Java this piece of my code is not working .....I am trying to add Warship with a type or warship , but I keep getting an error ? //add another WarShip instance to the ArrayList. //use a foreach loop to process the ArrayList and print the data for each ship. //count the number of ships that are not afloat. Return this count to main for printing. //In the shipShow method: the number of ships that are still a Float public static int shipShow(ArrayList fleetList) { //from the ArrayList, remove the CargoShip that was declared as type Ship. fleetList.remove(2); //Removing ElFaro form the list // review Type casting instanceof | up casting Ship < Warship , subclass is a object of its parents //add warship USS John Warner - add cast by (Ship) new WarShip Ship warship2 = (Ship) new WarShip("USS John Warner", 2015, true, "attack submarine", "United States"); fleetList.add(warship2); int count= 0; //use a foreach loop to process the ArrayList and print the data for each ship. for(Ship temp:fleetList) { //count the number of ships that are not afloat. Return this count to main for printing. if(temp.isAFloat()==false) { count++; //incrementing count if the ship is not aFloat } System.out.println(temp+"\n"); //displaying ship data } return count; //returning number of sinking ships } } Full ShipTest Code import java.util.ArrayList; import java.util.Arrays; public class ShipTest { public static void main(String[] args) { //make two objects with declared type Ship but actual type CruiseShip. Ship cruiseship1 = new CruiseShip("Magic",1998, true, 2700,"Caribbean"); // Super Class = ship SubClass = Cruiseship: Example of Up casting Ship cruiseship2 = new CruiseShip ("Titanic",1912, false, 1300, "Atlanic Ocean"); //make one object of declared type Ship but actual type CargoShip. Ship cargoship1 = new CargoShip("El faro",1974,false,"cargo", 391); //make one object of declared type CargoShip and actual type CargoShip. //casting needs to apply here because of the type Subtype = CargoShip | CargoShip CargoShip cargoship2 = new CargoShip ("Seawise Giant",1979,false,"crude oil", 564763); ///make a ship of declared type WarShip and actual type WarShip. Line 51 in BOLD , How do I add a Warship of Type Warship to my arraylist and Print this ? WarShip warship1 = new WarShip("USS Nimitz",1972,true,"suppcarrier ", "Unites States Navy"); //casting here //up casting is automatic // down casting is done manually //downcast the warship class by coding (Ship)cargo|warship to point the warship to the ship class // Array of type Ship named fleet = all the ships = 5 ships total ") Ship[]fleet = {cruiseship1,cruiseship2,cargoship1,cargoship2,(Ship) warship1}; //code a for loop that processes the fleet array to show display all ships and their data. for (Ship temp:fleet) { System.out.println(temp);} //use a method of class Arrays to create an ArrayList of type Ship from the fleet array. //asList(fleet) class returns a list passed to the ArrayList construtor for creating an Array object ArrayListfleetlist = new ArrayList(Arrays.asList(fleet)); //pass this ArrayList to a method named shipShow that also displays all ships and returns an integer //report the number of ships can sank System.out.println(shipShow(fleetlist)+" of these ships sank!"); } //In the shipShow method: //add another WarShip instance to the ArrayList. //use a foreach loop to process the ArrayList and print the data for each ship. //count the number of ships that are not afloat. Return this count to main for printing. //In the shipShow method: the number of ships that are still a Float public static int shipShow(ArrayList fleetList) { //from the ArrayList, remove the CargoShip that was declared as type Ship. fleetList.remove(2); //Removing ElFaro form the list // review Type casting instanceof | up casting Ship < Warship , subclass is a object of its parents //add warship USS John Warner - add cast by (Ship) new WarShip Ship warship2 = (Ship) new WarShip("USS John Warner", 2015, true, "attack submarine", "United States"); fleetList.add(warship2); int count= 0;
Java this piece of my code is not working .....I am trying to add Warship with a type or warship , but I keep getting an error ?
//add another WarShip instance to the ArrayList.
//use a foreach loop to process the ArrayList and print the data for each ship.
//count the number of ships that are not afloat. Return this count to main for printing.
//In the shipShow method: the number of ships that are still a Float
public static int shipShow(ArrayList<Ship> fleetList) {
//from the ArrayList, remove the CargoShip that was declared as type Ship.
fleetList.remove(2); //Removing ElFaro form the list
// review Type casting instanceof | up casting Ship < Warship , subclass is a object of its parents
//add warship USS John Warner - add cast by (Ship) new WarShip
Ship warship2 = (Ship) new WarShip("USS John Warner", 2015, true, "attack submarine", "United States");
fleetList.add(warship2);
int count= 0;
//use a foreach loop to process the ArrayList and print the data for each ship.
for(Ship temp:fleetList) {
//count the number of ships that are not afloat. Return this count to main for printing.
if(temp.isAFloat()==false) {
count++; //incrementing count if the ship is not aFloat
}
System.out.println(temp+"\n"); //displaying ship data
}
return count; //returning number of sinking ships
}
}
Full ShipTest Code
import java.util.ArrayList;
import java.util.Arrays;
public class ShipTest {
public static void main(String[] args) {
//make two objects with declared type Ship but actual type CruiseShip.
Ship cruiseship1 = new CruiseShip("Magic",1998, true, 2700,"Caribbean");
// Super Class = ship SubClass = Cruiseship: Example of Up casting
Ship cruiseship2 = new CruiseShip ("Titanic",1912, false, 1300, "Atlanic Ocean");
//make one object of declared type Ship but actual type CargoShip.
Ship cargoship1 = new CargoShip("El faro",1974,false,"cargo", 391);
//make one object of declared type CargoShip and actual type CargoShip.
//casting needs to apply here because of the type Subtype = CargoShip | CargoShip
CargoShip cargoship2 = new CargoShip ("Seawise Giant",1979,false,"crude oil", 564763);
///make a ship of declared type WarShip and actual type WarShip.
Line 51 in BOLD , How do I add a Warship of Type Warship to my arraylist and Print this ?
WarShip warship1 = new WarShip("USS Nimitz",1972,true,"suppcarrier ", "Unites States Navy");
//casting here
//up casting is automatic
// down casting is done manually
//downcast the warship class by coding (Ship)cargo|warship to point the warship to the ship class
// Array of type Ship named fleet = all the ships = 5 ships total ")
Ship[]fleet = {cruiseship1,cruiseship2,cargoship1,cargoship2,(Ship) warship1};
//code a for loop that processes the fleet array to show display all ships and their data.
for (Ship temp:fleet) {
System.out.println(temp);}
//use a method of class Arrays to create an ArrayList of type Ship from the fleet array.
//asList(fleet) class returns a list passed to the ArrayList construtor for creating an Array object
ArrayList<Ship>fleetlist = new ArrayList<Ship>(Arrays.asList(fleet));
//pass this ArrayList to a method named shipShow that also displays all ships and returns an integer
//report the number of ships can sank
System.out.println(shipShow(fleetlist)+" of these ships sank!");
}
//In the shipShow method:
//add another WarShip instance to the ArrayList.
//use a foreach loop to process the ArrayList and print the data for each ship.
//count the number of ships that are not afloat. Return this count to main for printing.
//In the shipShow method: the number of ships that are still a Float
public static int shipShow(ArrayList<Ship> fleetList) {
//from the ArrayList, remove the CargoShip that was declared as type Ship.
fleetList.remove(2); //Removing ElFaro form the list
// review Type casting instanceof | up casting Ship < Warship , subclass is a object of its parents
//add warship USS John Warner - add cast by (Ship) new WarShip
Ship warship2 = (Ship) new WarShip("USS John Warner", 2015, true, "attack submarine", "United States");
fleetList.add(warship2);
int count= 0;
//use a foreach loop to process the ArrayList and print the data for each ship.
for(Ship temp:fleetList) {
//count the number of ships that are not afloat. Return this count to main for printing.
if(temp.isAFloat()==false) {
count++; //incrementing count if the ship is not aFloat
}
System.out.println(temp+"\n"); //displaying ship data
}
return count; //returning number of sinking ships
}
}
//report the value of the integer returned by shipShow.
//In the shipShow method:
//from the ArrayList, remove the CargoShip that was declared as type Ship.
//add another WarShip instance to the ArrayList.
//use a foreach loop to process the ArrayList and print the data for each ship.
//count the number of ships that are not afloat. Return this count to main for printing.


Step by step
Solved in 3 steps with 1 images

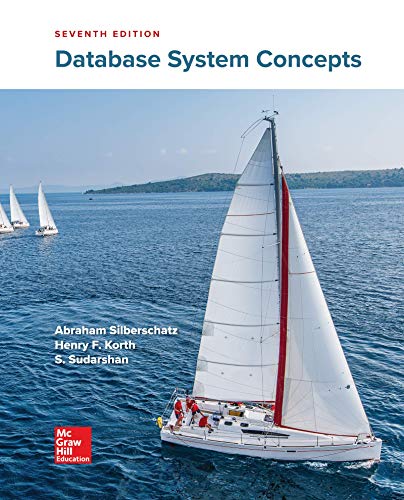
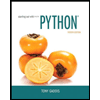
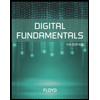
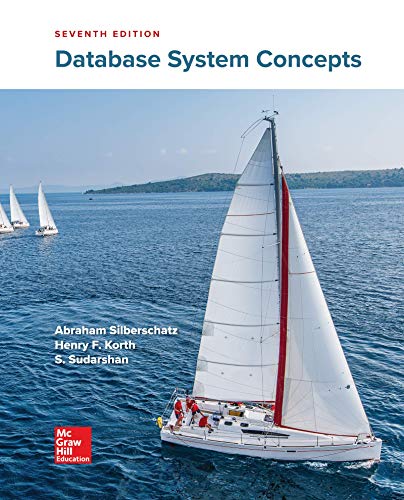
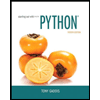
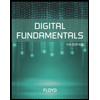
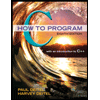
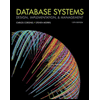
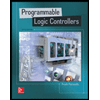