im having some trouble with this Requirements: Write a Python script that prompts the user for the length of a side of a polygon and prints a table of areas of several polygons. Sample output is shown below. Additional requirements: • Your program should use functions to organize the code. Implement these functions: - main The main function should call the function get_side_length and then send side_length to report. - get_side_length This function should prompt the user ("Input the length of a side: ") and return side_length to main as an int. - report This function should print a heading line ("side length...number of sides...area") and use a loop to print a table of values as shown in the sample output below. The report function should call polygon_area to calculate the areas needed for the table. The function report will have to call polygon_area once for each area that it needs to print. - polygon_area This function takes num_sides and side_length as parameters and returns the area of such a polygon. The area of such a polygon is computed by this formula: area = (num_sides * side_length * side_length) \ / (4 * math.tan(math.pi / num_sides))
im having some trouble with this
Requirements:
Write a Python script that prompts the user for the length of a side of a polygon and prints a
table of areas of several polygons. Sample output is shown below.
Additional requirements:
• Your
- main The main function should call the function get_side_length and
then send side_length to report.
- get_side_length This function should prompt the user ("Input the
length of a side: ") and return side_length to main as an int.
- report This function should print a heading line ("side length...number
of sides...area") and use a loop to print a table of values as shown in the
sample output below. The report function should call polygon_area to
calculate the areas needed for the table. The function report will have to call
polygon_area once for each area that it needs to print.
- polygon_area This function takes num_sides and side_length as
parameters and returns the area of such a polygon. The area of such a polygon is
computed by this formula:
area = (num_sides * side_length * side_length) \
/ (4 * math.tan(math.pi / num_sides))



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

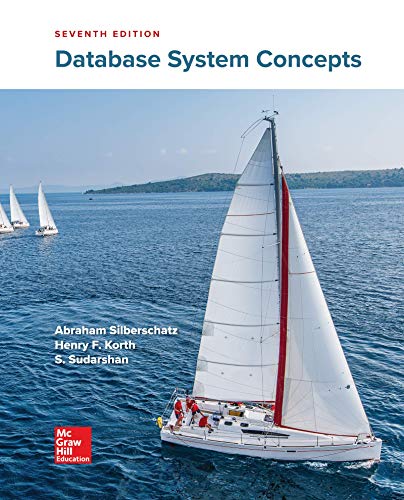
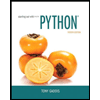
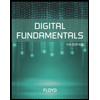
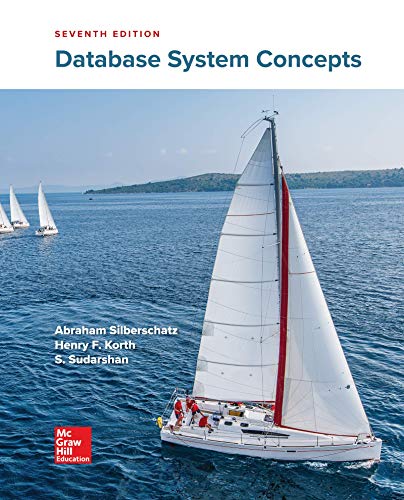
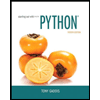
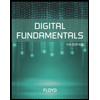
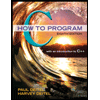
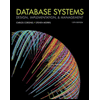
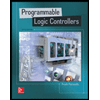