50 // Mark all the vertices as not visited and not part of recursion stack 51 Boolean visited[] = new Boolean [V]; 52 for (int i = 0; i < V; i++) 53 visited[i] = false; 54 55 // Begins iterating through the vertices 56 for (int i = 0; i < V; i++) 57 58 //Doesn't recur if already visited 59 if (!visited[i]) 60 if (isCyclicUtil(i, visited, 1)) 61 return true; 62 return false; 63 } 64 //Driver Method 65 ▼ public static void main(String[] args) { 66 //Graph creation 67 Scanner Edges = new Scanner(System.in); 68 System.out.println("Enter the number of edges."); 69 int edges = Edges.nextInt (); 70 Main g = new Main(edges); 71 ▼ for(int i = 0; i < (edges); i++){ 72 System.out.println("Enter the pair of vertices for this edge:" ); 73 int holding1 Edges.nextInt(); 74 int holding2 = Edges.nextInt(); 75 g.addEdge (holding1, holding2); 76 9} 77 78 } 79 //Cycle check 80 if (g.isCyclic()) 81 System.out.println("Graph contains cycle"); 82 83 System.out.println("Graph doesn't contain cycle"); else 2 import java.io.*; 3 import java.util.*; 4 //Undirected Graph Class 5 class Main { 6 //Number of Vertices 7 private int V; 8 //Adjacency Lists 9 private LinkedList adj[]; 10 //Constructor 11 Main (int v) { 12 V = V; 13 adj = new LinkedList[v]; 14 for (int i = 0; i < v; ++i) 15 adj[i] = new LinkedList(); 16 } 17 18 // Function that adds an edge to the graph 19▼ void addEdge(int v, int w) { 20 adj[v].add(w); 21 adj [w].add(v); 22 } 24 // A recursive cyclicUtil function 25 ▼ Boolean isCyclicUtil(int v, Boolean visited [], int parent) { 26 27 //Marks the current node as visited 28 visited [v] = true; 29 Integer i; 30 31 // Recur for all the vertices adjacent to this vertex 32 Iterator it = adj[v].iterator(); 33 while (it.hasNext()) { 34 iit.next(); 35 36 // If an adjacent is not visited, call for the cyclicutil method 37 if (!visited[i]) { 38 if (isCyclicUtil(i, visited, v)) 39 return true; 40 } 41 // If an adjacent is visited and not parent of current vertex, then there is a cycle 42 else if (i != parent) 43 return true; 44 } 45 return false;
50 // Mark all the vertices as not visited and not part of recursion stack 51 Boolean visited[] = new Boolean [V]; 52 for (int i = 0; i < V; i++) 53 visited[i] = false; 54 55 // Begins iterating through the vertices 56 for (int i = 0; i < V; i++) 57 58 //Doesn't recur if already visited 59 if (!visited[i]) 60 if (isCyclicUtil(i, visited, 1)) 61 return true; 62 return false; 63 } 64 //Driver Method 65 ▼ public static void main(String[] args) { 66 //Graph creation 67 Scanner Edges = new Scanner(System.in); 68 System.out.println("Enter the number of edges."); 69 int edges = Edges.nextInt (); 70 Main g = new Main(edges); 71 ▼ for(int i = 0; i < (edges); i++){ 72 System.out.println("Enter the pair of vertices for this edge:" ); 73 int holding1 Edges.nextInt(); 74 int holding2 = Edges.nextInt(); 75 g.addEdge (holding1, holding2); 76 9} 77 78 } 79 //Cycle check 80 if (g.isCyclic()) 81 System.out.println("Graph contains cycle"); 82 83 System.out.println("Graph doesn't contain cycle"); else 2 import java.io.*; 3 import java.util.*; 4 //Undirected Graph Class 5 class Main { 6 //Number of Vertices 7 private int V; 8 //Adjacency Lists 9 private LinkedList adj[]; 10 //Constructor 11 Main (int v) { 12 V = V; 13 adj = new LinkedList[v]; 14 for (int i = 0; i < v; ++i) 15 adj[i] = new LinkedList(); 16 } 17 18 // Function that adds an edge to the graph 19▼ void addEdge(int v, int w) { 20 adj[v].add(w); 21 adj [w].add(v); 22 } 24 // A recursive cyclicUtil function 25 ▼ Boolean isCyclicUtil(int v, Boolean visited [], int parent) { 26 27 //Marks the current node as visited 28 visited [v] = true; 29 Integer i; 30 31 // Recur for all the vertices adjacent to this vertex 32 Iterator it = adj[v].iterator(); 33 while (it.hasNext()) { 34 iit.next(); 35 36 // If an adjacent is not visited, call for the cyclicutil method 37 if (!visited[i]) { 38 if (isCyclicUtil(i, visited, v)) 39 return true; 40 } 41 // If an adjacent is visited and not parent of current vertex, then there is a cycle 42 else if (i != parent) 43 return true; 44 } 45 return false;
Oh no! Our experts couldn't answer your question.
Don't worry! We won't leave you hanging. Plus, we're giving you back one question for the inconvenience.
Submit your question and receive a step-by-step explanation from our experts in as fast as 30 minutes.
You have no more questions left.
Message from our expert:
Our experts are unable to provide you with a solution at this time. Try rewording your question, and make sure to submit one question at a time. We've credited a question to your account.
Your Question:
Java Program.
The directions for this program are as follows - write a java program of a data file structure for a connected graph that produces a tree. There is a cycle in a graph only if there is a back edge present in the graph. To find the back edge to any of its ancestor keep a visited array and if there is a back edge to any visited node then there is a loop and return true.
The photos provided show what I coded so far, however, I want any errors (if present) in the code to be fixed, and I want there to be a visual graph or tree outputted after the program asks the user to enter number of edges and vertices. Please help, as I don't know how to output a visual graph/tree that shows the values of the user's input.
![50 // Mark all the vertices as not visited and not part of recursion stack
51 Boolean visited[] = new Boolean [V];
52 for (int i = 0; i < V; i++)
53 visited[i] = false;
54
55
// Begins iterating through the vertices
56 for (int i = 0; i < V; i++)
57
58 //Doesn't recur if already visited
59 if (!visited[i])
60 if (isCyclicUtil(i, visited, 1))
61 return true;
62 return false;
63 }
64 //Driver Method
65 ▼ public static void main(String[] args) {
66 //Graph creation
67 Scanner Edges = new Scanner(System.in);
68 System.out.println("Enter the number of edges.");
69 int edges = Edges.nextInt ();
70 Main g = new Main(edges);
71 ▼ for(int i = 0; i < (edges); i++){
72 System.out.println("Enter the pair of vertices for this edge:" );
73 int holding1 Edges.nextInt();
74 int holding2 = Edges.nextInt();
75 g.addEdge (holding1, holding2);
76 9}
77
78 }
79 //Cycle check
80 if (g.isCyclic())
81 System.out.println("Graph contains cycle");
82
83 System.out.println("Graph doesn't contain cycle");
else](https://content.bartleby.com/qna-images/question/4cd5838b-3f34-487e-9642-7770197fe05c/2fc7ab5c-e6ba-4fd4-bc7d-9c1772719683/wn2lxd_thumbnail.png)
Transcribed Image Text:50 // Mark all the vertices as not visited and not part of recursion stack
51 Boolean visited[] = new Boolean [V];
52 for (int i = 0; i < V; i++)
53 visited[i] = false;
54
55
// Begins iterating through the vertices
56 for (int i = 0; i < V; i++)
57
58 //Doesn't recur if already visited
59 if (!visited[i])
60 if (isCyclicUtil(i, visited, 1))
61 return true;
62 return false;
63 }
64 //Driver Method
65 ▼ public static void main(String[] args) {
66 //Graph creation
67 Scanner Edges = new Scanner(System.in);
68 System.out.println("Enter the number of edges.");
69 int edges = Edges.nextInt ();
70 Main g = new Main(edges);
71 ▼ for(int i = 0; i < (edges); i++){
72 System.out.println("Enter the pair of vertices for this edge:" );
73 int holding1 Edges.nextInt();
74 int holding2 = Edges.nextInt();
75 g.addEdge (holding1, holding2);
76 9}
77
78 }
79 //Cycle check
80 if (g.isCyclic())
81 System.out.println("Graph contains cycle");
82
83 System.out.println("Graph doesn't contain cycle");
else
![2 import java.io.*;
3 import java.util.*;
4 //Undirected Graph Class
5 class Main {
6 //Number of Vertices
7 private int V;
8 //Adjacency Lists
9 private LinkedList<Integer> adj[];
10 //Constructor
11 Main (int v) {
12
V = V;
13 adj = new LinkedList[v];
14 for (int i = 0; i < v; ++i)
15 adj[i] = new LinkedList();
16 }
17
18 // Function that adds an edge to the graph
19▼ void addEdge(int v, int w) {
20 adj[v].add(w);
21 adj [w].add(v);
22 }
24 // A recursive cyclicUtil function
25 ▼ Boolean isCyclicUtil(int v, Boolean visited [], int parent) {
26
27 //Marks the current node as visited
28 visited [v] = true;
29 Integer i;
30
31 // Recur for all the vertices adjacent to this vertex
32
Iterator<Integer> it = adj[v].iterator();
33 while (it.hasNext()) {
34 iit.next();
35
36 // If an adjacent is not visited, call for the cyclicutil method
37 if (!visited[i]) {
38 if (isCyclicUtil(i, visited, v))
39 return true;
40 }
41 // If an adjacent is visited and not parent of current vertex, then there is a cycle
42 else if (i != parent)
43
return true;
44 }
45 return false;](https://content.bartleby.com/qna-images/question/4cd5838b-3f34-487e-9642-7770197fe05c/2fc7ab5c-e6ba-4fd4-bc7d-9c1772719683/4x1sjxf_thumbnail.png)
Transcribed Image Text:2 import java.io.*;
3 import java.util.*;
4 //Undirected Graph Class
5 class Main {
6 //Number of Vertices
7 private int V;
8 //Adjacency Lists
9 private LinkedList<Integer> adj[];
10 //Constructor
11 Main (int v) {
12
V = V;
13 adj = new LinkedList[v];
14 for (int i = 0; i < v; ++i)
15 adj[i] = new LinkedList();
16 }
17
18 // Function that adds an edge to the graph
19▼ void addEdge(int v, int w) {
20 adj[v].add(w);
21 adj [w].add(v);
22 }
24 // A recursive cyclicUtil function
25 ▼ Boolean isCyclicUtil(int v, Boolean visited [], int parent) {
26
27 //Marks the current node as visited
28 visited [v] = true;
29 Integer i;
30
31 // Recur for all the vertices adjacent to this vertex
32
Iterator<Integer> it = adj[v].iterator();
33 while (it.hasNext()) {
34 iit.next();
35
36 // If an adjacent is not visited, call for the cyclicutil method
37 if (!visited[i]) {
38 if (isCyclicUtil(i, visited, v))
39 return true;
40 }
41 // If an adjacent is visited and not parent of current vertex, then there is a cycle
42 else if (i != parent)
43
return true;
44 }
45 return false;
Recommended textbooks for you
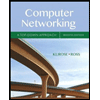
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
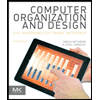
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
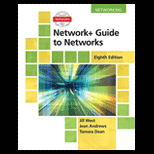
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
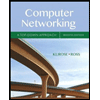
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
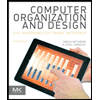
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
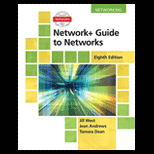
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
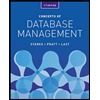
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
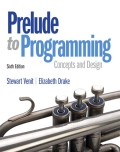
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
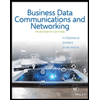
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY