Sales.java contains a Java program that prompts for and reads in the sales for each of 5 salespeople in a company. Now modify the program as follows: Declare an array called sales to save the sales of 5 salespeople. Complete the header of the first for loop. This loop initializes the array. Complete the header of the second for loop. This loop computes the total of array elements. Add code to compute and print the average sale. Add another loop to print the id of each salesperson and the number of their sales. The salespeople are objecting to having an id of 0—no one wants that designation. Modify your program so that the IDs run from 1-5 instead of 0-4. // *************************************************************** // Sales.java // // Reads in and stores sales for each of 5 salespeople. Displays // sales entered by salesperson id and total sales for all salespeople. // // *************************************************************** import java.util.Scanner; public class Sales { public static void main(String[] args) { final int SALESPEOPLE = 5; // Your code 1: declare the array called “sales” Scanner scan = new Scanner(System.in); // fill in the header of the for loop for (YOUR CODE HERE 2) { System.out.print("Enter sales for salesperson " + i + ": "); // Your code: read in the sales value for next salesperson } System.out.println("\nSalesperson Sales"); System.out.println(" ------------------ "); // fill in the condition of the for loop for (YOUR CODE HERE 3) { //YOUR CODE HERE 4, update a running total } // YOUR CODE HERE 4: print out the total // YOUR CODE HERE 4: print out the average // YOUR CODE HERE 5: add another loop } }
Sales.java contains a Java program that prompts for and reads in the sales for each of 5 salespeople in a company. Now modify the program as follows:
-
Declare an array called sales to save the sales of 5 salespeople.
-
Complete the header of the first for loop. This loop initializes the array.
-
Complete the header of the second for loop. This loop computes the total of array elements.
-
Add code to compute and print the average sale.
-
Add another loop to print the id of each salesperson and the number of their sales.
-
The salespeople are objecting to having an id of 0—no one wants that designation. Modify your program so that the IDs run from 1-5 instead of 0-4.
// ***************************************************************
// Sales.java
//
// Reads in and stores sales for each of 5 salespeople. Displays
// sales entered by salesperson id and total sales for all salespeople.
//
// ***************************************************************
import java.util.Scanner;
public class Sales
{
public static void main(String[] args)
{
final int SALESPEOPLE = 5;
// Your code 1: declare the array called “sales”
Scanner scan = new Scanner(System.in);
// fill in the header of the for loop
for (YOUR CODE HERE 2)
{
System.out.print("Enter sales for salesperson " + i + ": ");
// Your code: read in the sales value for next salesperson
}
System.out.println("\nSalesperson Sales");
System.out.println(" ------------------ ");
// fill in the condition of the for loop
for (YOUR CODE HERE 3)
{
//YOUR CODE HERE 4, update a running total
}
// YOUR CODE HERE 4: print out the total
// YOUR CODE HERE 4: print out the average
// YOUR CODE HERE 5: add another loop
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

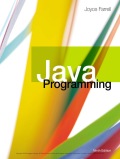
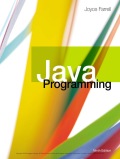