C++ Programming Language ::::::: Redo the same functions this time as nonmember functions please : NOTE: You can add only one function into the linked list class get_at_position which will return value of element at given position. 1) Insert before tail : Insert a value into a simply linked list, such that it's location will be before tail. So if a list contains {1, 2, 3}, insert before tail value 9 is called, the list will become {1, 2, 9, 3}. 2) Insert before value : Insert a value into a simply linked list, such that it's location will be before a particular value. So if a list contains {1, 2, 3}, insert before 2 value 9 is called, the list will become {1, 9, 2, 3}. 3)Count common elements : Count common values between two simply linked lists. So if a list1 contains {1, 2, 3, 4, 5}, and list2 contains {1, 3, 4, 6}, number of common elements is 3. 4) Check if sorted : Check if elements of simply linked lists are sorted in ascending order or not. So if a list contains {1, 3, 7, 8, 9} its sorted, but if a list contains {1, 3, 7, 2, 5} its not sorted. 5) Find sublist : Find and return sublist in a list, the start and stop positions indicate where the sublist starts and where it ends. So if a list contains {1, 3, 7, 8, 9}, sublist at start 2 and stop 4 is {3, 7, 8} . this is the simply linked list >>
C++ Programming Language ::::::: Redo the same functions this time as nonmember functions please :
NOTE: You can add only one function into the linked list class get_at_position which will return value of element at given position.
1) Insert before tail : Insert a value into a simply linked list, such that it's location will be before tail.
So if a list contains {1, 2, 3}, insert before tail value 9 is called, the list will become {1, 2, 9, 3}.
2) Insert before value : Insert a value into a simply linked list, such that it's location will be before a particular value.
So if a list contains {1, 2, 3}, insert before 2 value 9 is called, the list will become {1, 9, 2, 3}.
3)Count common elements : Count common values between two simply linked lists.
So if a list1 contains {1, 2, 3, 4, 5}, and list2 contains {1, 3, 4, 6}, number of common elements is 3.
4) Check if sorted : Check if elements of simply linked lists are sorted in ascending order or not.
So if a list contains {1, 3, 7, 8, 9} its sorted, but if a list contains {1, 3, 7, 2, 5} its not sorted.
5) Find sublist : Find and return sublist in a list, the start and stop positions indicate where the sublist starts and where it ends.
So if a list contains {1, 3, 7, 8, 9}, sublist at start 2 and stop 4 is {3, 7, 8} .
this is the simply linked list >>
#include <iostream>
using namespace std;
enum error {success,underflow,out};
struct node
{
int data;
node* next;
node()
{ next=0; }
node(int d,node *n=0)
{ data=d; next=n; }
};
class list
{
node *head,*tail;
public:
list();
bool is_empty();
void print();
int size();
void add_begin(int el);
void add_end(int el);
error add_pos(int el,int pos);
void add_sorted(int el);
error delete_begin();
error delete_end();
error delete_pos(int pos);
error delete_el(int el);
//bool search(int el);
int search(int el);
//node * search(int el);
~list();
list(list &o);
void operator=(list &o);
};
list::list()
{
head=tail=0;
}
bool list::is_empty()
{
return head==0;
}
void list::print()
{
for(node *i=head;i!=0;i=i->next)
cout<<i->data<<" ";
cout<<endl;
}
int list::size()
{
int c=0;
node *tmp=head;
for(;tmp!=0;tmp=tmp->next)
c++;
return c;
}
void list::add_end(int el)
{
if(is_empty())
head=tail=new node(el);
else
tail=tail->next=new node(el);
}
void list::add_begin(int el)
{
if(is_empty())
head=tail=new node(el);
else
head=new node(el,head);
}
error list::add_pos(int el,int pos)
{
if(pos<1 || pos>size()+1)
return out;
if(pos==1)
add_begin(el);
else
if(pos==size()+1)
add_end(el);
else
{
node *t1=head;
for(int i=1;i<pos-1;i++)
t1=t1->next;
t1->next=new node(el,t1->next);
}
return success;
}
error list::delete_begin()
{
if(is_empty())
return underflow;
if(size()==1) //or head==tail
//or head->next==0
{
delete head;
head=tail=0;
}
else
{
node *t=head;
head=head->next;
delete t;
}
return success;
}
error list::delete_end()
{
if(is_empty())
return underflow;
if(size()==1)
delete_begin();
else
{
node *t=head;
for(;t->next!=tail;t=t->next);
delete tail;
t->next=0;
tail=t;
}
return success;
}
error list::delete_pos(int pos)
{
if(is_empty())
return underflow;
if(pos<1 || pos>size())
return out;
if(pos==1)
delete_begin();
else
if(pos==size())
delete_end();
else
{
node *p1=head;
for(int i=1;i<pos-1;i++)
p1=p1->next;
node *p2=p1->next;
node *p3=p2->next;
delete p2;
p1->next=p3;
}
return success;
}
/*
bool list::search(int el)
{
for(node *t=head;t!=0;t=t->next)
if(el==t->data)
return true;
return false;
}
*/
int list::search(int el)
{
int c=1;
for(node *t=head;t!=0;t=t->next,c=c+1)
if(el==t->data)
return c;
return 0;
}
/*
node* list::search(int el)
{
for(node *t=head;t!=0;t=t->next)
if(el==t->data)
return t;
return 0;
}
*/
error list::delete_el(int el)
{
if(is_empty())
return underflow;
if(search(el)==0)
return out;
return delete_pos(search(el));
//int p=search(el);
//return delete_pos(p);
}
list::~list()
{
while(!is_empty())
delete_begin();
cout<<"Nodes deleted"<<endl;
}
void list::operator=(list &o)
{
while(!is_empty())
delete_end();
if(!o.is_empty())
{
node*t,*t2=o.head;
t=head=new node(t2->data);
while(t2->next!=0)
{
t2=t2->next;
t->next=new node(t2->data);
t=t->next;
}
tail=t;
}
else
head=tail=0;
}
list::list(list &o)
{
//while(!is_empty())
//delete_end();
if(!o.is_empty())
{
node*t,*t2=o.head;
t=head=new node(t2->data);
while(t2->next!=0)
{
t2=t2->next;
t->next=new node(t2->data);
t=t->next;
}
tail=t;
}
else
head=tail=0;
}

Step by step
Solved in 3 steps with 5 images

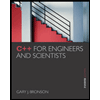
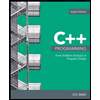
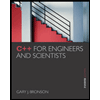
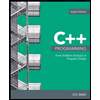