Calculator Assignment Modify a calculator program that currently displays the Abstract Syntax Tree (AST) structure after parsing an input expression. Instead of printing the AST, enhance the program to evaluate the expression and display the result. Add support for the binary "%" remainder (modulo) operator and introduce variables with the assignment operator "=". Key steps to implement the assignment operator and variables: Introduce a new static attribute in the AstNode class to represent variables: Add a method isIdentifier() to the TokenStream class to detect variables: Modify the primaryRule() in AstNode to handle variables and variable assignment: Handle new node types in the AstNode evaluate() method for variables and assignment: These changes allow the calculator to evaluate expressions, support variables, and handle variable assignments. Users can create and use variables in subsequent expressions. EmployeeJava Type: package week3; import static week3.MainApp.*; public enum EmployeeType { FULL_TIME (EMPLOYEE_FULL_TIME), PART_TIME (EMPLOYEE_PART_TIME), CONSULTANT (EMPLOYEE_CONSULTANT); private int value; public int getValue() { return value; } private EmployeeType( int value ) { this.value = value; } @Override public String toString() { if (this.ordinal() == FULL_TIME.ordinal() ) { return "FT"; } else if (this.ordinal() == PART_TIME.ordinal() ) { return "PT"; } else { return "CONSULTANT"; } } } MAINAPP.java package week3; public class MainApp { public static final int EMPLOYEE_FULL_TIME = 100; public static final int EMPLOYEE_PART_TIME = 200; public static final int EMPLOYEE_CONSULTANT = 300; private static void printEmployeeType(EmployeeType employeeType) { switch (employeeType) { case FULL_TIME: System.out.println(employeeType.getValue()); break; case PART_TIME: System.out.println(employeeType.getValue()); break; case CONSULTANT: System.out.println(employeeType.getValue()); break; } }//printEmployeeType public static void main(String[] args) { EmployeeType employeeType = EmployeeType.FULL_TIME; printEmployeeType( employeeType ); employeeType = EmployeeType.PART_TIME; printEmployeeType( employeeType ); employeeType = EmployeeType.CONSULTANT; printEmployeeType( employeeType ); }//main
Calculator Assignment
Modify a calculator
Key steps to implement the assignment operator and variables:
- Introduce a new static attribute in the AstNode class to represent variables:
- Add a method isIdentifier() to the TokenStream class to detect variables:
- Modify the primaryRule() in AstNode to handle variables and variable assignment:
- Handle new node types in the AstNode evaluate() method for variables and assignment:
These changes allow the calculator to evaluate expressions, support variables, and handle variable assignments. Users can create and use variables in subsequent expressions.
EmployeeJava Type:
package week3;
import static week3.MainApp.*;
public enum EmployeeType {
FULL_TIME (EMPLOYEE_FULL_TIME),
PART_TIME (EMPLOYEE_PART_TIME),
CONSULTANT (EMPLOYEE_CONSULTANT);
private int value;
public int getValue() {
return value;
}
private EmployeeType( int value ) {
this.value = value;
}
@Override
public String toString() {
if (this.ordinal() == FULL_TIME.ordinal() ) {
return "FT";
} else if (this.ordinal() == PART_TIME.ordinal() ) {
return "PT";
} else {
return "CONSULTANT";
}
}
}
MAINAPP.java
package week3;
public class MainApp {
public static final int EMPLOYEE_FULL_TIME = 100;
public static final int EMPLOYEE_PART_TIME = 200;
public static final int EMPLOYEE_CONSULTANT = 300;
private static void printEmployeeType(EmployeeType employeeType) {
switch (employeeType) {
case FULL_TIME:
System.out.println(employeeType.getValue());
break;
case PART_TIME:
System.out.println(employeeType.getValue());
break;
case CONSULTANT:
System.out.println(employeeType.getValue());
break;
}
}//printEmployeeType
public static void main(String[] args) {
EmployeeType employeeType = EmployeeType.FULL_TIME;
printEmployeeType( employeeType );
employeeType = EmployeeType.PART_TIME;
printEmployeeType( employeeType );
employeeType = EmployeeType.CONSULTANT;
printEmployeeType( employeeType );
}//main
}//class MainApp

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

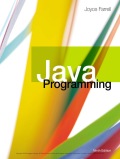
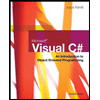
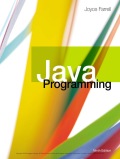
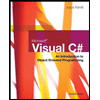