LocalResource(String date, String sector)- Actions of the constructor include accepting a date in the format “dd/mm/yyyy”, initializing the base class, storing the sector and storing an id as a consecutively increasing integer. Note that the constructor must ensure that the date of birth information is recorded. b. getId():Integer - returns the ID of the current instance of LocalResource c. getSector():String – returns the sector associated with the current instance of localResource d. getTRN():String – returns the trn number of the current instance of LocalResource. The process used to determine the TRN is to add the id to the number 100000000 and then returning the string equivalent. e. Update the NineToFiver class to ensure it properly extends the LocalResource class f. In method getContact() of NineToFiver, remove the comments so that the method returns "Local Employee #"+and the id of the contact. Write a concrete class LocalConsultant that extends LocalResource, and implements Citizen and Consultant. LocalConsultant exposes the following public methods: a. LocalConsultant(String dob, String sector, double skillPrice, double taxRate) Saves local instance data – populates superclass data and calculates and saves a permit tax for the instance with a value given by taxRate*skillPrice. b. earnFromSkill():double- return the skillPrice for the instance c. getContact():String – return the string value obtained by joining the text “LocalConsultant#” with the id associated with the person d. getPay():double – returns the value obtained by subtracting the permit tax from the value earned from the skill. Attempted code: class LocalResource extends Person { private static int idCounter = 0; private int id; private String sector; private String trn; // Constructor for LocalResource class // Accepts a date in the format "dd/mm/yyyy", initializes the base class, // stores the sector, and stores an ID as a consecutively increasing integer public LocalResource(String date, String sector) { super(); // Call the default constructor of the Person class for reference String[] parts = date.split("/"); super.setDob(Integer.parseInt(parts[0]), Integer.parseInt(parts[1]), Integer.parseInt(parts[2])); this.sector = sector; this.id = ++idCounter; this.trn = getTRN(); } // Returns the sector associated with the current instance of LocalResource public String getSector() { return sector; } // Returns the ID of the current instance of LocalResource public int getId() { return id; } public String getTRN() { return String.valueOf(id + 100000000); } // Returns a string representation of the LocalResource object // in the format "[dd]LocalResource#id to be paid $pay in the sector sector" public String toString() { NumberFormat formatter = NumberFormat.getCurrencyInstance(); return "[" + super.getDobDay() + "]" + getContact() + " to be paid " + formatter.format(getPay()) + " in the " + sector + " sector"; } // Abstract method from the Person class that must be implemented // Returns the pay for the current instance of LocalResource @Override public double getPay() { return 0; } @Override public String getContact() { return "LocalResource#" + id; } } class LocalConsultant extends LocalResource implements Citizen, Consultant { private double skillPrice; private double permitTax; public LocalConsultant(String dob, String sector, double skillPrice, double taxRate) { super(sector, dob); this.skillPrice = skillPrice; this.permitTax = taxRate * skillPrice; } public double earnFromSkill() { return skillPrice; } public String getContact() { return "LocalConsultant#" + getId(); } public double getPay() { return earnFromSkill() - permitTax; } }
LocalResource(String date, String sector)- Actions of the constructor include accepting a date in the format “dd/mm/yyyy”, initializing the base class, storing the sector and storing an id as a consecutively increasing integer. Note that the constructor must ensure that the date of birth information is recorded. b. getId():Integer - returns the ID of the current instance of LocalResource c. getSector():String – returns the sector associated with the current instance of localResource d. getTRN():String – returns the trn number of the current instance of LocalResource. The process used to determine the TRN is to add the id to the number 100000000 and then returning the string equivalent. e. Update the NineToFiver class to ensure it properly extends the LocalResource class f. In method getContact() of NineToFiver, remove the comments so that the method returns "Local Employee #"+and the id of the contact.
-
Write a concrete class LocalConsultant that extends LocalResource, and implements Citizen and Consultant. LocalConsultant exposes the following public methods: a. LocalConsultant(String dob, String sector, double skillPrice, double taxRate)
- Saves local instance data – populates superclass data and calculates and saves a permit tax for the instance with a value given by taxRate*skillPrice. b. earnFromSkill():double- return the skillPrice for the instance c. getContact():String – return the string value obtained by joining the text “LocalConsultant#” with the id associated with the person d. getPay():double – returns the value obtained by subtracting the permit tax from the value earned from the skill.
- Attempted code:
-
class LocalResource extends Person {
private static int idCounter = 0;
private int id;
private String sector;
private String trn;// Constructor for LocalResource class
// Accepts a date in the format "dd/mm/yyyy", initializes the base class,
// stores the sector, and stores an ID as a consecutively increasing integer
public LocalResource(String date, String sector) {
super(); // Call the default constructor of the Person class for reference
String[] parts = date.split("/");
super.setDob(Integer.parseInt(parts[0]), Integer.parseInt(parts[1]), Integer.parseInt(parts[2]));
this.sector = sector;
this.id = ++idCounter;
this.trn = getTRN();
}// Returns the sector associated with the current instance of LocalResource
public String getSector() {
return sector;
}// Returns the ID of the current instance of LocalResource
public int getId() {
return id;
}public String getTRN() {
return String.valueOf(id + 100000000);
}// Returns a string representation of the LocalResource object
// in the format "[dd]LocalResource#id to be paid $pay in the sector sector"
public String toString() {
NumberFormat formatter = NumberFormat.getCurrencyInstance();
return "[" + super.getDobDay() + "]" + getContact() + " to be paid " +
formatter.format(getPay()) + " in the " + sector + " sector";
}// Abstract method from the Person class that must be implemented
// Returns the pay for the current instance of LocalResource
@Override
public double getPay() {
return 0;
}
@Override
public String getContact() {
return "LocalResource#" + id;
}
}class LocalConsultant extends LocalResource implements Citizen, Consultant {
private double skillPrice;
private double permitTax;
public LocalConsultant(String dob, String sector, double skillPrice, double taxRate)
{
super(sector, dob);
this.skillPrice = skillPrice;
this.permitTax = taxRate * skillPrice;
}
public double earnFromSkill()
{
return skillPrice;
}
public String getContact()
{
return "LocalConsultant#" + getId();
}
public double getPay()
{
return earnFromSkill() - permitTax;
}
}



Step by step
Solved in 2 steps

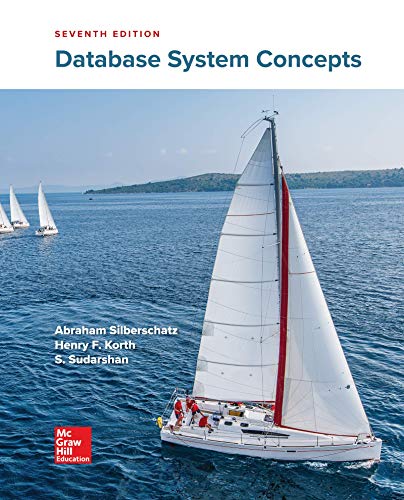
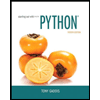
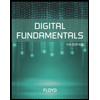
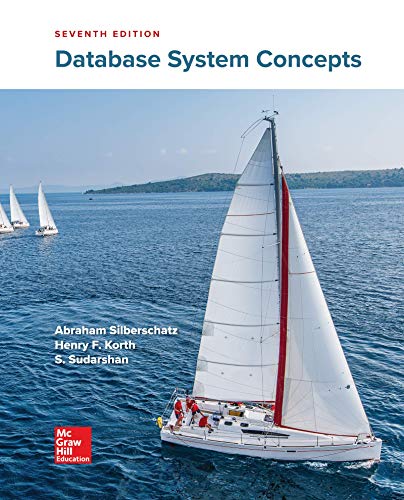
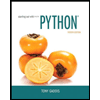
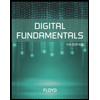
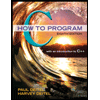
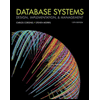
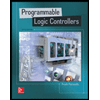