//Movie.java import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructor public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } // overloaded parameterized constructor public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; //numCastMember is set to array length this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() { return this.isKidFriendly; } public int getNumCastMembers() { return this.numCastMembers; } public String[] getCastMembers() { return this.castMembers.clone(); } //Setter methods public void setMovieName(String movieName) { this.movieName = movieName; } public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } public void setIsKidFriendly(boolean isKidFriendly) { this.isKidFriendly = isKidFriendly; } //replace cast memeber at index with new cast memeber //return false if index in invalid //else return true; public boolean replaceCastMember(int index, String castMemberName) { if (index < 0 || index > numCastMembers) { return false; } castMembers[index] = castMemberName; return true; } //compare two arrays //return true if thery are same else return false public boolean doArraysMatch(String[] arr1, String[] arr2) { if (arr1 == null && arr2 == null) { return true; } //here is the change you were wrote arr1.length != arr2.length if (arr1.length == arr2.length) { for (int i = 0; i < arr1.length; i++) { //to ignore case compare lowercase version of both stirng //here is the change. If default constructor get called then array length is 10 but there is no any casting available if (arr1[i] != null && arr2[i] != null) { if (arr1[i].toLowerCase().equals(arr2[i].toLowerCase()) == false) { return false; } } } return true; } return false; } //return comma separated name of castmembers public String getCastMemberNamesAsString() { if (numCastMembers == 0) { return "none"; } String names = castMembers[0]; for (int i = 1; i < numCastMembers; i++) { names += ", " + castMembers[i]; } return names; } public String toString() { String friendly; if (isKidFriendly()) { friendly = "kid friendly"; } else { friendly = "not kid freindly"; } return "Movie:" + " [ Minutes " + String.format("%03d" , getNumMinutes())+ " " + "| Movie Name: " + getMovieName() + " " + "| " + friendly + " " + "| Number of Cast Members: " + getNumCastMembers() + " " + "| Cast Members: " + getCastMemberNamesAsString() + " " + "]"; //"Movie: [ Minutes 142 | Movie Name: The Shawshank Redemption | not kid friendly | Number of Cast Members: 3 | Cast Members: Tim Robbins, Morgan Freeman, Bob Gunton ] } //compare two movie object //return true if every member variable matches else return false public boolean equals(Object o) { Movie m = (Movie) o; return movieName.equals(m.getMovieName()) && numMinutes == m.getNumMinutes() && doArraysMatch(castMembers, m.getCastMembers()); } //tester method to check the program public static void main(String[] args) { Movie movie = new Movie("The Shawshank Redemption", 142, false, new String[]{"Tim Robbins", "Morgan Freeman", "Bob Gunton"}); Movie movie1 = new Movie(" Aladin", 90, true, new String[]{"Scott Weigner", "Robin Williams", "Linda Larkin", "Jonathan Freeman"}); System.out.println(movie.toString()); System.out.println(movie1.toString()); //replaceCastMember movie.replaceCastMember(0, "Tim Southee"); System.out.println("After Replacement:"); System.out.println(movie.toString()); //compare two movie object System.out.println("Compare the two objects:"); System.out.println("equals:" + movie.equals(movie1)); } }
//Movie.java
import java.util.Arrays;
public class Movie {
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
// default constructor
public Movie() {
movieName = "Flick";
numMinutes = 0;
isKidFriendly = false;
numCastMembers = 0;
castMembers = new String[10];
}
// overloaded parameterized constructor
public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) {
this.movieName = movieName;
this.numMinutes = numMinutes;
