Output should be 5. Please help me find my error, and create 5 tests below in a seperate file using the corrected code. Thanks. 1. create a strawberryStand object 2. create one or more MenuItem objects and add them to the strawberryStand's menu 3. create a dictionary of sales for the day that includes sales of at least one item that isn't in the menu 4. try calling enter_sales_for_today(), passing that sales dictionary as the argument 5. If an InvalidSalesItem is raised, it should be caught with a try/except that prints an explanatory message for the user (otherwise the function should proceed normally). class MenuItem: def __init__(self, name, wholesale_cost, selling_price): self.name = name self.wholesale_cost = wholesale_cost self.selling_price = selling_price def get_name(self): return self.name def get_wholesale_cost(self): return self.wholesale_cost def get_selling_price(self): return self.selling_price class SalesForDay: def __init__(self, day, sales_dict): self.day = day self.sales_dict = sales_dict def get_day(self): return self.day def get_sales_dict(self): return self.sales_dict class StrawberryStand: def __init__(self, name): self.name = name self.current_day = 0 self.menu = {} self.sales_record = [] def get_name(self): return self.name def add_menu_item(self, menu_item): self.menu[menu_item.get_name()] = menu_item def enter_sales_for_today(self, sales_dict): for key in sales_dict: if key not in self.menu: raise InvalidSalesItemError self.sales_record.append(SalesForDay(self.current_day, sales_dict)) self.current_day += 1 def get_sales_dict_for_day(self, day): for sales_day in self.sales_record: if sales_day.get_day() == day: return sales_day.get_sales_dict() def total_sales_for_menu_item(self, menu_item_name): total_sales = 0 for sales_day in self.sales_record: total_sales += sales_day.get_sales_dict()[menu_item_name] return total_sales def total_profit_for_menu_item(self, menu_item_name): return self.menu[menu_item_name].get_selling_price() * self.total_sales_for_menu_item(menu_item_name) def total_profit_for_stand(self): total_profit = 0 for key in self.menu: total_profit += self.total_profit_for_menu_item(key) return total_profit stand = StrawberryStand('Straw R Us') item1 = MenuItem('Strawberry', .5, 1.5) stand.add_menu_item(item1) item2 = MenuItem('cake', .2, 1) stand.add_menu_item(item2); day0 = { 'Strawberry': 5, 'cake': 2 } stand.enter_sales_for_today(day0) print(f"Strawberry profit = {stand.total_profit_for_menu_item('Strawberry')}")
Output should be 5. Please help me find my error, and create 5 tests below in a seperate file using the corrected code. Thanks.
1. create a strawberryStand object
2. create one or more MenuItem objects and add them to the strawberryStand's menu
3. create a dictionary of sales for the day that includes sales of at least one item that isn't in the menu
4. try calling enter_sales_for_today(), passing that sales dictionary as the argument
5. If an InvalidSalesItem is raised, it should be caught with a try/except that prints an explanatory message for the user (otherwise the function should proceed normally).
class MenuItem:
def __init__(self, name, wholesale_cost, selling_price):
self.name = name
self.wholesale_cost = wholesale_cost
self.selling_price = selling_price
def get_name(self):
return self.name
def get_wholesale_cost(self):
return self.wholesale_cost
def get_selling_price(self):
return self.selling_price
class SalesForDay:
def __init__(self, day, sales_dict):
self.day = day
self.sales_dict = sales_dict
def get_day(self):
return self.day
def get_sales_dict(self):
return self.sales_dict
class StrawberryStand:
def __init__(self, name):
self.name = name
self.current_day = 0
self.menu = {}
self.sales_record = []
def get_name(self):
return self.name
def add_menu_item(self, menu_item):
self.menu[menu_item.get_name()] = menu_item
def enter_sales_for_today(self, sales_dict):
for key in sales_dict:
if key not in self.menu:
raise InvalidSalesItemError
self.sales_record.append(SalesForDay(self.current_day, sales_dict))
self.current_day += 1
def get_sales_dict_for_day(self, day):
for sales_day in self.sales_record:
if sales_day.get_day() == day:
return sales_day.get_sales_dict()
def total_sales_for_menu_item(self, menu_item_name):
total_sales = 0
for sales_day in self.sales_record:
total_sales += sales_day.get_sales_dict()[menu_item_name]
return total_sales
def total_profit_for_menu_item(self, menu_item_name):
return self.menu[menu_item_name].get_selling_price() * self.total_sales_for_menu_item(menu_item_name)
def total_profit_for_stand(self):
total_profit = 0
for key in self.menu:
total_profit += self.total_profit_for_menu_item(key)
return total_profit
stand = StrawberryStand('Straw R Us')
item1 = MenuItem('Strawberry', .5, 1.5)
stand.add_menu_item(item1)
item2 = MenuItem('cake', .2, 1)
stand.add_menu_item(item2);
day0 = {
'Strawberry': 5,
'cake': 2
}
stand.enter_sales_for_today(day0)
print(f"Strawberry profit = {stand.total_profit_for_menu_item('Strawberry')}")

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

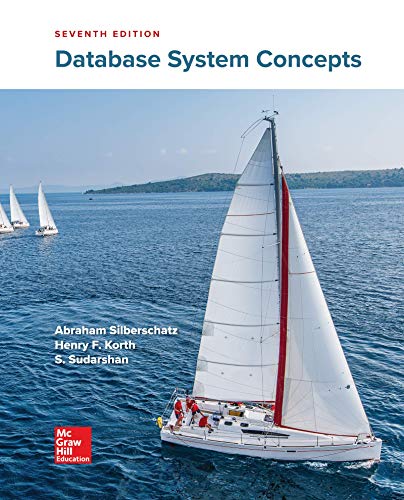
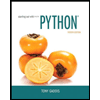
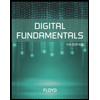
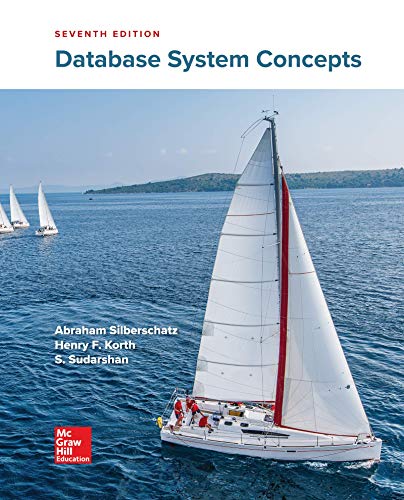
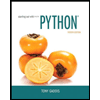
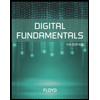
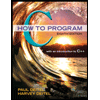
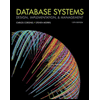
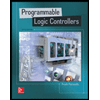