For this task you will need to implement a Guesses class, which will store all of the locations that a player has targeted/guessed so far. Again, the included Runner class is provided purely so you can manually debug your code by hitting the Run button and observing the results. It is not included in any tests, so feel free to make any changes you like to it. Your workspace for this task includes a compiled Cell class, that has been implemented according to the specification described in Part 1. Specification We have started you off with the skeleton of the Guesses class. Your task is to complete this class so that it has the following methods: A constructor that accepts no parameters, and results in a new instance of Guesses being created. An addGuess method, which accepts an instance of Cell, and returns nothing. Calling this method should result in this instance of Guesses remembering that the given location has been guessed. An isGuessed method, which accepts an instance of Cell, and returns either true or false depending on whether the given location has been previously guessed or not. A getTotalGuesses method, which accepts no parameters, and returns the number of guesses that have previously been made. This number should only go up when new valid guesses are made. If addGuess is called with an invalid location, or a location that has previously been guessed, then the number should remain unchanged. All of these methods should be public and not static. There should not be any other public methods or properties, but you are welcome to add any private properties and methods that you feel are necessary/helpful. Runner: import java.util.Scanner ; public class Runner { public static void main(String[] args) { Scanner input = new Scanner(System.in) ; Guesses guesses = new Guesses() ; //add a valid guess guesses.addGuess(new Cell(0,0)); //add the same guess guesses.addGuess(Cell.fromString("A1")) ; //add an invalid guess guesses.addGuess(new Cell(8,0)); //There should be one valid guess System.out.println("You have made " + guesses.getTotalGuesses() + " valid, distinct guesses") ; while (true) { System.out.println("Please enter a guess:") ; Cell guess = Cell.fromString(input.nextLine()); if (guess == null) { System.out.println("Invalid guess"); continue ; } if (guesses.isGuessed(guess)) { System.out.println("You have guessed this already!") ; } guesses.addGuess(guess) ; System.out.println("You have made " + guesses.getTotalGuesses() + " valid, distinct guesses") ; } } } Gusses code (required): public class Guesses { //Your code here }
For this task you will need to implement a Guesses class, which will store all of the locations that a player has targeted/guessed so far.
Again, the included Runner class is provided purely so you can manually debug your code by hitting the Run button and observing the results. It is not included in any tests, so feel free to make any changes you like to it.
Your workspace for this task includes a compiled Cell class, that has been implemented according to the specification described in Part 1.
Specification
We have started you off with the skeleton of the Guesses class. Your task is to complete this class so that it has the following methods:
A constructor that accepts no parameters, and results in a new instance of Guesses being created.
An addGuess method, which accepts an instance of Cell, and returns nothing. Calling this method should result in this instance of Guesses remembering that the given location has been guessed.
An isGuessed method, which accepts an instance of Cell, and returns either true or false depending on whether the given location has been previously guessed or not.
A getTotalGuesses method, which accepts no parameters, and returns the number of guesses that have previously been made. This number should only go up when new valid guesses are made. If addGuess is called with an invalid location, or a location that has previously been guessed, then the number should remain unchanged.
All of these methods should be public and not static. There should not be any other public methods or properties, but you are welcome to add any private properties and methods that you feel are necessary/helpful.
Runner:
import java.util.Scanner ;
public class Runner {
public static void main(String[] args) {
Scanner input = new Scanner(System.in) ;
Guesses guesses = new Guesses() ;
//add a valid guess
guesses.addGuess(new Cell(0,0));
//add the same guess
guesses.addGuess(Cell.fromString("A1")) ;
//add an invalid guess
guesses.addGuess(new Cell(8,0));
//There should be one valid guess
System.out.println("You have made " + guesses.getTotalGuesses() + " valid, distinct guesses") ;
while (true) {
System.out.println("Please enter a guess:") ;
Cell guess = Cell.fromString(input.nextLine());
if (guess == null) {
System.out.println("Invalid guess");
continue ;
}
if (guesses.isGuessed(guess)) {
System.out.println("You have guessed this already!") ;
}
guesses.addGuess(guess) ;
System.out.println("You have made " + guesses.getTotalGuesses() + " valid, distinct guesses") ;
}
}
}
Gusses code (required):
public class Guesses {
//Your code here
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

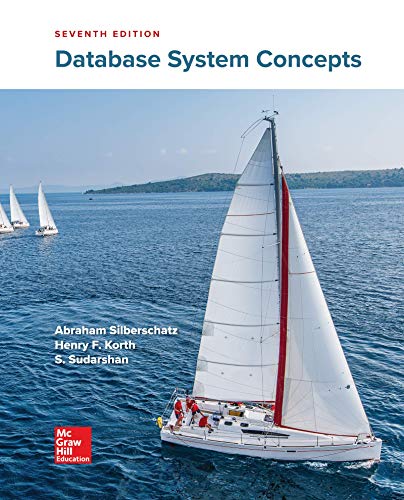
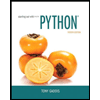
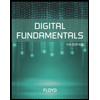
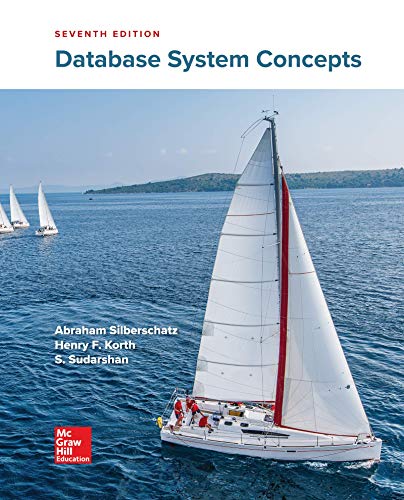
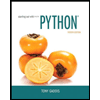
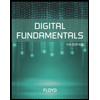
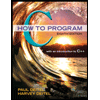
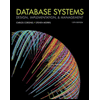
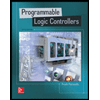