C++ HurdleGame The HurdleGame class contains all the functions invoked as a result of a user action (i.e. when a user presses a key on the Hurdle Frontend, one of the functions in HurdleGame will be called. A HurdleGame object comprises a HurdleWords object (which stores all valid guesses and valid Hurdles), and a HurdleState object, which stores and tracks all the game states. Any action should update the HurdleState object. Each function in HurdleGame is called as a result of a user action on the Hurdle Frontend. To understand this, please read the Hurdle Backend API section below before implementing. You are responsible for implementing each of the functions below. LetterEntered ● Called by the Hurdle frontend when the user enters a letter. ● Hint: when a user enters a letter, how should the underlying HurdleState be modified to reflect the change resulting from that key press? WordSubmitted ● Called by the Hurdle front end when the user submits a guess by pressing enter. ● Hint: when a user submits a guess, you must validate that guess: ○ You must check if that guess is a valid length (5 letters long). If it is not, then this is a suitable time to set the “errorMessage” field to indicate to the Hurdle Frontend that there are not enough letters in the submitted guess (see JSON Response section below) ○ You must also check if that guess is a valid guess (i.e. it must be in the Dictionary!). For example, “zzzzz” is not a real world, and would be invalid. Use the helper function you implemented in the Dictionary class to make this check. Like above, set the “errorMessage” field in the JSON response if needed. ○ Hint: Make sure to clear the errorMessage when an action is not an error. You may do so by setting the errorMessage to an empty string. LetterDeleted ● Called by the Hurdle front end when the user presses backspace. ● Hint: how does your HurdleState object store the letters of the guess being typed out? How should you modify the game state if a user backspaces (deletes) the last letter they typed? ● Hint: Are there situations when pressing backspace has no effect on the game state? NewHurdle ● Called by the Hurdle frontend when the user clicks "Next Hurdle". ● Hint: you should clean up the game state entirely (or instantiate from scratch) to start a brand-new game. A new secret Hurdle should be selected for the new game. hurdle.cc file #include "hurdle.h" // ========================= YOUR CODE HERE ========================= // This implementation file is where you should implement the member // functions declared in the header, only if you didn't implement // them inline in the header. // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the HurdleGame class. // =================================================================== crow::json::wvalue HurdleGame::JsonFromHurdleState() { // The JSON object to return to the Hurdle Frontend. crow::json::wvalue hurdle_state_json({}); // ===================== YOUR CODE HERE ===================== // Fill the hurdle_state_json with the data expected by the // Hurdle frontend. The frontend expects the following keys: // 1. "answer" // 2. "boardColors" // 3. "guessedWords" // 4. "gameStatus" // 5. "errorMessage" // 6. [OPTIONAL] "letterColors" // See the "JSON Response" section of tinyurl.com/cpsc121-s23-hurdle // // You can set the key in the JSON to a value like so: // hurdle_state_json[] = // // See below for an example to set the "answer" key: hurdle_state_json["answer"] = "titan"; // Replace this! // ========================================================== return hurdle_state_json; } hurdle.h file #include #include #include "hurdlewords.h" #include "hurdlestate.h" #include "server_utils/crow_all.h" #ifndef HURDLE_H #define HURDLE_H class HurdleGame { public: HurdleGame(HurdleWords words) : hurdlewords_(words), hurdle_state_(words.GetRandomHurdle()) {} // Called by the Hurdle frontend when the user clicks "Next Hurdle". void NewHurdle(); // Called by the Hurdle frontend when the user enters a letter. void LetterEntered(char key); // Called by the Hurdle frontend when the user submits a guess. void WordSubmitted(); // Called by the Hurdle frontend when the presses backspace. void LetterDeleted(); // JsonFromHurdleState returns a JSON object representing the Hurdle state. // This is used to send the game state to the Hurdle Frontend in a readable // format. crow::json::wvalue JsonFromHurdleState(); private: HurdleState hurdle_state_; HurdleWords hurdlewords_; }; #endif // HURDLE_H
C++
HurdleGame
The HurdleGame class contains all the functions invoked as a result of a user action (i.e. when a user presses a key on the Hurdle Frontend, one of the functions in HurdleGame will be called.
A HurdleGame object comprises a HurdleWords object (which stores all valid guesses and valid Hurdles), and a HurdleState object, which stores and tracks all the game states. Any action should update the HurdleState object.
Each function in HurdleGame is called as a result of a user action on the Hurdle Frontend. To understand this, please read the Hurdle Backend API section below before implementing.
You are responsible for implementing each of the functions below.
LetterEntered
● Called by the Hurdle frontend when the user enters a letter.
● Hint: when a user enters a letter, how should the underlying HurdleState be modified to reflect the change resulting from that key press?
WordSubmitted
● Called by the Hurdle front end when the user submits a guess by pressing enter.
● Hint: when a user submits a guess, you must validate that guess:
○ You must check if that guess is a valid length (5 letters long). If it is not, then this is a suitable time to set the “errorMessage” field to indicate to the Hurdle Frontend that there are not enough letters in the submitted guess (see JSON Response section below)
○ You must also check if that guess is a valid guess (i.e. it must be in the Dictionary!). For example, “zzzzz” is not a real world, and would be invalid. Use the helper function you implemented in the Dictionary class to make this check. Like above, set the “errorMessage” field in the JSON response if needed.
○ Hint: Make sure to clear the errorMessage when an action is not an error. You may do so by setting the errorMessage to an empty string.
LetterDeleted
● Called by the Hurdle front end when the user presses backspace.
● Hint: how does your HurdleState object store the letters of the guess being typed out? How should you modify the game state if a user backspaces (deletes) the last letter they typed?
● Hint: Are there situations when pressing backspace has no effect on the game state?
NewHurdle
● Called by the Hurdle frontend when the user clicks "Next Hurdle".
● Hint: you should clean up the game state entirely (or instantiate from scratch) to start a brand-new game. A new secret Hurdle should be selected for the new game.
hurdle.cc file
#include "hurdle.h"
// ========================= YOUR CODE HERE =========================
// This implementation file is where you should implement the member
// functions declared in the header, only if you didn't implement
// them inline in the header.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the HurdleGame class.
// ===================================================================
crow::json::wvalue HurdleGame::JsonFromHurdleState() {
// The JSON object to return to the Hurdle Frontend.
crow::json::wvalue hurdle_state_json({});
// ===================== YOUR CODE HERE =====================
// Fill the hurdle_state_json with the data expected by the
// Hurdle frontend. The frontend expects the following keys:
// 1. "answer"
// 2. "boardColors"
// 3. "guessedWords"
// 4. "gameStatus"
// 5. "errorMessage"
// 6. [OPTIONAL] "letterColors"
// See the "JSON Response" section of tinyurl.com/cpsc121-s23-hurdle
//
// You can set the key in the JSON to a value like so:
// hurdle_state_json[<key>] = <value>
//
// See below for an example to set the "answer" key:
hurdle_state_json["answer"] = "titan"; // Replace this!
// ==========================================================
return hurdle_state_json;
}
hurdle.h file
#include <string>
#include <vector>
#include "hurdlewords.h"
#include "hurdlestate.h"
#include "server_utils/crow_all.h"
#ifndef HURDLE_H
#define HURDLE_H
class HurdleGame {
public:
HurdleGame(HurdleWords words)
: hurdlewords_(words), hurdle_state_(words.GetRandomHurdle()) {}
// Called by the Hurdle frontend when the user clicks "Next Hurdle".
void NewHurdle();
// Called by the Hurdle frontend when the user enters a letter.
void LetterEntered(char key);
// Called by the Hurdle frontend when the user submits a guess.
void WordSubmitted();
// Called by the Hurdle frontend when the presses backspace.
void LetterDeleted();
// JsonFromHurdleState returns a JSON object representing the Hurdle state.
// This is used to send the game state to the Hurdle Frontend in a readable
// format.
crow::json::wvalue JsonFromHurdleState();
private:
HurdleState hurdle_state_;
HurdleWords hurdlewords_;
};
#endif // HURDLE_H

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

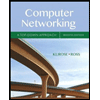
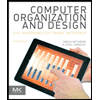
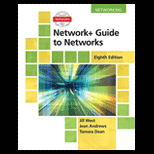
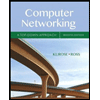
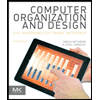
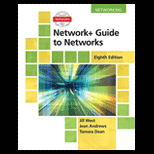
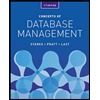
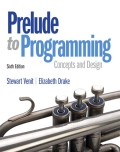
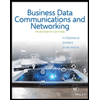