I am learning C++ and would like to make cleaner code. I am learning on Udemy.com how having the main at the bottom of the program helps when making blocks of code. What I would like to do is take some code I already have, and have the user enter two prime colors to make a mixed color, then ask the user if they would like to mix another color with two of the same prime and one other prime color to get: yellow-green, yellow-orange, orange-red, red-purple, blue-green, and blue-purple. Is my logic flawed? What is the correct logic? How would I set up this code to have different functions outside the main with the main at the bottom? I know it is supposed to read the information first somehow. I just can't process how to write it.
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
I am learning C++ and would like to make cleaner code. I am learning on Udemy.com how having the main at the bottom of the program helps when making blocks of code.
What I would like to do is take some code I already have, and have the user enter two prime colors to make a mixed color, then ask the user if they would like to mix another color with two of the same prime and one other prime color to get: yellow-green, yellow-orange, orange-red, red-purple, blue-green, and blue-purple.
Is my logic flawed? What is the correct logic? How would I set up this code to have different functions outside the main with the main at the bottom? I know it is supposed to read the information first somehow. I just can't process how to write it.
#include <iostream>
#include <string>
using namespace std;
int main() {
string arr[] = {"red", "blue", "yellow"};
int color1, color2;
string mixedColor;
char choice;
do {
cout << "Enter one prime color followed by a second prime color: "
<< "'0' for red, '1' for blue, and '2' for yellow: ";
cin >> color1 >> color2;
if ((color1 == 0 && color2 == 1) || (color1 == 1 && color2 == 0)) {
mixedColor = "purple";
} else if ((color1 == 0 && color2 == 2) || (color1 == 2 && color2 == 0)) {
mixedColor = "orange";
} else if ((color1 == 1 && color2 == 2) || (color1 == 2 && color2 == 1)) {
mixedColor = "green";
} else {
mixedColor = "No Mix";
}
// Display results
cout << "Mixed color for your selection (" << arr[color1] << " and " << arr[color2] << ") is " << mixedColor << endl;
// Continue or exit?
cout << "Would you like to mix again? (Y/N): ";
cin >> choice;
} while (toupper(choice) == 'Y');
cout << "Goodbye!" << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

I want to keep the array for this mixer and came up with this solution.
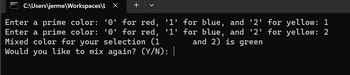