Is the UML diagram I made for this code correct? I was not sure if I needed to add the objects, and not really sure the format they should be in if needed. Guest guest1 = new Guest (" *** ", " *** "); nameList.add(guest1); or does it look correct? //-------here is the program I made, UML diagram is attached in images-- import java.util.ArrayList; public class GuestList { public static void main(String[]args) { ArrayList nameList = new ArrayList (); //Guest objects with names Guest guest1 = new Guest("John", "Doe"); Guest guest2 = new Guest("Mary", "Smith"); Guest guest3 = new Guest("Jean", "Peterson"); Guest guest4 = new Guest("Sean", "Bott"); Guest guest5 = new Guest("Anna", "Hall"); //adds Guest Objects to the nameList array nameList.add(guest1); nameList.add(guest2); nameList.add(guest3); nameList.add(guest4); nameList.add(guest5); // Call the displayGuests(). System.out.println("Here is the list of guests."); displayGuests(nameList); System.out.println(); // for readability purposes // Update the list. // Add 2 more guests. System.out.println("Adding Adriana Lima to the guest list."); nameList.add(new Guest("Adriana","Lima")); System.out.println(); // for readability purposes System.out.println("Adding Robert Shabazz to the guest list."); nameList.add(new Guest("Robert","Shabazz")); System.out.println(); // for readability purposes // Delete the guest who has registered first. System.out.println("Deleting the first guest from the guest list..."); nameList.remove(0); // John Doe will be removed System.out.println(); // for readability purposes // Change the first name of guest 'Mary Smith' to 'Jane'. System.out.println("Changing the first name of guest Mary Smith to Jane."); for (Guest g : nameList) { if (g.getFirstName().equalsIgnoreCase("Mary") && g.getLastName().equalsIgnoreCase("Smith")) { g.setFirstName("Jane"); } } System.out.println(); // for readability purposes // Call the displayGuests(). System.out.println("Here is the modified list of guests."); displayGuests(nameList); } // end main() public static void displayGuests(ArrayList guestList) { for (Guest guest : guestList) { System.out.println(guest.toString()); } } // end displayGuests() }
Is the UML diagram I made for this code correct?
I was not sure if I needed to add the objects, and not really sure the format they should be in if needed.
Guest guest1 = new Guest (" *** ", " *** ");
nameList.add(guest1);
or does it look correct?
//-------here is the program I made, UML diagram is attached in images--
import java.util.ArrayList;
public class GuestList {
public static void main(String[]args) {
ArrayList <Guest> nameList = new ArrayList <Guest>();
//Guest objects with names
Guest guest1 = new Guest("John", "Doe");
Guest guest2 = new Guest("Mary", "Smith");
Guest guest3 = new Guest("Jean", "Peterson");
Guest guest4 = new Guest("Sean", "Bott");
Guest guest5 = new Guest("Anna", "Hall");
//adds Guest Objects to the nameList array
nameList.add(guest1);
nameList.add(guest2);
nameList.add(guest3);
nameList.add(guest4);
nameList.add(guest5);
// Call the displayGuests().
System.out.println("Here is the list of guests.");
displayGuests(nameList);
System.out.println(); // for readability purposes
// Update the list.
// Add 2 more guests.
System.out.println("Adding Adriana Lima to the guest list.");
nameList.add(new Guest("Adriana","Lima"));
System.out.println(); // for readability purposes
System.out.println("Adding Robert Shabazz to the guest list.");
nameList.add(new Guest("Robert","Shabazz"));
System.out.println(); // for readability purposes
// Delete the guest who has registered first.
System.out.println("Deleting the first guest from the guest list...");
nameList.remove(0); // John Doe will be removed
System.out.println(); // for readability purposes
// Change the first name of guest 'Mary Smith' to 'Jane'.
System.out.println("Changing the first name of guest Mary Smith to Jane.");
for (Guest g : nameList) {
if (g.getFirstName().equalsIgnoreCase("Mary") &&
g.getLastName().equalsIgnoreCase("Smith")) {
g.setFirstName("Jane");
}
}
System.out.println(); // for readability purposes
// Call the displayGuests().
System.out.println("Here is the modified list of guests.");
displayGuests(nameList);
} // end main()
public static void displayGuests(ArrayList<Guest> guestList) {
for (Guest guest : guestList) {
System.out.println(guest.toString());
}
} // end displayGuests()
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

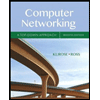
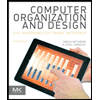
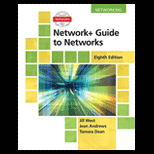
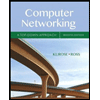
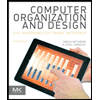
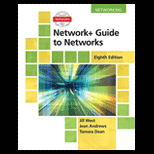
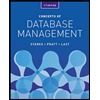
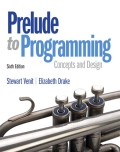
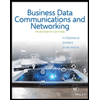