Last lab, we modified the inventory program to write a file. This lab, we’ll modify it to read a file. First, take your program from the last lab. Add one more feature: at the end, just before you close the file, write the word “END” all by itself on a line. Run that program once to create a file of items with “END”. Now, copy that program to a new file cp lab7.cpp lab8.cpp so you can use it as a basis for this lab. Modify lab8 so instead of reading from cin and writing to a file, you read from your file and write to cout. The only tricky part is deciding when to stop reading. In lab7, you stopped reading when the user said they didn’t want to continue. For lab8, keep reading until you read “END” for the name of the produce. You can use “==” on strings, so if (product name == ”END”) will tell you that you can stop reading. PHOTO OF WHAT IT SHOULD LOOK LIKE INCLUDED My code for last lab: #include #include #include using namespace std; int main () { ofstream w; int c=1; string filename; cout << "File Name: "; getline(cin, filename); w.open(filename.c_str()); while(c==1){ string product_name; cout << "Product name: "; getline(cin, product_name,'\n'); w << product_name << endl; double wppi; //wholesale price per item cout << "Wholesale price: "; cin >> wppi; w << "Wholesale Price: " << wppi << " "; int quanity; cout << "Quanity: "; cin >> quanity; w << "Quanity: " << quanity << " "; double total_price_paid; total_price_paid = wppi * quanity; double markup; cout << "What is the markup? "; cin >> markup; double retail_price; double anticipated_profit; retail_price = wppi + markup; anticipated_profit = total_price_paid - wppi; w << "Retail Price: " << retail_price << " " << "Anticipated Profit: " << anticipated_profit << endl; cout << "Do you want to process another record? Type 1 for Yes or 2 for No." <> c; cin.ignore(); } return 0; }
Last lab, we modified the inventory program to write a file. This lab, we’ll modify it to read a file. First, take your program from the last lab. Add one more feature: at the end, just before you close the file, write the word “END” all by itself on a line. Run that program once to create a file of items with “END”. Now, copy that program to a new file cp lab7.cpp lab8.cpp so you can use it as a basis for this lab. Modify lab8 so instead of reading from cin and writing to a file, you read from your file and write to cout. The only tricky part is deciding when to stop reading. In lab7, you stopped reading when the user said they didn’t want to continue. For lab8, keep reading until you read “END” for the name of the produce. You can use “==” on strings, so if (product name == ”END”) will tell you that you can stop reading.
PHOTO OF WHAT IT SHOULD LOOK LIKE INCLUDED
My code for last lab:
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
int main ()
{
ofstream w;
int c=1;
string filename;
cout << "File Name: ";
getline(cin, filename);
w.open(filename.c_str());
while(c==1){
string product_name;
cout << "Product name: ";
getline(cin, product_name,'\n');
w << product_name << endl;
double wppi; //wholesale price per item
cout << "Wholesale price: ";
cin >> wppi;
w << "Wholesale Price: " << wppi << " ";
int quanity;
cout << "Quanity: ";
cin >> quanity;
w << "Quanity: " << quanity << " ";
double total_price_paid;
total_price_paid = wppi * quanity;
double markup;
cout << "What is the markup? ";
cin >> markup;
double retail_price;
double anticipated_profit;
retail_price = wppi + markup;
anticipated_profit = total_price_paid - wppi;
w << "Retail Price: " << retail_price << " " << "Anticipated Profit: " << anticipated_profit << endl;
cout << "Do you want to process another record? Type 1 for Yes or 2 for No." <<endl;
cin >> c;
cin.ignore();
}
return 0;
}
![[mingliênolaris:~/TA]$ more 8.txt
big apple
10 10 10 l0 10
yellow oringe
20 20 20 20 20
END
aaa bbb
30 30 30 30 30
[mingli@polaris:~/TA]$ ./lab8
big apple
10 10 10 10 10
yellow oringe
20 20 20 20 20
END
data in file
output](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5bd3697d-7244-4dc8-845a-c06954fa71b0%2F63168538-12d7-4c46-a480-0a28f43f2e10%2F6vg1zhm_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

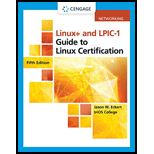
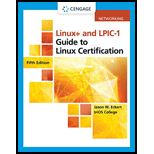