Develop a C# solution that retrieves a table definition from a SQL Server database and generates the C# source code representing the table as a C# class. The SQL used to obtain the table information is shown below. SELECT C.object_id, C.name as 'column_name', C.column_id, C.system_type_id, T.name as 'system_type_name', C.max_length, C.is_nullable FROM SYS.columns C, SYS. types T WHERE C.system_type_id= T.system_type_id AND C.object_id = (SELECT object_id FROM SYS. TABLES WHERE name = 'STT001_STUDENT') ORDER BY C. column_id; Query Results: object_id column_name column_id system_type_id system_type_name max_length is nullable
Develop a C# solution that retrieves a table definition from a SQL Server database and generates the C# source code representing the table as a C# class. The SQL used to obtain the table information is shown below. SELECT C.object_id, C.name as 'column_name', C.column_id, C.system_type_id, T.name as 'system_type_name', C.max_length, C.is_nullable FROM SYS.columns C, SYS. types T WHERE C.system_type_id= T.system_type_id AND C.object_id = (SELECT object_id FROM SYS. TABLES WHERE name = 'STT001_STUDENT') ORDER BY C. column_id; Query Results: object_id column_name column_id system_type_id system_type_name max_length is nullable
Chapter3: Data Representation
Section: Chapter Questions
Problem 3RP
Related questions
Question

Transcribed Image Text:Develop a C# solution that retrieves a table definition from a SQL Server database and
generates the C# source code representing the table as a C# class. The SQL used to obtain the
table information is shown below.
SELECT
C.object_id,
C.name as 'column_name',
C.column_id,
C.system_type_id,
T.name as 'system_type_name',
C.max_length,
C.is_nullable
FROM SYS.columns C, SYS. types T
WHERE C.system_type_id = T.system_type_id AND
C.object_id = (SELECT object_id FROM SYS. TABLES WHERE name = 'STT001_STUDENT')
ORDER BY C. column_id;
Query Results:
object_id column_name column_id system_type_id system_type_name max_length is_nullable
1205579333 Id
56
1205579333 GPA
108
175
167
167
1205579333 MajorCode
1205579333 FirstName
1205579333
LastName
1
}
2
3
4
5
An example output for this program is shown below.
public class Student
{
public int Id { get; set; }
public decimal GPA { get; set; }
public string MajorCode { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
int
numeric
char
varchar
varchar
4
5
5
50
50
dooooo
0
Even though the program is not yet complete, there is a buzz around the office about how
helpful this feature will be for other databases (e.g., Oracle, SQLite, MySQL, etc.) in addition to
SQL Server, so the design should support extending the application to support additional source
databases as enhancements in future. Additionally, there is a discussion of supporting other
language outputs, including Java, C++, Visual Basic, and Python; however, the initial
deployment is only required to support the C# programming language.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
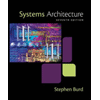
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
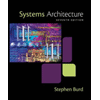
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning