add override display method using System; //base class class Book{ //data members private string title; private string author; protected double price; //constructor public Book(string t, string a){ title = t; author = a; price = 500; } //method to display details public void display(){ Console.WriteLine("Title:" + title + " author:" + author + " price:" + price); } } //child class class PopularBooks : Book { //constructor public PopularBooks(string t, string a) :base(t,a) { price = 50000; } } class Program { static void Main() { //an array of 5 objects Book[] B = new Book[5]; //input title and author for(int i=0; i<5;i++){ Console.Write("Input title: "); string name = Console.ReadLine(); Console.Write("Input author name: "); string author = Console.ReadLine(); //if author is popular, //call constructor of sub class if (author.Equals("Khaled Hosseini") || author.Equals("Oscar Wilde") || author.Equals("Rembrandt")){ B[i] = new PopularBooks(name, author); } //call base class constructor else B[i] = new Book(name, author); Console.WriteLine(); } //display details of all books for (int i=0;i<5;i++){ B[i].display(); Console.WriteLine(); } } }
add override display method
using System;
//base class
class Book{
//data members
private string title;
private string author;
protected double price;
//constructor
public Book(string t, string a){
title = t;
author = a;
price = 500;
}
//method to display details
public void display(){
Console.WriteLine("Title:" + title + " author:" + author + " price:" + price);
}
}
//child class
class PopularBooks : Book {
//constructor
public PopularBooks(string t, string a) :base(t,a) {
price = 50000;
}
}
class Program {
static void Main() {
//an array of 5 objects
Book[] B = new Book[5];
//input title and author
for(int i=0; i<5;i++){
Console.Write("Input title: ");
string name = Console.ReadLine();
Console.Write("Input author name: ");
string author = Console.ReadLine();
//if author is popular,
//call constructor of sub class
if (author.Equals("Khaled Hosseini") || author.Equals("Oscar Wilde") || author.Equals("Rembrandt")){
B[i] = new PopularBooks(name, author);
}
//call base class constructor
else
B[i] = new Book(name, author);
Console.WriteLine();
}
//display details of all books
for (int i=0;i<5;i++){
B[i].display();
Console.WriteLine();
}
}
}

Step by step
Solved in 2 steps with 1 images

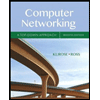
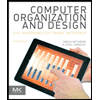
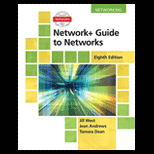
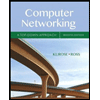
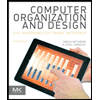
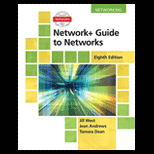
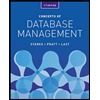
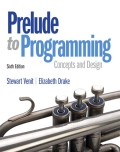
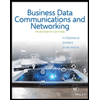