In class HashTable implement a hash table and consider the following: (i) Keys are integers (therefore also negative!) and should be stored in the table int[] data. (ii) As a hash function take h(x) = (x · 701) mod 2000. The size of the table is therefore 2000. Be careful when computing the index of a negative key. For example, the index of the key x = −10 is h(−10) = (−7010) mod 2000 = (2000(−4) + 990) mod 2000 = 990. Hence, indices should be non-negative integers between 0 and 1999! (iii) Implement insert, which takes an integer and inserts it into a table. The method returns true, if the insertion is successful. If an element is already in the table, the function insert should return false. (iv) Implement search, which takes an integer and finds it in the table. The method returns true, if the search is successful and false otherwise. (v) Implement delete, which takes an integer and deletes it form the table. The method returns true, if the deletion is successful and false otherwise. (vi) Collision should be solved by chaining. However, not as usually by linked list, but with a hash table implemented in class HashTable2 (combined structure). The base is given: public class HashTable { HashTable2[] data; public boolean insert(int key) { throw new UnsupportedOperationException("Need to implement this function"); } public boolean search(int key) { throw new UnsupportedOperationException("Need to implement this function"); } public boolean delete(int key) { throw new UnsupportedOperationException("Need to implement this function"); } }
- In class HashTable implement a hash table and consider the following:
(i) Keys are integers (therefore also negative!) and should be stored in the table
int[] data.
(ii) As a hash function take h(x) = (x · 701) mod 2000. The size of the table is
therefore 2000. Be careful when computing the index of a negative key. For
example, the index of the key x = −10 is
h(−10) = (−7010) mod 2000 = (2000(−4) + 990) mod 2000 = 990.
Hence, indices should be non-negative integers between 0 and 1999!
(iii) Implement insert, which takes an integer and inserts it into a table. The
method returns true, if the insertion is successful. If an element is already in
the table, the function insert should return false.
(iv) Implement search, which takes an integer and finds it in the table. The method
returns true, if the search is successful and false otherwise.
(v) Implement delete, which takes an integer and deletes it form the table. The
method returns true, if the deletion is successful and false otherwise.
(vi) Collision should be solved by chaining. However, not as usually by linked list,
but with a hash table implemented in class HashTable2 (combined structure).
The base is given:
HashTable2[] data;
public boolean insert(int key) {
throw new UnsupportedOperationException("Need to implement this function");
}
public boolean search(int key) {
throw new UnsupportedOperationException("Need to implement this function");
}
public boolean delete(int key) {
throw new UnsupportedOperationException("Need to implement this function");
}
}
![-
In class Hash Table implement a hash table and consider the following:
(i) Keys are integers (therefore also negative!) and should be stored in the table
int[] data.
(ii) As a hash function take h(r) = (x - 701) mod 2000. The size of the table is
therefore 2000. Be careful when computing the index of a negative key. For
example, the index of the key x = -10 is
h(-10) = (-7010) mod 2000 = (2000(-4) +990) mod 2000 = 990.
Hence, indices should be non-negative integers between 0 and 1999!
(iii) Implement insert, which takes an integer and inserts it into a table. The
method returns true, if the insertion is successful. If an element is already in
the table, the function insert should return false.
(iv) Implement search, which takes an integer and finds it in the table. The method
returns true, if the search is successful and false otherwise.
(v) Implement delete, which takes an integer and deletes it form the table. The
method returns true, if the deletion is successful and false otherwise.
(vi) Collision should be solved by chaining. However, not as usually by linked list,
but with a hash table implemented in class HashTable2 (combined structure).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffac9c7c8-0bf2-489c-b48d-8061944140b1%2Fc025886b-dfb2-42f4-928f-911fc0c0fef4%2Fc2ognws_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

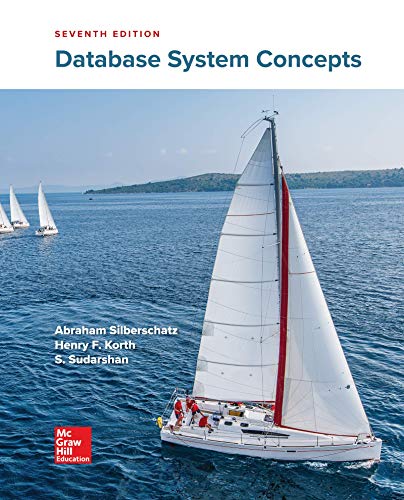
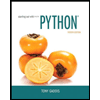
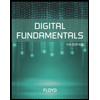
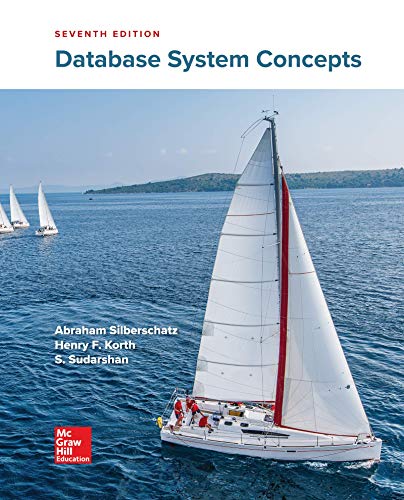
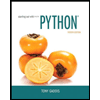
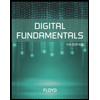
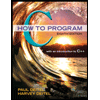
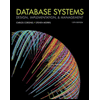
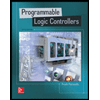