This builds up on the the files provided, in wrote a program to count unigrams and bigrams and calculate conditional probabilities on each book. The goal of this assignment is to process multiple books in parallel. Specification: Download 20 books from (http://www.gutenberg.org/ebooks/) in the "Plain Text UTF-8" format. Create a directory and save the books under it. Please do not submit these books. Modify the provided program and write additional code, if needed, to process all books in parallel using: (1) Processes (H) Implicit threads using "#pragma omp critical (mutex_name)" and "#pragma omp parallel for" from the Open MP library, and (i) Explicit threads using the pthread library (Read Chapter 12 of the Head First C book). For (1), you must compile the provided code and name the executable "gutenberg." Then write another C program to run the gutenberg program on each file as an independent process. Important: once your program finishes running all processes, it must use the waitpid function on each child process in the parent process. For (li), your code must be compiled using the "-fopenmp" option to allow you to use the Open MP library for implicit threads. For (i) and (ili), your code must be compiled using the "-std=c11" option to ensure that malloc and free are thread safe. For (i), your code must be compiled using the "-pthread" option to allow proper execution of multi-threads. For (i) and (i) a block of consecutive print statements must be executed by a single thread only, Le., these statements must be treated as a critical section that is protected by its own mutex. Your program must accept multiple file names as command line arguments (no hard-coded file names in your code). To pass multiple file you the program use: > gutenberg some_directory/*.txt Your code will be tested using the latest version of the GNU C compiler on a Unix-based operating system. If you do not have a Unix-based machine you may want to use Windows Subsystem or a Virtual Box under your own responsibility! Your code must be well commented. When writing your comments, you should focus on what the code does at a high level; for example, describe the main steps of an algorithm-not every detail (that what the code is for). A ReadMe.txt file (including instructions on how to compile and how to run your program along with any known problems) must be provided under the subdirectory where the solution to a specific part is saved, i.e., three ReadMe files must be submitted-one for each part.
Use Bst Below As Example feel free to use Whatever Feels Easiest
#include "bst.h"
/**
* This function assumes that the key is allocated on the heap already
*/
node* insert(node *temp_root, char *key, double value) {
if (temp_root == NULL) {
temp_root = malloc(sizeof(node));
temp_root->key = key;
temp_root->value = value;
temp_root->left = NULL;
temp_root->right = NULL;
} else if (strcmp(key, temp_root->key) < 0) {
temp_root->left = insert(temp_root->left, key, value);
} else if (strcmp(key, temp_root->key) > 0) {
temp_root->right = insert(temp_root->right, key, value);
} else {
printf("%s already exists in the tree. No action taken\n", key);
}
return temp_root;
}
/**
* This functions searches for a key in a tree
* Returns the node that has the key or NULL otherwise
*/
node* find(node *temp_root, char *key) {
node *result = NULL;
if (temp_root != NULL) {
if (strcmp(key, temp_root->key) == 0) {
result = temp_root; // I found it
} else if (strcmp(key, temp_root->key) < 0) {
result = find(temp_root->left, key);
} else {
result = find(temp_root->right, key);
}
}
return result;
}
void print_helper(node *tree, FILE *out) {
if (tree != NULL) {
print_helper(tree->left, out);
fprintf(out, "%s\t%f\n", tree->key, tree->value);
print_helper(tree->right, out);
}
}
/**
* Print contents of a tree in order
*/
void tprint(node *tree, char *file_name) {
FILE *out = fopen(file_name, "w");
print_helper(tree, out);
fclose(out);
}
/**
* Reclaim memory on the heap
*/
void clear(node *tree) {
if (tree != NULL) {
clear(tree->left);
clear(tree->right);
free(tree->key);
free(tree);
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

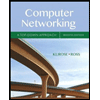
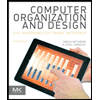
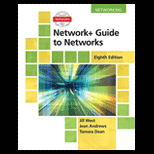
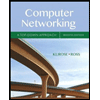
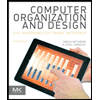
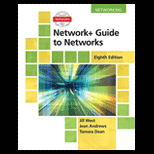
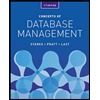
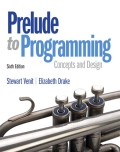
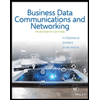