this.isKidFriendly = isKidFriendly;
//numCastMember is set to array length
this.numCastMembers = castMembers.length;
this.castMembers = castMembers;
}
public String getMovieName() {
return this.movieName;
}
public int getNumMinutes() {
return this.numMinutes;
}
public boolean getIsKidFriendly() {
return this.isKidFriendly;
}
public boolean isKidFriendly() {
return this.isKidFriendly;
}
public int getNumCastMembers() {
return this.numCastMembers;
}
public String[] getCastMembers() {
return this.castMembers.clone();
}
//Setter methods
public void setMovieName(String movieName) {
this.movieName = movieName;
}
public void setNumMinutes(int numMinutes) {
this.numMinutes = numMinutes;
}
public void setIsKidFriendly(boolean isKidFriendly) {
this.isKidFriendly = isKidFriendly;
}
//replace cast memeber at index with new cast memeber
//return false if index in invalid
//else return true;
public boolean replaceCastMember(int index, String castMemberName) {
if (index < 0 || index > numCastMembers) {
return false;
}
castMembers[index] = castMemberName;
return true;
}
//compare two arrays
//return true if thery are same else return false
public boolean doArraysMatch(String[] arr1, String[] arr2) {
if (arr1 == null && arr2 == null) {
return true;
}
//here is the change you were wrote arr1.length != arr2.length
if (arr1.length == arr2.length) {
for (int i = 0; i < arr1.length; i++) {
//to ignore case compare lowercase version of both stirng
//here is the change. If default constructor get called then array length is 10 but there is no any casting available
if (arr1[i] != null && arr2[i] != null) {
if (arr1[i].toLowerCase().equals(arr2[i].toLowerCase()) == false) {
return false;
}
}
}
return true;
}
return false;
}
//return comma separated name of castmembers
public String getCastMemberNamesAsString() {
if (numCastMembers == 0) {
return "none";
}
String names = castMembers[0];
for (int i = 1; i < numCastMembers; i++) {
names += ", " + castMembers[i];
}
return names;
}
public String toString() {
String friendly;
if (isKidFriendly()) {
friendly = "kid friendly";
} else {
friendly = "not kid freindly";
}
return "Movie:"
+ " [ Minutes " + String.format("%03d" , getNumMinutes())+ " "
+ "| Movie Name: " + getMovieName() + " "
+ "| " + friendly + " "
+ "| Number of Cast Members: " + getNumCastMembers() + " "
+ "| Cast Members: " + getCastMemberNamesAsString() + " "
+ "]";
//"Movie: [ Minutes 142 | Movie Name: The Shawshank Redemption | not kid friendly | Number of Cast Members: 3 | Cast Members: Tim Robbins, Morgan Freeman, Bob Gunton ]
}
//compare two movie object
//return true if every member variable matches else return false
public boolean equals(Object o) {
Movie m = (Movie) o;
return movieName.equals(m.getMovieName())
&& numMinutes == m.getNumMinutes()
&& doArraysMatch(castMembers, m.getCastMembers());
}
//tester method to check the program
public static void main(String[] args) {
Movie movie = new Movie("The Shawshank Redemption", 142, false, new String[]{"Tim Robbins", "Morgan Freeman", "Bob Gunton"});
Movie movie1 = new Movie(" Aladin", 90, true, new String[]{"Scott Weigner", "Robin Williams", "Linda Larkin", "Jonathan Freeman"});
System.out.println(movie.toString());
System.out.println(movie1.toString());
//replaceCastMember
movie.replaceCastMember(0, "Tim Southee");
System.out.println("After Replacement:");
System.out.println(movie.toString());
//compare two movie object
System.out.println("Compare the two objects:");
System.out.println("equals:" + movie.equals(movie1));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

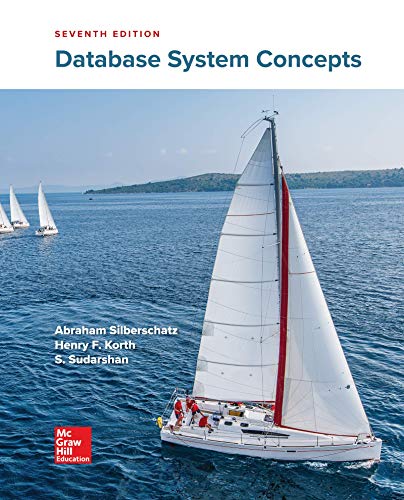
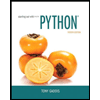
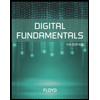
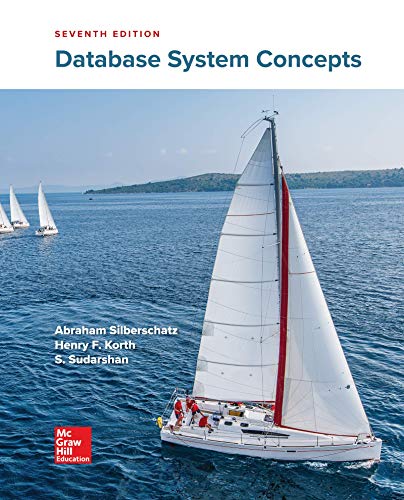
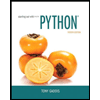
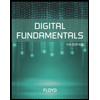
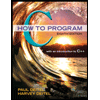
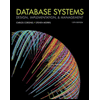
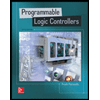