1. Listed next is the skeleton for a class named InventoryItem. Each inventory item has a name and a unique ID number: class InventoryItem private String name; private int uniqueItemID; } Flesh out the class with appropriate accessors, constructors, and mutators. The uniqueItemID 's are assigned by your store and can be set from outside the InventoryItem class-your code does not have to ensure that they are unique. Next, modify the class so that it implements the Comparable interface. The compareTo() method should compare the uniqueItemID 'S; e.g., the InventoryItem with item ID 5 is less than the InventoryItem with ID 10. Test your class by creating an array of sample InventoryItem 's and sort them using a sorting method that takes as input an array of type Comparable.
1. Listed next is the skeleton for a class named InventoryItem. Each inventory item has a name and a unique ID number: class InventoryItem private String name; private int uniqueItemID; } Flesh out the class with appropriate accessors, constructors, and mutators. The uniqueItemID 's are assigned by your store and can be set from outside the InventoryItem class-your code does not have to ensure that they are unique. Next, modify the class so that it implements the Comparable interface. The compareTo() method should compare the uniqueItemID 'S; e.g., the InventoryItem with item ID 5 is less than the InventoryItem with ID 10. Test your class by creating an array of sample InventoryItem 's and sort them using a sorting method that takes as input an array of type Comparable.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 5RQ
Related questions
Question
I need some help with this coding problem
![1. Listed next is the skeleton for a class named InventoryItem. Each inventory item has a name and a
unique ID number:
class InventoryItem
private String name;
private int uniqueItemID;
Flesh out the class with appropriate accessors, constructors, and mutators. The uniqueItemID 's are
assigned by your store and can be set from outside the InventoryItem class-your code does not have
to ensure that they are unique. Next, modify the class so that it implements the Comparable interface.
The compareTo() method should compare the uniqueItemID 's; e.g., the InventoryItem with item ID 5 is
less than the InventoryItem with ID 10. Test your class by creating an array of sample InventoryItem 's
and sort them using a sorting method that takes as input an array of type Comparable.
2. Define an interface named Shape with a single method named area that calculates the area of the
geometric shape:
public double area();
Next, define a class named Circle that implements Shape . The Circle class should have an instance
variable for the radius, a constructor that sets the radius, accessor/ mutator methods for the radius,
and an implementation of the area method. Also define a class named Rectangle that implements
Shape. The Rectangle class should have instance variables for the height and width, a constructor that
sets the height and width, accessor and mutator methods for the height and width, and an
implementation of the area method.
The following test code should then output the area of the Circle and Rectangle objects:
public static void main(String[] args)
{
Circle c = new Circle(4); // Radius of 4
Rectangle r = new Rectangle(4,3); // Height
showArea (c);
showArea (r);
4, width = 3
}
public static void showArea (Shape s)
double area = s.area();
System.out.println("The area of the shape is
area);
+](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F62cc3b2c-ca5c-4dc2-884f-aa56aeff03f7%2Fded73906-bc44-4b5a-990a-435dff85bd49%2Fjfk5qrn_processed.png&w=3840&q=75)
Transcribed Image Text:1. Listed next is the skeleton for a class named InventoryItem. Each inventory item has a name and a
unique ID number:
class InventoryItem
private String name;
private int uniqueItemID;
Flesh out the class with appropriate accessors, constructors, and mutators. The uniqueItemID 's are
assigned by your store and can be set from outside the InventoryItem class-your code does not have
to ensure that they are unique. Next, modify the class so that it implements the Comparable interface.
The compareTo() method should compare the uniqueItemID 's; e.g., the InventoryItem with item ID 5 is
less than the InventoryItem with ID 10. Test your class by creating an array of sample InventoryItem 's
and sort them using a sorting method that takes as input an array of type Comparable.
2. Define an interface named Shape with a single method named area that calculates the area of the
geometric shape:
public double area();
Next, define a class named Circle that implements Shape . The Circle class should have an instance
variable for the radius, a constructor that sets the radius, accessor/ mutator methods for the radius,
and an implementation of the area method. Also define a class named Rectangle that implements
Shape. The Rectangle class should have instance variables for the height and width, a constructor that
sets the height and width, accessor and mutator methods for the height and width, and an
implementation of the area method.
The following test code should then output the area of the Circle and Rectangle objects:
public static void main(String[] args)
{
Circle c = new Circle(4); // Radius of 4
Rectangle r = new Rectangle(4,3); // Height
showArea (c);
showArea (r);
4, width = 3
}
public static void showArea (Shape s)
double area = s.area();
System.out.println("The area of the shape is
area);
+
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
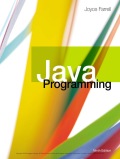
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
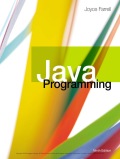
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT