on PYTHON \-----> 13 # set the title and x_label, y_label for temp graph_drawi... wind_temp.txt 1 import matplotlib.pyplot as plt 2 3 # A function to draw a line graph based on the given data 4 def draw_graph (dic_data): 14 plt.title("Temperature") 5 plt.figure() 6 15 plt.xlabel("Day") days = list(dic_data.keys()) 7 16 plt.ylabel("Celsius") values = list(dic_data.values()) 8 17 plt.savefig('temp_line.png') temps = [two_tuple [0] for two_tuple in values] 9 18 plt.clf() # clears figure for next plot winds = [two_tuple [1] for two_tuple in values] 10 19 11 20 21 # Plots winds as function of days in another plot file plt.plot(days, winds) 12 # Plots temps as function of days in one plot file plt.plot(days, temps) 13 22 23 24 25 26 # set the title and x_label, y_label for wind plt.title("Wind Speed") plt.xlabel("Day") plt.ylabel("km/h") plt.savefig('wind_line.png') You should complete only one code block: the function get_valid_data_source The function get_valid_data_source should open and read the data from wind_temp.txt, where the first column of comma-delimited values represents the day, the second column represents the recorded temperature, and the third column represents the recorded wind speed. I.e. each line should be stored as a key-value pair in a dictionary dic_data, where each key should be the day and each value should be a tuple containing the temperature and the wind speed. The function closes the file and returns the dic_data that will be passed back to the try block. DO NOT CHANGE the try and except in your workspace You MUST use open(), read (), close(), split(), and int(). wind_temp.txt 26 27 # set the title and x_label, y_label for wind plt.title("Wind Speed") plt.xlabel("Day") plt.ylabel("km/h") plt.savefig('wind_line.png') 28 ### DO NOT CHANGE THE ABOVE CODE! %#2## 29 30 def get_valid_data_source(filename): # Task: Complete the function 31 32 33 34 14 plt.title("Temperature") 15 16 17 18 # set the title and x_label, y_label for temp plt.xlabel("Day") plt.ylabel("Celsius") plt.savefig('temp_line.png') plt.clf() # clears figure for next plot 19 20 21 # Plots winds as function of days in another plot file plt.plot(days, winds) 22 23 24 25 1 1,21,42 2 2,21,37 3 3,22,43 4 4,22,37 5 5,23,39 35 ### Do NOT CHANGE THE FOLLOWING try AND except BLOCKS #2## 36 try: 37 filename = input("What is the name of the text file you are using for graph drawing? ") dic_data = get_valid_data_source(filename) 38 39 draw_graph (dic_data) 40 except FileNotFoundError as e: 41 print("There's no such file found: {}, please choose from the given options.".format(filename)) 6 6,25,40 7,30,39 8 8,22,35 9 9,24,39 10 10,22,43 11 11,21,33 Your program should behave according to these two criteria: [user@sahara ~]$ python3 graph_drawing_tool.py What is the name of the text file you are using for graph drawing? wind_temp.txt 1) When you call your program with the filename wind_temp.txt, it should output the following two files. The first plot (temp_line.png) should look exactly like this: Temperature 30 28 26 24 22 8 9 10 11 Day The second plot (wind_line.png) should look exactly like this: Wind Speed 36 34 1 2 5 6 9 10 11 Day 2) When the user enters some filename that does not exist, an error message is printed by the exception block: [user@sahara ~]$ python3 graph_drawing_tool.py What is the name of the text file you are using for graph drawing? sensor.txt /home/graph_drawing_tool.py Spaces: 4 (Auto) Terminal
on PYTHON \-----> 13 # set the title and x_label, y_label for temp graph_drawi... wind_temp.txt 1 import matplotlib.pyplot as plt 2 3 # A function to draw a line graph based on the given data 4 def draw_graph (dic_data): 14 plt.title("Temperature") 5 plt.figure() 6 15 plt.xlabel("Day") days = list(dic_data.keys()) 7 16 plt.ylabel("Celsius") values = list(dic_data.values()) 8 17 plt.savefig('temp_line.png') temps = [two_tuple [0] for two_tuple in values] 9 18 plt.clf() # clears figure for next plot winds = [two_tuple [1] for two_tuple in values] 10 19 11 20 21 # Plots winds as function of days in another plot file plt.plot(days, winds) 12 # Plots temps as function of days in one plot file plt.plot(days, temps) 13 22 23 24 25 26 # set the title and x_label, y_label for wind plt.title("Wind Speed") plt.xlabel("Day") plt.ylabel("km/h") plt.savefig('wind_line.png') You