(Advanced C++) I need help to write an algorithm step for the two-part A&B codes below (Note: I have the code; I just need the algorithms).
(Advanced C++) I need help to write an
Part A:
#include <iostream>
#include <algorithm>
using namespace std;
void mysort(int *arr, int n)
{
sort(arr, arr+n); //sort is an inbuilt function
}
void avgscore(int *arr, int n, float *avgval)
{
*avgval=0.0; //initializing value
for (int i=0;i<n;i++)
{
*avgval=*avgval+*(arr+i);
}
*avgval=*avgval/(1.0*n);
}
int main()
{
int n;
cout<<"Enter Number of Test Scores\n";
cin>>n;
int *arr = new int[n];
cout<<"Enter Test Scores:\n";
int i,num;
for (i=0;i<n;i++) //taking inputs
{
cin>>num;
if(num<0)
{
cout<<"No negative numbers!\n";
if (i>0)
{
i--;
}
else
{
i=-1;
}
continue;
}
*(arr+i)=num;
}
mysort(arr,n);
float avgval;
avgscore(arr, n, &avgval);
cout<<"The sorted Array is:\n";
for (i=0;i<n;i++) //displaying sorted array
{
cout<<*(arr+i)<<" ";
}
cout<<endl;
cout<<"The Average Test Score is: "<<avgval<<endl;
return 0;
}
Part B:
#include <iostream>
#include <algorithm>
using namespace std;
typedef struct Student //declaring structure
{
string name;
int score;
}Student;
bool compareTwoScores(Student a, Student b) //Function to compare two structures according to score
{
return a.score < b.score;
}
void mysort(Student *arr, int n)
{
sort(arr, arr+n, compareTwoScores); //sort is an inbuilt function
}
void avgscore(Student *arr, int n, float *avgval)
{
*avgval=0.0; //initializing value
for (int i=0;i<n;i++)
{
*avgval=*avgval+(*(arr+i)).score;
}
*avgval=*avgval/(1.0*n);
}
int main()
{
int n;
cout<<"Enter Number of Test Scores\n";
cin>>n;
Student *arr = new Student[n];
cout<<"Enter Name of Student and Test Scores:\n";
int i,num;
string myname;
for (i=0;i<n;i++) //taking inputs
{
cin>>myname;
cin>>num;
if(num<0)
{
cout<<"No negative numbers!\n";
if (i>0)
{
i--;
}
else
{
i=-1;
}
continue;
}
(*(arr+i)).name=myname;
(*(arr+i)).score=num;
}
mysort(arr,n);
cout<<"The sorted Array is:\n";
for (i=0;i<n;i++) //displaying sorted array
{
cout<<(*(arr+i)).name<<" ";
cout<<(*(arr+i)).score<<"\n";
}
cout<<endl;
float avgval;
avgscore(arr, n, &avgval);
cout<<"The Average Test Score is: "<<avgval<<endl;
return 0;
}

Step by step
Solved in 2 steps

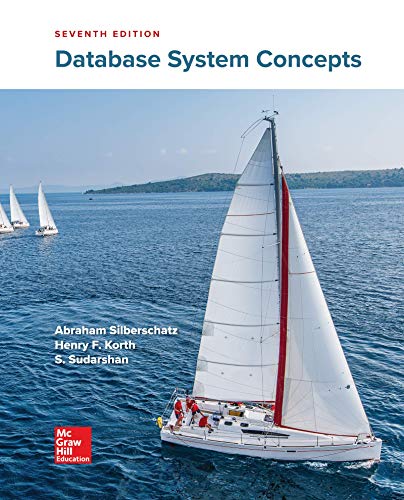
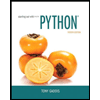
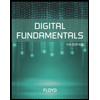
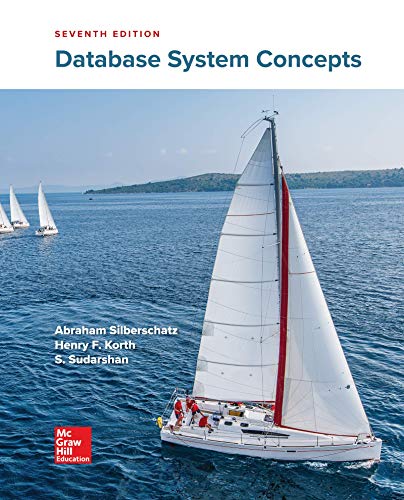
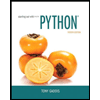
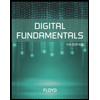
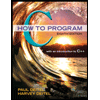
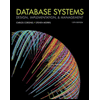
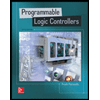