitions below:
class Node
// attributes
String flight_no; // flight number
Date arrival time; // time at which flight lands
class PriorityQueue
// attributes
int currentSize;
Node root;
FlightNode[] array new Node [25];
// methods
void clear();
Boolean isEmpty();
Boolean add (Node x);
Node remove();
void percolate Down (int hole);
.1 Create two files Node.java and PriorityQueue.java
.2 Copy the above definitions into the corresponding files
.3 Write code for a default and a loaded constructor for Node
.4 Write code for a default and a loaded constructor for PriorityQueue
.5 Write accessor and mutator methods for the attributes of Node
.6 Write accessor and mutator methods for the attributes of PriorityQueue
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
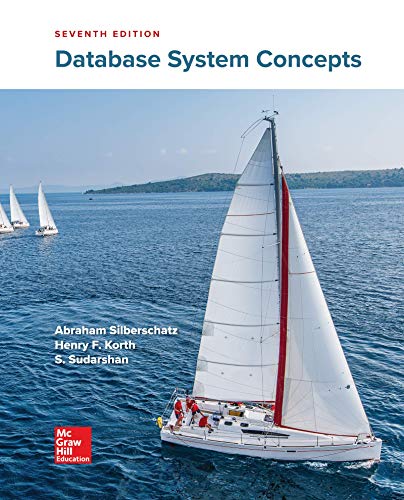
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
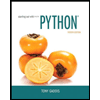
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
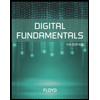
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
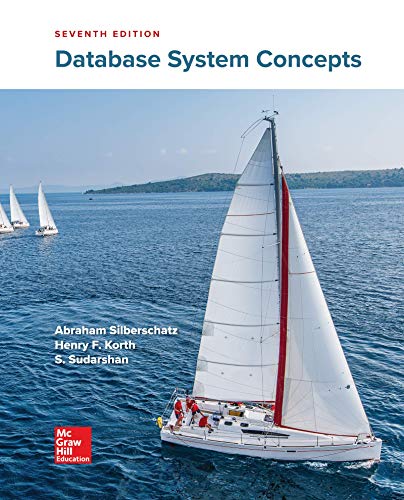
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
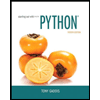
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
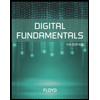
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
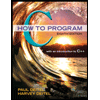
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
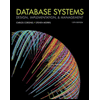
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
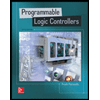
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education