class LinkedListQ: def __init__(self); self.--head = None #head is the first node def append(self, data): temp1- Node (data) if self.__headNone: self.--head = temp! else: temp self.__head while temp.getNextO !-None: temptemp.getNextO temp.setNext(temp1) def __str__(self): temp self.__head mystr while temp != None; mystr + str(temp) + '->' # temp is a type Node temptemp.getNextO mystr += 'None' return mystr def __len__(self): temp self.__head if self.__headNone: return0 count 1 while temp.getNext() != None: temptemp.getNextO count += 1 return count def __getitem__(self, index): if index 〉 1en(self): print("Index out of bounds") return None temp self.__head for i in range(index): temptemp.getNextO return temp.getData)
This is for python
For this assignment, you will write a program to simulate a payroll application. To that effect, you will also create an Employee class, according to the specifications below. Since an Employee list might be large, and individual Employee objects may contain significant information themselves, we store the Employee list as a linked list.
Employee class:
Attributes
• Employee ID: you can use a string – the ID will contain digits and/or hyphens. The ID must be provided during object construction and there should be no
• Number of hours worked in a week: a floating-point number.
• Hourly pay rate: a floating-point number that represents how much the employee is paid for one hour of work.
• Gross wages: a floating-point number that stores the number of hours times the hourly rate.
Methods
• A constructor (__init__)
• Setter methods as needed.
• Getter methods as needed.
• This class should overload the __str__ or __repr__ methods so that Employee objects can be easily displayed using the print() function.
Node and Linked List class:
You will need to provide an implementation of the Node and the LinkedList classes. If you wish, you can re-use the code demoed during lecture but you will need to add a comment citing the source and you may need to modify it.
Script:
Your main script will simulate a basic payroll system. There should be a menu with the following options:
• Add new employee: this option allows you to enter the ID of a new employee and his/her hourly rate, creates an Employee object accordingly, and adds it to the list of Employees.
• Enter Employee Hours: this option displays each employee ID and asks the user to enter the number of hours worked by each employee. The script should also (re)calculate the gross wages for each employee (hours times pay rate) and update their associated attributes accordingly.
• Display Payroll: this option displays each employee’s identification number, hours worked, hourly rate, and gross wages.
• Update Hourly Rate: this option allows the user update the hourly rate of one employee. The user should enter the ID of the employee. If the ID exits in the list, then the hourly rate can be updated.
• Remove Employee for payroll: this option allows the user to remove an employee. The user should enter the ID of the employee. If the ID exits in the list, then the employee can be removed from the list.
• Quit the program You should continue to display the choices, and respond to user input, until the user chooses to quit/exit the program.
Validation:
• Each employee number should be unique. Do not accept duplicate employee numbers.
• Do not accept negative numbers for hours worked.
• Do not accept numbers less than 6.00 for pay rate.
This is the node class:
class Node():
def __init__(self, data, next = None):
self.data = data
self.next = next
def __str__(self):
return str(self.data)
def getNext(self):
return self.next
def setNext(self, n):
self.next = n
def getData(self):
return self.data
Attached is Linkedlist class, however, you need to make/add some changes to the class


Trending now
This is a popular solution!
Step by step
Solved in 6 steps

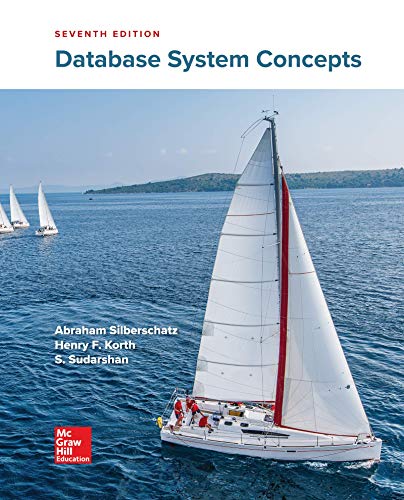
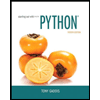
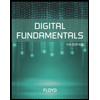
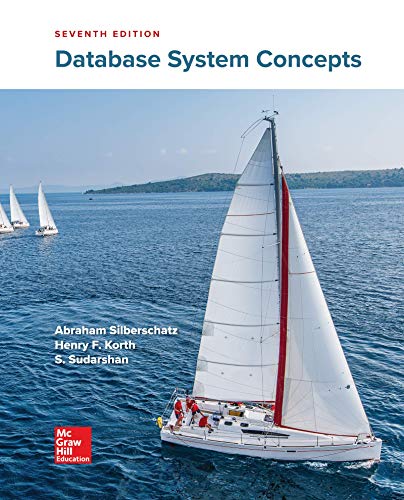
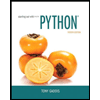
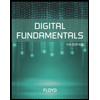
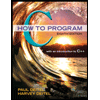
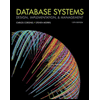
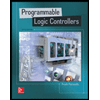