Problem: Assume that you have been hired at Oman Investment and Finance Co. SAOG (OIFC) to write a java program that prints a summary of customers' bills for water and electricity. Each bill has type, account number, consumption amount (kilowatt/gallon) and the month of the bill. Each bill charges an amount of 16.78 OMR as supply service charges. Electricity bill is either industrial or residential. The electricity total energy charge is computed based on the type of the usage. For industrial each kilowatt consumed costs 12 Baisa while for residential it costs 10 Baisa per kilowatt consumed. The total amount is the sum of the energy charges plus the bill charges. Water bill applies different rates based on the water consumption where the first 5000 gallons cost 2 Baisa per gallon and any gallon more than 5000 costs 2.5 Baisa. Water bill also includes sewage charges which is one third the water charges. The total amount is the sum of the water charges, sewage charges and the bill charges. Bill Water Electricity According to the description above, you are asked to develop an OOP solution that includes the following: [6.25 points] Class Bill which has: Variables to store bill information as described above. A constructor with parameters to initialize bill information. Accesor method for the consumption amount. getCharges() method that returns the supply service charges. Override toString() method to return a bill information line as shown in the sample output. (Check Figure 2 below) [6.75 points] Class Water which has: A constructor with parameters to initialize a water bill information. Override the getCharges method to compute the total amount according to the description above. [5.75 points] Class Electricity which has: Variables to store whether the usage is industrial or residential. A constructor with parameters to initialize an electricity bill information. Override the getCharges method to compute the total amount according to the description above. Write the code for the tester class yourNameOIFC which has: o [5.75 points] readBills method that accepts a scanner object and returns a list of bills. It reads each line from the input file and construct the corresponding bill type. [6 points] printBillsSummary method that accepts a list of bills and prints the summary including the total charges as shown in the sample output (check Figure 2 below). You should replace the string "Ahmed" with your first name. [6.5 points] main method that asks a user for input file name checks whether the file exist read from the input file objects of type Water or Electricity into an array list by calling readBills. The line for water objects starts with "W" while for electricity objects it starts with "E". Check Figure 1 for a sample input file, "bills.txt", which is also available on Moodle. calls printBillsSummary method to print the output shown in Figure 2 after reading the whole input file. Bonus (Optional) [1 point]: Print all water bills before the electricity bills as shown in Figure 3. o o
Problem: Assume that you have been hired at Oman Investment and Finance Co. SAOG (OIFC) to write a java program that prints a summary of customers' bills for water and electricity. Each bill has type, account number, consumption amount (kilowatt/gallon) and the month of the bill. Each bill charges an amount of 16.78 OMR as supply service charges. Electricity bill is either industrial or residential. The electricity total energy charge is computed based on the type of the usage. For industrial each kilowatt consumed costs 12 Baisa while for residential it costs 10 Baisa per kilowatt consumed. The total amount is the sum of the energy charges plus the bill charges. Water bill applies different rates based on the water consumption where the first 5000 gallons cost 2 Baisa per gallon and any gallon more than 5000 costs 2.5 Baisa. Water bill also includes sewage charges which is one third the water charges. The total amount is the sum of the water charges, sewage charges and the bill charges. Bill Water Electricity According to the description above, you are asked to develop an OOP solution that includes the following: [6.25 points] Class Bill which has: Variables to store bill information as described above. A constructor with parameters to initialize bill information. Accesor method for the consumption amount. getCharges() method that returns the supply service charges. Override toString() method to return a bill information line as shown in the sample output. (Check Figure 2 below) [6.75 points] Class Water which has: A constructor with parameters to initialize a water bill information. Override the getCharges method to compute the total amount according to the description above. [5.75 points] Class Electricity which has: Variables to store whether the usage is industrial or residential. A constructor with parameters to initialize an electricity bill information. Override the getCharges method to compute the total amount according to the description above. Write the code for the tester class yourNameOIFC which has: o [5.75 points] readBills method that accepts a scanner object and returns a list of bills. It reads each line from the input file and construct the corresponding bill type. [6 points] printBillsSummary method that accepts a list of bills and prints the summary including the total charges as shown in the sample output (check Figure 2 below). You should replace the string "Ahmed" with your first name. [6.5 points] main method that asks a user for input file name checks whether the file exist read from the input file objects of type Water or Electricity into an array list by calling readBills. The line for water objects starts with "W" while for electricity objects it starts with "E". Check Figure 1 for a sample input file, "bills.txt", which is also available on Moodle. calls printBillsSummary method to print the output shown in Figure 2 after reading the whole input file. Bonus (Optional) [1 point]: Print all water bills before the electricity bills as shown in Figure 3. o o
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Problem:
Assume that you have been hired at Oman Investment and Finance Co. SAOG (OIFC) to write a java
program that prints a summary of customers' bills for water and electricity. Each bill has type, account
number, consumption amount (kilowatt/gallon) and the month of the bill. Each bill charges an amount
of 16.78 OMR as supply service charges. Electricity bill is either industrial or residential. The electricity
total energy charge is computed based on the type of the usage. For industrial each kilowatt consumed
costs 12 Baisa while for residential it costs 10 Baisa per kilowatt consumed. The total amount is the
sum of the energy charges plus the bill charges. Water bill applies different rates based on the water
consumption where the first 5000 gallons cost 2 Baisa per gallon and any gallon more than 5000 costs
2.5 Baisa. Water bill also includes sewage charges which is one third the water charges. The total
amount is the sum of the water charges, sewage charges and the bill charges.
Bill
Water
Electricity
According to the description above, you are asked to develop an OOP solution that includes the
following:
[6.25 points] Class Bill which has:
Variables to store bill information as described above.
A constructor with parameters to initialize bill information.
Accesor method for the consumption amount.
getCharges() method that returns the supply service charges.
Override toString( ) method to return a bill information line as shown in the sample
output. (Check Figure 2 below)
[6.75 points] Class Water which has:
A constructor with parameters to initialize a water bill information.
Override the getCharges method to compute the total amount according to the description
above.
[5.75 points] Class Electricity which has:
Variables to store whether the usage is industrial or residential.
A constructor with parameters to initialize an electricity bill information.
Override the getCharges method to compute the total amount according to the description
above.
Write the code for the tester class yourNameOIFC which has:
[5.75 points] readBills method that accepts a scanner object and returns a list of bills. It
reads each line from the input file and construct the corresponding bill type.
o [6 points] printBillsSummary method that accepts a list of bills and prints the summary
including the total charges as shown in the sample output (check Figure 2 below). You
should replace the string "Ahmed" with your first name.
[6.5 points] main method that
asks a user for input file name
checks whether the file exist
read from the input file objects of type Water or Electricity into an array list by
calling readBills. The line for water objects starts with "W" while for electricity
objects it starts with "E". Check Figure 1 for a sample input file, “bills.txt", which
is also available on Moodle.
calls printBillsSummary method to print the output shown in Figure 2 after reading
the whole input file.
Bonus (Optional) [1 point]: Print all water bills before the electricity bills as shown in Figure 3.
A elearn.squ.edu.om](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5101b5a6-2e37-422b-85f3-a3d6cf4a77ff%2F2d97cd64-04c4-4895-9723-eeefcca4dbf9%2Fif8lihb_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Problem:
Assume that you have been hired at Oman Investment and Finance Co. SAOG (OIFC) to write a java
program that prints a summary of customers' bills for water and electricity. Each bill has type, account
number, consumption amount (kilowatt/gallon) and the month of the bill. Each bill charges an amount
of 16.78 OMR as supply service charges. Electricity bill is either industrial or residential. The electricity
total energy charge is computed based on the type of the usage. For industrial each kilowatt consumed
costs 12 Baisa while for residential it costs 10 Baisa per kilowatt consumed. The total amount is the
sum of the energy charges plus the bill charges. Water bill applies different rates based on the water
consumption where the first 5000 gallons cost 2 Baisa per gallon and any gallon more than 5000 costs
2.5 Baisa. Water bill also includes sewage charges which is one third the water charges. The total
amount is the sum of the water charges, sewage charges and the bill charges.
Bill
Water
Electricity
According to the description above, you are asked to develop an OOP solution that includes the
following:
[6.25 points] Class Bill which has:
Variables to store bill information as described above.
A constructor with parameters to initialize bill information.
Accesor method for the consumption amount.
getCharges() method that returns the supply service charges.
Override toString( ) method to return a bill information line as shown in the sample
output. (Check Figure 2 below)
[6.75 points] Class Water which has:
A constructor with parameters to initialize a water bill information.
Override the getCharges method to compute the total amount according to the description
above.
[5.75 points] Class Electricity which has:
Variables to store whether the usage is industrial or residential.
A constructor with parameters to initialize an electricity bill information.
Override the getCharges method to compute the total amount according to the description
above.
Write the code for the tester class yourNameOIFC which has:
[5.75 points] readBills method that accepts a scanner object and returns a list of bills. It
reads each line from the input file and construct the corresponding bill type.
o [6 points] printBillsSummary method that accepts a list of bills and prints the summary
including the total charges as shown in the sample output (check Figure 2 below). You
should replace the string "Ahmed" with your first name.
[6.5 points] main method that
asks a user for input file name
checks whether the file exist
read from the input file objects of type Water or Electricity into an array list by
calling readBills. The line for water objects starts with "W" while for electricity
objects it starts with "E". Check Figure 1 for a sample input file, “bills.txt", which
is also available on Moodle.
calls printBillsSummary method to print the output shown in Figure 2 after reading
the whole input file.
Bonus (Optional) [1 point]: Print all water bills before the electricity bills as shown in Figure 3.
A elearn.squ.edu.om

Transcribed Image Text:Water
A bills.txt
W, 12387844,January,4786
E, 12387844, January, 3872,"YES"
w, 86787642, March, 5876
W, 77868213,April, 7342
E, 89782421, March, 3982, "NO"
w, 87981243, April,8231
w, 98798273, February, 3283
W, 12387844, March, 2787
E, 12387844, April,4872, "N0"
E, 80823134, December,5983.2,"YES"
Figure 1: Sample input file “bills.txt". YES means industrial usage.
run:
Enter file name:> data.txt
Sorry! data. txt not found. Try again.
Enter file name:> bills.in
Sorry! bills.in not found. Try again.
Enter file name:> bills.txt
Show your name here
Ahmed's OIFC
Bills
May 2, 2021
|Туре
Account #
Month
Consumption
Amount
Water
Electricity
Water
4786.00
3872.00
5876.00
7342.00
29.543
55.500
33.033
37.920
56.600
40.883
25.535
24.212
12387844
12387844
86787642
77868213
89782421
87981243
98798273
12387844
January
January
March
April
March
April
February
Water
Electricity
Water
Water
Water
3982.00
8231.00
3283.00
2787.00
4872.00
5983.20
March
Electricity
Electricity
12387844
80823134
April
December
65.500
76.612
Total
445.34 OMR
BUILD SUCCESSFUL (total time: 11 seconds)
Figure 2: Sample output for input file “bills.txt"
run:
Enter file name:> bills.txt
Ahmed's OIFC
Bills
May 2, 2021
Туре
Account #
Month
Consumption
Amount
12387844
2787.00
3283.00
Water
March
24.212
25.535
February
April
April
March
January
January
March
April
December
Water
98798273
87981243
8231.00
40.883
Water
Water
Water
77868213
7342.00
37.920
33.033
86787642
5876.00
4786.00
3872.00
3982.00
4872.00
5983.20
12387844
29.543
רםכ
12387844
Electricity
Electricity
Electricity
Electricity
55.500
56.600
65.500
89782421
12387844
80823134
76.612
Total
BUILD SUCCESSFUL (total time: 4 seconds)
445.34 OMR
Figure 3: Sample output for input file “bills.txt" where water bills are printed before electricity bills.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
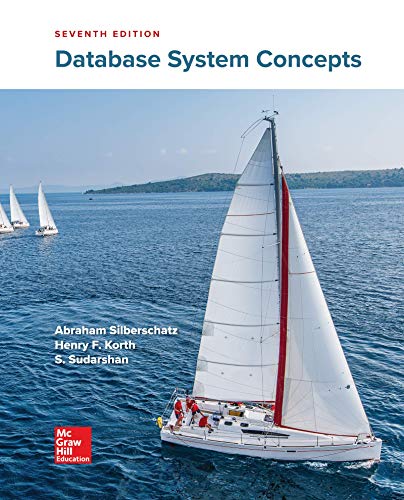
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
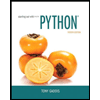
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
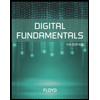
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
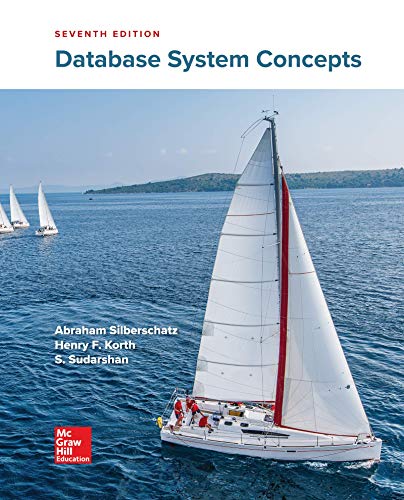
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
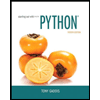
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
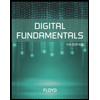
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
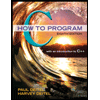
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
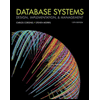
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
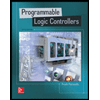
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education