n Java, Consider a crime wave during two criminals simultaneously commit crimes while two detectives simultaneously solve the crimes. A crime has an integer seriousness level between 0 (creating a public nuisance) and 4 (murder). Criminals commit crimes with random seriousness and wait a random amount of time between 0 and 100 milliseconds between crimes. Each criminal commits 50 crimes and then retires to live from the loot. Detectives solve the most serious crimes first, resting for 60 milliseconds after solving each crime. The application continues running until both the criminals have retired (ie, the two threads containing the criminal Runnables have terminated) and all the crimes have been solved. Here are the first few lines of output from a sample run of the program: c1 commits a crime of seriousness 1 d1 solves a crime of seriousness 1 c1 commits a crime of seriousness 4 d2 solves a crime of seriousness 4 c2 commits a crime of seriousness 0 c2 commits a crime of seriousness 0 Here are the last few lines of output from a typical run: d1 solves a crime of seriousness 0 d2 solves a crime of seriousness 0 committed: 100 solved: 100 Complete the missing code: package crime; import java.util.PriorityQueue; import java.util.Queue; import java.util.Random; public class CrimeWave { Random r = new Random(); // create the priority queue for the crimes here. Call it crimes. Integer committed = 0; Integer solved = 0; public void meanStreets() { Detective d1 = new Detective("d1"); Detective d2 = new Detective("d2"); Criminal c1 = new Criminal("c1"); Criminal c2 = new Criminal("c2"); // create and start the threads (one per criminal and one per detective) here // write a while loop here that continues as long as at least one of the criminals is // still in business (ie, which the thread for the criminal is not terminated). If the // loop condition is true, use Thread.sleep to wait 200 milliseconds before checking again System.out.println("committed: " + committed); System.out.println("solved: " + solved); } public synchronized void commitCrime(Crime c) { // write one line of code to add the crime to crimes committed++; } public synchronized void solveCrimes(String name) { try { while (crimes.isEmpty()) Thread.sleep(25); // write code here to remove the next crime from the priority queu crimes and set an int called s to the crime's // seriousness level solved++; System.out.println(name + " solves a crime of seriousness " + s); } catch (Exception e) { e.printStackTrace(); } } public static void main(String[] args) { CrimeWave c = new CrimeWave(); c.meanStreets(); System.exit(0); } private class Crime implements Comparable { // finish writing Crime here. Use Random.nextInt(10) to get the random level of seriousness. } private class Criminal implements Runnable { String name; private Criminal(String nameIn) { name = nameIn; } @Override public void run() { for (int i = 0; i < 50; i++) { try { Thread.sleep(r.nextInt(100)); } catch (InterruptedException e) { e.printStackTrace(); } int s = (r.nextInt(5)); Crime c = new Crime(s); System.out.println(name + " commits a crime of seriousness " + s); commitCrime(c); } } } private class Detective implements Runnable { // finish writing Detective here } }
In Java, Consider a crime wave during two criminals simultaneously commit crimes while two detectives simultaneously
solve the crimes. A crime has an integer seriousness level between 0 (creating a public nuisance) and 4 (murder).
Criminals commit crimes with random seriousness and wait a random amount of time between 0 and 100
milliseconds between crimes. Each criminal commits 50 crimes and then retires to live from the loot. Detectives
solve the most serious crimes first, resting for 60 milliseconds after solving each crime. The application continues
running until both the criminals have retired (ie, the two threads containing the criminal Runnables have
terminated) and all the crimes have been solved. Here are the first few lines of output from a sample run of the
program:
c1 commits a crime of seriousness 1
d1 solves a crime of seriousness 1
c1 commits a crime of seriousness 4
d2 solves a crime of seriousness 4
c2 commits a crime of seriousness 0
c2 commits a crime of seriousness 0
Here are the last few lines of output from a typical run:
d1 solves a crime of seriousness 0
d2 solves a crime of seriousness 0
committed: 100
solved: 100
Complete the missing code:
package crime;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Random;
public class CrimeWave {
Random r = new Random();
// create the priority queue for the crimes here. Call it crimes.
Integer committed = 0;
Integer solved = 0;
public void meanStreets() {
Detective d1 = new Detective("d1");
Detective d2 = new Detective("d2");
Criminal c1 = new Criminal("c1");
Criminal c2 = new Criminal("c2");
// create and start the threads (one per criminal and one per detective) here
// write a while loop here that continues as long as at least one of the criminals is
// still in business (ie, which the thread for the criminal is not terminated). If the
// loop condition is true, use Thread.sleep to wait 200 milliseconds before checking again
System.out.println("committed: " + committed);
System.out.println("solved: " + solved);
}
public synchronized void commitCrime(Crime c) {
// write one line of code to add the crime to crimes
committed++;
}
public synchronized void solveCrimes(String name) {
try {
while (crimes.isEmpty())
Thread.sleep(25);
// write code here to remove the next crime from the priority queu crimes and set an int called s to the crime's
// seriousness level
solved++;
System.out.println(name + " solves a crime of seriousness " + s);
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
CrimeWave c = new CrimeWave();
c.meanStreets();
System.exit(0);
}
private class Crime implements Comparable<Crime> {
// finish writing Crime here. Use Random.nextInt(10) to get the random level of seriousness.
}
private class Criminal implements Runnable {
String name;
private Criminal(String nameIn) {
name = nameIn;
}
@Override
public void run() {
for (int i = 0; i < 50; i++) {
try {
Thread.sleep(r.nextInt(100));
} catch (InterruptedException e) {
e.printStackTrace();
}
int s = (r.nextInt(5));
Crime c = new Crime(s);
System.out.println(name + " commits a crime of seriousness "
+ s);
commitCrime(c);
}
}
}
private class Detective implements Runnable {
// finish writing Detective here
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

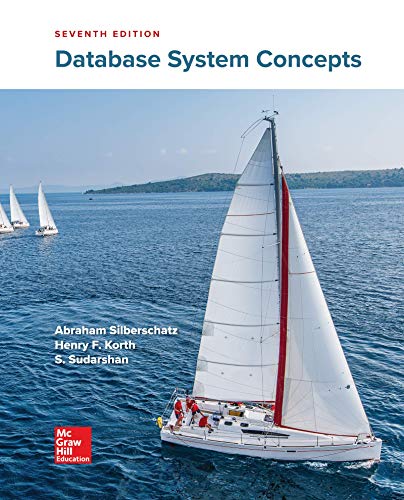
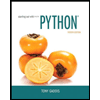
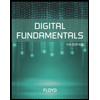
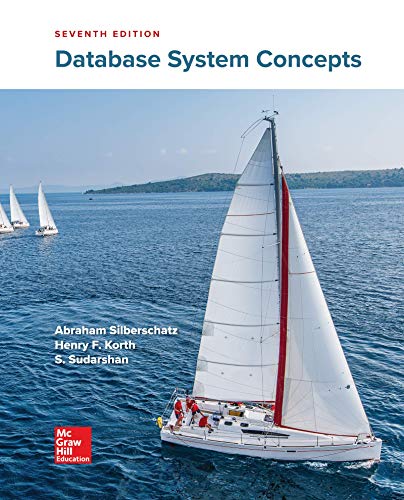
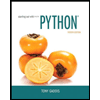
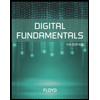
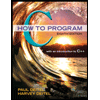
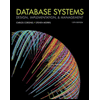
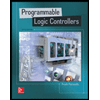