1 Description of the Program In this assignment, you will make two classes, FullTime Employee and PartTimeEmployee, that inherit from a superclass Employee. The implementation of class Employee is given. You will also need to write a test program to test the methods you write for these two classes. The implementation details are described as follows. Stage1: In the first file Full TimeEmployee.java, you should include the following addi- tional instance variables and methods (other than all instance variables and methods inher- ited from class Employee): Private instance variable weeklySalary: • A constructor takes four inputs (firstname, lastname, SSN and salary); • One additional getter method to return the instance variable (accessor); One setter method to set the instance variable (mutator); • One overriding method earnings () that returns the weekly earnings (should be as same as the getSalary() method); A method toString that converts an fulltime employee's information into string form (including firstname, last name, ssn, earning and class name). The string should have a neat output format as shown in Figure 1. You should override superclass toString() method. Stage2: In the second file Part Time Employee.java, you should include the following addi- tional instance variables and methods (other than all instance variables and methods inher- ited from class Employee): Private instance variables wage (wage per hour) and hours (hours worked for week); • A constructor takes five inputs: firstname, firstname, SSN, hours, and wage; Two additional getter methods to return the instance variables (accessor); • Two setter methods to set the instance variables (mutator);
Go off this code:
public abstract class Employee
{
private String firstName;
private String lastName;
private String SSN;
double salary;
public Employee( String first, String last, String ssn )
{
firstName = first;
lastName = last;
SSN = ssn;
}
public void setfirstName( String first )
{
firstName = first; // should validate
}
public String getfirstName()
{
return firstName;
}
public void setlastName( String last )
{
lastName = last; // should validate
}
public String getlastName()
{
return lastName;
}
public void setSSN( String ssn )
{
this.SSN = ssn;
}
public String getSSN()
{
return SSN;
}
@Override
public String toString()
{
return String.format( "%-12s%-12s%-12s", getfirstName(), getlastName(), getSSN() );
}
public abstract double earnings();
}
![One overriding method earnings () that returns the earnings based on hours and wage.
For hours in excess of 40 hours, the pay will be time-and-a-half (Check your output
with the output in Figure 1 to make sure your calculation is correct);
• A method toString that converts an parttime employee's information into string form
(including firstname, lastname, ssn, earnings and class name). The string should have
a neat output format as shown in Figure 1. You should override superclass toString()
method.
Blue: Terminal Window - Prog3_Employee_v2
Options
A List of Employees, Earnings and class Types:
John
Larry
Sally
Smith
Darren
Ashley
Smith
Lippert
Kelly
Wilson
Weber
Parker
333-44-9982
449-22-2340
400-33-5571
880-25-3412
594-98-3411
888-33-3399
$800.50 FullTimeEmployee
$250.20 PartTimeEmployee
$400.00
PartTimeEmployee
$1,250.00 FullTimeEmployee
Part Time Employee
FullTimeEmployee
$550.00
$1,195.00
Figure 1: A screenshot of the program output.
Stage 3: In the third file EmployeeTester.java, you will need to do the followings:
1. You will create an array of Employee type, with the array size of 10. The goal here
is to use this array to store the references for subclass objects (FullTime Employee,
Part Time Employee). This is the application of Polymorphism we discussed in class.
2. You will initialize each object of the array using the values from the file of "data.txt"
[Note: Your must read the values in from the file using Scanner object, you
are not allowed to initialize the objects manually]. The first value in each line
of the file could be 1 (Full Time Employee) or 2 (Part TimeEmployee), which will be
used to determine which kind of object you will initialize. In another word, if you read
1, then you will create an object of FullTime Employee. Otherwise, you will create
an object of Part Time Employee. The remaining values of the lines will be used to
initialize each instance variable of that particular type of the object.
3. You will print out the contents of each element (i.e., object) in the array using toString()
method. Keep in mind, Polymorphism will take care for you so you don't need to check
what kind of objects you are invoking.
Your outputs should look like the one I provide in Figure 1.
2](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffa5ca6d3-7e28-4545-b49f-c13c41c00283%2F31d0e7d1-34a6-44b3-bdbc-7e78247cb249%2Fv6ye7u_processed.png&w=3840&q=75)


Step by step
Solved in 6 steps with 5 images

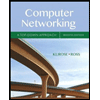
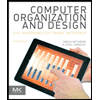
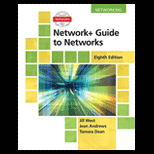
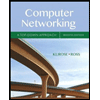
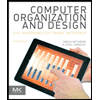
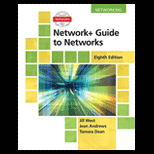
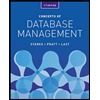
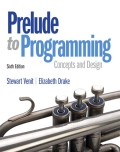
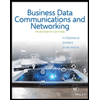