Please convert the code to C language //separate chaining #include using namespace std; class node { public: int data; node* next; node() { data = 0; next = NULL; } node(int x) { data = x; next = NULL; } }; node* add(node* head, int data) { if (head == NULL) { head = new node(data); return head; } node* temp = head; while (temp->next) { temp = temp->next; } temp->next = new node(data); return head; } void print(node* head) { if (!head) { cout << "NULL\n"; return; } node* temp = head; while (temp) { cout << temp->data << "->"; temp = temp->next; } cout << "NULL\n"; } int main() { //set of input numbers vector arr{ 123, 456, 763, 656, 908, 238, 231 }; //initialize the hash table //each entry of the hash table is a linkedlist vector hash(10); //size of hashtable is 10 //using hash function f(x)=no of digits in x for (int a : arr) { hash[a % 10] = add(hash[a % 10], a); } cout << "Hash table is:\n"; for (int i = 0; i < 10; i++) { cout << i << "---- "; print(hash[i]); } return 0; } Output:
Please convert the code to C language
//separate chaining
#include <bits/stdc++.h>
using namespace std;
class node {
public:
int data;
node* next;
node()
{
data = 0;
next = NULL;
}
node(int x)
{
data = x;
next = NULL;
}
};
node* add(node* head, int data)
{
if (head == NULL) {
head = new node(data);
return head;
}
node* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = new node(data);
return head;
}
void print(node* head)
{
if (!head) {
cout << "NULL\n";
return;
}
node* temp = head;
while (temp) {
cout << temp->data << "->";
temp = temp->next;
}
cout << "NULL\n";
}
int main()
{
//set of input numbers
//initialize the hash table
//each entry of the hash table is a linkedlist
vector<node*> hash(10); //size of hashtable is 10
//using hash function f(x)=no of digits in x
for (int a : arr) {
hash[a % 10] = add(hash[a % 10], a);
}
cout << "Hash table is:\n";
for (int i = 0; i < 10; i++) {
cout << i << "---- ";
print(hash[i]);
}
return 0;
}
Output:


Step by step
Solved in 3 steps with 3 images

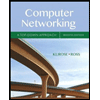
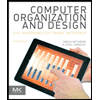
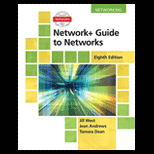
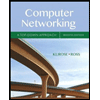
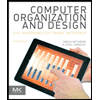
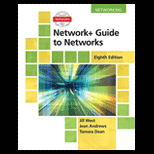
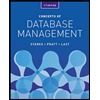
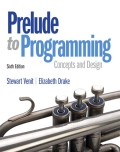
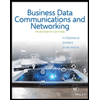