should complete only one code block: the function get_valid_data_source The function get_valid_data_source should open and read the data from wind_temp.txt, where the first column of comma-delimited values represents the day, the second column represents the recorded temperature, and the third column represents the recorded wind speed. I.e. each line should be stored as a key-value pair in a dictionary dic_data, where each key should be the day and each value should be a tuple containing the temperature and the wind speed. The function closes the file and returns the dic_data that will be passed back to the try block. DO NOT CHANGE the try and except in your workspace You MUST use open(), read (), close(), split(), and int(). wind_temp.txt 26 27 # set the title and x_label, y_label for wind plt.title("Wind Speed") plt.xlabel("Day") plt.ylabel("km/h") plt.savefig('wind_line.png') 28 ### DO NOT CHANGE THE ABOVE CODE! %#2## 29 30 def get_valid_data_source(filename): # Task: Complete the function 31 32 33 34 14 plt.title("Temperature") 15 16 17 18 # set the title and x_label, y_label for temp plt.xlabel("Day") plt.ylabel("Celsius") plt.savefig('temp_line.png') plt.clf() # clears figure for next plot 19 20 21 # Plots winds as function of days in another plot file plt.plot(days, winds) 22 23 24 25 1 1,21,42 2 2,21,37 3 3,22,43 4 4,22,37 5 5,23,39 35 ### Do NOT CHANGE THE FOLLOWING try AND except BLOCKS #2## 36 try: 37 filename = input("What is the name of the text file you are using for graph drawing? ") dic_data = get_valid_data_source(filename) 38 39 draw_graph (dic_data) 40 except FileNotFoundError as e: 41 print("There's no such file found: {}, please choose from the given options.".format(filename)) 6 6,25,40 7,30,39 8 8,22,35 9 9,24,39 10 10,22,43 11 11,21,33 Your program should behave according to these two criteria: [user@sahara ~]$ python3 graph_drawing_tool.py What is the name of the text file you are using for graph drawing? wind_temp.txt 1) When you call your program with the filename wind_temp.txt, it should output the following two files. The first plot (temp_line.png) should look exactly like this: Temperature 30 28 26 24 22 8 9 10 11 Day The second plot (wind_line.png) should look exactly like this: Wind Speed 36 34 1 2 5 6 9 10 11 Day 2) When the user enters some filename that does not exist, an error message is printed by the exception block: [user@sahara ~]$ python3 graph_drawing_tool.py What is the name of the text file you are using for graph drawing? sensor.txt /home/graph_drawing_tool.py Spaces: 4 (Auto) Terminal
Chapter16: Graphics
Section: Chapter Questions
Problem 17RQ
Related questions
Question
![on
PYTHON
\----->
13
# set the title and x_label, y_label for temp
graph_drawi... wind_temp.txt
1 import matplotlib.pyplot as plt
2
3 # A function to draw a line graph based on the given data
4 def draw_graph (dic_data):
14
plt.title("Temperature")
5
plt.figure()
6
15
plt.xlabel("Day")
days = list(dic_data.keys())
7
16
plt.ylabel("Celsius")
values = list(dic_data.values())
8
17
plt.savefig('temp_line.png')
temps = [two_tuple [0] for two_tuple in values]
9
18
plt.clf() # clears figure for next plot
winds = [two_tuple [1] for two_tuple in values]
10
19
11
20
21
# Plots winds as function of days in another plot file
plt.plot(days, winds)
12
# Plots temps as function of days in one plot file
plt.plot(days, temps)
13
22
23
24
25
26
# set the title and x_label, y_label for wind
plt.title("Wind Speed")
plt.xlabel("Day")
plt.ylabel("km/h")
plt.savefig('wind_line.png')
You should complete only one code block: the function
get_valid_data_source
The function get_valid_data_source should open and read the data from
wind_temp.txt, where the first column of comma-delimited values represents
the day, the second column represents the recorded temperature, and the third
column represents the recorded wind speed. I.e. each line should be stored as a
key-value pair in a dictionary dic_data, where each key should be the day and
each value should be a tuple containing the temperature and the wind speed. The
function closes the file and returns the dic_data that will be passed back to the
try block.
DO NOT CHANGE the try and except in your workspace
You MUST use open(), read (), close(), split(), and int().
wind_temp.txt
26
27
# set the title and x_label, y_label for wind
plt.title("Wind Speed")
plt.xlabel("Day")
plt.ylabel("km/h")
plt.savefig('wind_line.png')
28 ### DO NOT CHANGE THE ABOVE CODE! %#2##
29
30 def get_valid_data_source(filename):
# Task: Complete the function
31
32
33
34
14
plt.title("Temperature")
15
16
17
18
# set the title and x_label, y_label for temp
plt.xlabel("Day")
plt.ylabel("Celsius")
plt.savefig('temp_line.png')
plt.clf() # clears figure for next plot
19
20
21
# Plots winds as function of days in another plot file
plt.plot(days, winds)
22
23
24
25
1 1,21,42
2 2,21,37
3 3,22,43
4 4,22,37
5 5,23,39
35 ### Do NOT CHANGE THE FOLLOWING try AND except BLOCKS #2##
36 try:
37 filename = input("What is the name of the text file you are using for graph drawing? ")
dic_data = get_valid_data_source(filename)
38
39
draw_graph (dic_data)
40 except FileNotFoundError as e:
41
print("There's no such file found: {}, please choose from the given options.".format(filename))
6 6,25,40
7,30,39
8 8,22,35
9 9,24,39
10 10,22,43
11 11,21,33
Your program should behave according to these two criteria:
[user@sahara ~]$ python3 graph_drawing_tool.py
What is the name of the text file you are using for graph drawing? wind_temp.txt
1) When you call your program with the filename wind_temp.txt, it should
output the following two files. The first plot (temp_line.png) should look exactly
like this:
Temperature
30
28
26
24
22
8
9 10
11
Day
The second plot (wind_line.png) should look exactly like this:
Wind Speed
36
34
1
2
5
6
9
10
11
Day
2) When the user enters some filename that does not exist, an error message is
printed by the exception block:
[user@sahara ~]$ python3 graph_drawing_tool.py
What is the name of the text file you are using for graph drawing? sensor.txt
/home/graph_drawing_tool.py Spaces: 4 (Auto)
Terminal](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb4d9d51c-cee3-4855-8494-55e8cc009ab5%2F4cd58978-20e6-4731-8c81-73c63328dde5%2Fipwvjvo_processed.png&w=3840&q=75)
Transcribed Image Text:on
PYTHON
\----->
13
# set the title and x_label, y_label for temp
graph_drawi... wind_temp.txt
1 import matplotlib.pyplot as plt
2
3 # A function to draw a line graph based on the given data
4 def draw_graph (dic_data):
14
plt.title("Temperature")
5
plt.figure()
6
15
plt.xlabel("Day")
days = list(dic_data.keys())
7
16
plt.ylabel("Celsius")
values = list(dic_data.values())
8
17
plt.savefig('temp_line.png')
temps = [two_tuple [0] for two_tuple in values]
9
18
plt.clf() # clears figure for next plot
winds = [two_tuple [1] for two_tuple in values]
10
19
11
20
21
# Plots winds as function of days in another plot file
plt.plot(days, winds)
12
# Plots temps as function of days in one plot file
plt.plot(days, temps)
13
22
23
24
25
26
# set the title and x_label, y_label for wind
plt.title("Wind Speed")
plt.xlabel("Day")
plt.ylabel("km/h")
plt.savefig('wind_line.png')
You should complete only one code block: the function
get_valid_data_source
The function get_valid_data_source should open and read the data from
wind_temp.txt, where the first column of comma-delimited values represents
the day, the second column represents the recorded temperature, and the third
column represents the recorded wind speed. I.e. each line should be stored as a
key-value pair in a dictionary dic_data, where each key should be the day and
each value should be a tuple containing the temperature and the wind speed. The
function closes the file and returns the dic_data that will be passed back to the
try block.
DO NOT CHANGE the try and except in your workspace
You MUST use open(), read (), close(), split(), and int().
wind_temp.txt
26
27
# set the title and x_label, y_label for wind
plt.title("Wind Speed")
plt.xlabel("Day")
plt.ylabel("km/h")
plt.savefig('wind_line.png')
28 ### DO NOT CHANGE THE ABOVE CODE! %#2##
29
30 def get_valid_data_source(filename):
# Task: Complete the function
31
32
33
34
14
plt.title("Temperature")
15
16
17
18
# set the title and x_label, y_label for temp
plt.xlabel("Day")
plt.ylabel("Celsius")
plt.savefig('temp_line.png')
plt.clf() # clears figure for next plot
19
20
21
# Plots winds as function of days in another plot file
plt.plot(days, winds)
22
23
24
25
1 1,21,42
2 2,21,37
3 3,22,43
4 4,22,37
5 5,23,39
35 ### Do NOT CHANGE THE FOLLOWING try AND except BLOCKS #2##
36 try:
37 filename = input("What is the name of the text file you are using for graph drawing? ")
dic_data = get_valid_data_source(filename)
38
39
draw_graph (dic_data)
40 except FileNotFoundError as e:
41
print("There's no such file found: {}, please choose from the given options.".format(filename))
6 6,25,40
7,30,39
8 8,22,35
9 9,24,39
10 10,22,43
11 11,21,33
Your program should behave according to these two criteria:
[user@sahara ~]$ python3 graph_drawing_tool.py
What is the name of the text file you are using for graph drawing? wind_temp.txt
1) When you call your program with the filename wind_temp.txt, it should
output the following two files. The first plot (temp_line.png) should look exactly
like this:
Temperature
30
28
26
24
22
8
9 10
11
Day
The second plot (wind_line.png) should look exactly like this:
Wind Speed
36
34
1
2
5
6
9
10
11
Day
2) When the user enters some filename that does not exist, an error message is
printed by the exception block:
[user@sahara ~]$ python3 graph_drawing_tool.py
What is the name of the text file you are using for graph drawing? sensor.txt
/home/graph_drawing_tool.py Spaces: 4 (Auto)
Terminal
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 1 steps

Recommended textbooks for you
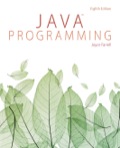
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
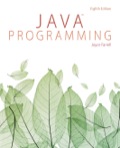
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT