public static void calculateTurnScore(int scoreOption) { //*** //*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE //*** //*** 1) Write a switch statement with the switch-expression being on variable "scoreOption". //*** 2) The cases being 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13 //*** NOTE: These case values are not wrapped in quotes because variable "scoreOption" is of int datatype. //*** A) When case 1: //*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "aces". //*** B) When case 2: //*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "twos". //*** C) When case 3: //*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "threes". //*** D) When case 4: //*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "fours". //*** E) When case 5: //*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "fives". //*** F) When case 6: //*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "sixes". //*** G) When case 7: //*** a) Call method "calculateThreeOfKind" passing no arguments, assigning this method call to variable //*** "threeKind". //*** H) When case 8: //*** a) Call method "calculateFourOfKind" passing no arguments, assigning this method call to variable //*** "fourKind". //*** I) When case 9: //*** a) Call method "calculateFullHouse" passing no arguments, assigning this method call to variable //*** "fullHouse". //*** J) When case 10: //*** a) Call method "calculateSmallStraight" passing no arguments, assigning this method call to variable //*** "smallStraight". //*** K) When case 11: //*** a) Call method "calculateLargeStraight" passing no arguments, assigning this method call to variable //*** "largeStraight". //*** L) When case 12, use the following code: //*** //*** int checkYahtzee = calculateYahtzee(); //*** //*** if (yahtzee == SCORE_NO_VALUE) //*** yahtzee = checkYahtzee; //*** else if (yahtzee == YAHTZEE_SCORE && checkYahtzee == YAHTZEE_SCORE) //*** if (yahtzeeBonusCount == SCORE_NO_VALUE) //*** yahtzeeBonusCount = 1; //*** else //*** yahtzeeBonusCount++; //*** break; //*** //*** M) When case 13: //*** a) Call method "calculateChance" passing no arguments, assigning this method call to variable //*** "chance". //*** //*** NOTE: Include a break statement for every case. //*** //*** //*** Your code for Steps 1 and 2 goes here. //*** }
Hello! I am having trouble on how to write out the Java code based on the instructions (in bold //***) that I am given. As a note, this specific Java code is a code that is going to setup the basic framework for a game of Yahtzee. In other words, you will need to understand the rules of Yahtzee and implement the rules into coding. I appreciate the help. Thanks! Also, I am using the IntelliJ IDEA application when writing out this program. Here is what I need help with:
public static void calculateTurnScore(int scoreOption) {
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** 1) Write a switch statement with the switch-expression being on variable "scoreOption".
//*** 2) The cases being 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13
//*** NOTE: These case values are not wrapped in quotes because variable "scoreOption" is of int datatype.
//*** A) When case 1:
//*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "aces".
//*** B) When case 2:
//*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "twos".
//*** C) When case 3:
//*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "threes".
//*** D) When case 4:
//*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "fours".
//*** E) When case 5:
//*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "fives".
//*** F) When case 6:
//*** a) Call method "calculateUpperSectionCategory" passing variable "scoreOption" as an argument, //*** assigning this method call to variable "sixes".
//*** G) When case 7:
//*** a) Call method "calculateThreeOfKind" passing no arguments, assigning this method call to variable //*** "threeKind".
//*** H) When case 8:
//*** a) Call method "calculateFourOfKind" passing no arguments, assigning this method call to variable //*** "fourKind".
//*** I) When case 9:
//*** a) Call method "calculateFullHouse" passing no arguments, assigning this method call to variable //*** "fullHouse".
//*** J) When case 10:
//*** a) Call method "calculateSmallStraight" passing no arguments, assigning this method call to variable //*** "smallStraight".
//*** K) When case 11:
//*** a) Call method "calculateLargeStraight" passing no arguments, assigning this method call to variable //*** "largeStraight".
//*** L) When case 12, use the following code:
//***
//*** int checkYahtzee = calculateYahtzee();
//***
//*** if (yahtzee == SCORE_NO_VALUE)
//*** yahtzee = checkYahtzee;
//*** else if (yahtzee == YAHTZEE_SCORE && checkYahtzee == YAHTZEE_SCORE)
//*** if (yahtzeeBonusCount == SCORE_NO_VALUE)
//*** yahtzeeBonusCount = 1;
//*** else
//*** yahtzeeBonusCount++;
//*** break;
//***
//*** M) When case 13:
//*** a) Call method "calculateChance" passing no arguments, assigning this method call to variable //*** "chance".
//***
//*** NOTE: Include a break statement for every case.
//***
//***
//*** Your code for Steps 1 and 2 goes here.
//***
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

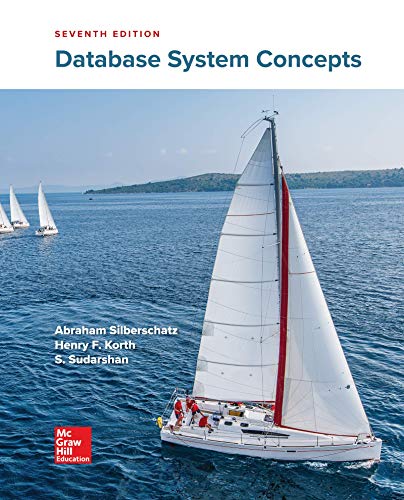
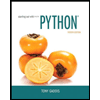
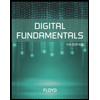
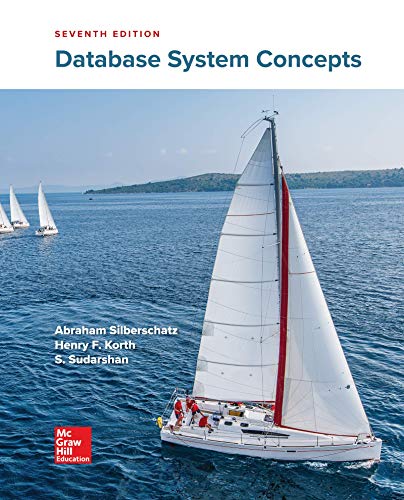
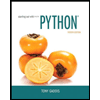
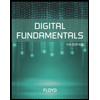
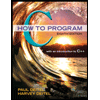
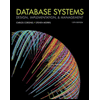
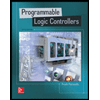