0.3 Write a Python program that manages a store with the following specifications: 0.3.1 Program flow: 1. When the program starts, main() calls readStoreItems() that asks the user to input a file name which contains the store items. If the file is not found, appropriate message should be shown and exceptions should be handled. The program should keep asking the user for a file name until a file is found. 2. When a file is found, the program reads the available store items (item name and price) from the file and stores them in a dictionary which is returned to the main() function. A sample input file is shown below: Milk 4.95 Tea 3.0 Coffee 18.5 3. The printMenu() is then called to display the menu with the following options: 1 Display all items 2 Add an item 3 Remove an item 4 Update the price of an item 5 Save the items to a file 6 Exit 4. The program then prompts the user to input a selection. 5. Every time the user makes invalid selection, a message is displayed, and the user is asked to input another selection until a valid selection is made. 6. After the execution of every selection, the menu is displayed again, and the user is prompted to enter a selection until he selects option 8. 0.3.2 Coding specification: 1. Define a class Item that has the following methods: . displayAllItems: Display all available items (Item name and price). • addItem: Ask the user to input a new item name to add. If the item already exists, a message should be shown, and the item is not added. If the item is not found, ask the user to input the price for that item, then add the item and its price to the item list (the dictionary). • removeItem: Ask the user to input an item to remove, if the item is not available, an appropriate error message should be shown, otherwise, the item is removed. • updateItem: Ask the user for an Item to update. If the item is not available, an appropriate error message should be displayed, otherwise, ask the user for the new price and update it. If the price is not valid an appropriate error message should be displayed. • saveItems: Ask the user to input a file name and save all items in the dictionary to the file. Appropriate exceptions should be handled. 2. The main() function should . Create an object "myStore" from Item class . Ask the user to select an input file • Display the menu and ask the use to select an option from the menu . Control the calls for the functions in them class 0.3.3 General requirements: 1. Make sure to handle all required exceptions when dealing with files (read or write) or data type conversion. 2. Provide appropriate documentation for the code 3. Define appropriate variables. 4. Assume the data in the file is with valid format • Sample program run: Enter filename: myfile.txt Error: the input file: myfile.txt is not found Enter filename: data.txt Select your option 1 Display all items 2 Add an item 3 Remove an item 4 Update the price of an item 5 Save the items to a file 6 Exit Enter your selection: 1 The available items are: MIIK 4.75 Coffee 18.50 Bread 4.20 Tea 2.10 Select your option Select yo option Display all items 2 All St update the price of an item 5 Save the items to a file & Exit Enter an ito name to add: Tea The item Tea already exists, it cannot be added Display all items 2 Allan ten update the price of an item Save the items to a file & vit ****..... Enter your selection: 2 inter an Its name to add: Apple Enter the new Ites Price: 2.8 Apple has been added successfully Select your option 1 Display all items 2 Add an to 3 vit date the price of an item S Save the items to a file 6 fait Enter your selection: 3 inter an ito name to Potato The item Potate is not available, try another ites 1 Display all tas 2 Add a Sto date the price of an item 5 Save the items to a file & bit inter your selection: 3 Tea Mas been removed successfully Select your option Display all items 2 Allan Ste update the price of an ites S Save the items to a file Int Enter your selection: Enter an iton Nane to update: Te The ten Tea is not available, try another it Select your option 3 Display all items 1 ts update the price of an ite Save the items to file a & frit inter your selection Enteritos Nane to update: Pilk inter the new price: 4.1 The price for: is has been modified successfully, the new **************** *************** Select your option Display all its 2 Add an its 3 ite update the price of an ite S Save the items to a file fait wwwww. inter your selection Enter filme datata Select your aption 1 Display all items 2 Add an Ste 3 oven its update the price of an ite Save the items to a file Thank you for using my Program
0.3 Write a Python program that manages a store with the following specifications: 0.3.1 Program flow: 1. When the program starts, main() calls readStoreItems() that asks the user to input a file name which contains the store items. If the file is not found, appropriate message should be shown and exceptions should be handled. The program should keep asking the user for a file name until a file is found. 2. When a file is found, the program reads the available store items (item name and price) from the file and stores them in a dictionary which is returned to the main() function. A sample input file is shown below: Milk 4.95 Tea 3.0 Coffee 18.5 3. The printMenu() is then called to display the menu with the following options: 1 Display all items 2 Add an item 3 Remove an item 4 Update the price of an item 5 Save the items to a file 6 Exit 4. The program then prompts the user to input a selection. 5. Every time the user makes invalid selection, a message is displayed, and the user is asked to input another selection until a valid selection is made. 6. After the execution of every selection, the menu is displayed again, and the user is prompted to enter a selection until he selects option 8. 0.3.2 Coding specification: 1. Define a class Item that has the following methods: . displayAllItems: Display all available items (Item name and price). • addItem: Ask the user to input a new item name to add. If the item already exists, a message should be shown, and the item is not added. If the item is not found, ask the user to input the price for that item, then add the item and its price to the item list (the dictionary). • removeItem: Ask the user to input an item to remove, if the item is not available, an appropriate error message should be shown, otherwise, the item is removed. • updateItem: Ask the user for an Item to update. If the item is not available, an appropriate error message should be displayed, otherwise, ask the user for the new price and update it. If the price is not valid an appropriate error message should be displayed. • saveItems: Ask the user to input a file name and save all items in the dictionary to the file. Appropriate exceptions should be handled. 2. The main() function should . Create an object "myStore" from Item class . Ask the user to select an input file • Display the menu and ask the use to select an option from the menu . Control the calls for the functions in them class 0.3.3 General requirements: 1. Make sure to handle all required exceptions when dealing with files (read or write) or data type conversion. 2. Provide appropriate documentation for the code 3. Define appropriate variables. 4. Assume the data in the file is with valid format • Sample program run: Enter filename: myfile.txt Error: the input file: myfile.txt is not found Enter filename: data.txt Select your option 1 Display all items 2 Add an item 3 Remove an item 4 Update the price of an item 5 Save the items to a file 6 Exit Enter your selection: 1 The available items are: MIIK 4.75 Coffee 18.50 Bread 4.20 Tea 2.10 Select your option Select yo option Display all items 2 All St update the price of an item 5 Save the items to a file & Exit Enter an ito name to add: Tea The item Tea already exists, it cannot be added Display all items 2 Allan ten update the price of an item Save the items to a file & vit ****..... Enter your selection: 2 inter an Its name to add: Apple Enter the new Ites Price: 2.8 Apple has been added successfully Select your option 1 Display all items 2 Add an to 3 vit date the price of an item S Save the items to a file 6 fait Enter your selection: 3 inter an ito name to Potato The item Potate is not available, try another ites 1 Display all tas 2 Add a Sto date the price of an item 5 Save the items to a file & bit inter your selection: 3 Tea Mas been removed successfully Select your option Display all items 2 Allan Ste update the price of an ites S Save the items to a file Int Enter your selection: Enter an iton Nane to update: Te The ten Tea is not available, try another it Select your option 3 Display all items 1 ts update the price of an ite Save the items to file a & frit inter your selection Enteritos Nane to update: Pilk inter the new price: 4.1 The price for: is has been modified successfully, the new **************** *************** Select your option Display all its 2 Add an its 3 ite update the price of an ite S Save the items to a file fait wwwww. inter your selection Enter filme datata Select your aption 1 Display all items 2 Add an Ste 3 oven its update the price of an ite Save the items to a file Thank you for using my Program
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:0.3 Write a Python program that manages a store with the following specifications:
0.3.1 Program flow:
1. When the program starts, main() calls readStoreItems() that asks the user to input a file name which contains the store items. If the file is not
found, appropriate message should be shown and exceptions should be handled. The program should keep asking the user for a file name until a file is
found.
2. When a file is found, the program reads the available store items (item name and price) from the file and stores them in a dictionary which is returned to
the main() function. A sample input file is shown below:
Milk
4.95
Tea
3.0
Coffee
18.5
3. The printMenu() is then called to display the menu with the following options:
1 Display all items.
2 Add an item
3 Remove an item
4 Update the price of an item
5 Save the items to a file
6 Exit
4. The program then prompts the user to input a selection.
5. Every time the user makes invalid selection, a message is displayed, and the user is asked to input another selection until a valid selection is made.
6. After the execution of every selection, the menu is displayed again, and the user is prompted to enter a selection until he selects option 6.
0.3.2 Coding specification:
1. Define a class Item that has the following methods:
. displayAllItems: Display all available items (Item name and price).
• addItem: Ask the user to input a new item name to add. If the item already exists, a message should be shown, and the item is not added. If the
item is not found, ask the user to input the price for that item, then add the item and its price to the item list (the dictionary).
• removeItem: Ask the user to input an item to remove, if the item is not available, an appropriate error message should be shown, otherwise, the
item is removed.
• updateItem: Ask the user for an Item to update. If the item is not available, an appropriate error message should be displayed, otherwise, ask the
user for the new price and update it. If the price is not valid an appropriate error message should be displayed.
• saveItems: Ask the user to input a file name and save all items in the dictionary to the file. Appropriate exceptions should be handled.
2. The main() function should
• Create an object "myStore" from Item class
. Ask the user to select an input file
• Display the menu and ask the use to select an option from the menu
• Control the calls for the functions in Item class
0.3.3 General requirements:
1. Make sure to handle all required exceptions when dealing with files (read or write) or data type conversion.
2. Provide appropriate documentation for the code.
3. Define appropriate variables.
4. Assume the data in the file is with valid format.
• Sample program run
Enter filename: myfile.txt
Error: the input file: myfile.txt is not found
Enter Filename: data.txt
Select your option
1 Display all items
2 Add an item
3 Renove an iten
4 Update the price of an item
5 Save the items to a file
6 Exit
****
Enter your selection: 1
The available items are:
Milk
4.75
Coffee
18.50
Bread
4.20
Tea
2.10
71
Select your option
1 Display all items
2 Add an item
3 Remove an iton
4 Update the price of an ites
5 Save the items to a file
6 Exit
inter your selection: 2
Enter an Ites nase to add: Tea
The iten Tea
The aleate existe st cent be added
al
Select your option
Display all items
2 Add t
3 Remove an ite
4 update the price of an ites
5 Save the items to a file
6 Exit
.......
Enter your selection: 2
Enter an Its name to add: Apple
Enter the new Ites Price: 2.8
Apple has been added successfully
Select your option
1 Display all items
2 Add an ite
3 Remove
it
4 update the price of an item
5 Save the items to a file
6 Exit
Enter your selection: 3
Enter an Itos nase to Rosove: Potato
The item Potato is not available, try another ite
www.
******
Select your option
1
Display all items
2 Add an It
3 Remove
it
4 update the price of an item
5 Save the items to a file
6 Exit
DEN
*************
***************
inter your selection: 3
Enter an Its name to Remove: Tea
Tea Has been removed successfully
Select your option
Display all items
2 Add to
3 Remove an ites
4 update the price of an item
5 Save the items to a file
Exit
MAS
Enter your selection: 4
telection
Enter an ite Nase to update: Tea
The iten Tea is not available, try another item
Select your option
Display all items
2 Add an ite
3 Remove an item
3
vit
4 update the price of an ites
s Save the items to a file
6 Exit
Enter your selection: 4
Enter an itos Nase to update: Milk
Enter the new price: 4.1
The price for: milk has been modified successfully, the new pr
******************
Select your option
1 Display all items
2 Add
to
3 Remove an item
4 update the price of an ites
5 Save the items to a file
6 Exit
inter your selection:
Enter Filename: data.txt
********
Select your option
1 Display all its
2 Add an i
3 Remove an iton
4 update the price of an item
5 Save the items to a file
6 Exit
inter your selection:
Thank you for using my Program
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
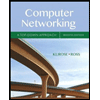
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
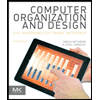
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
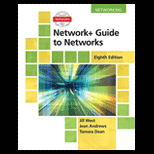
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
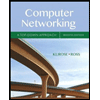
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
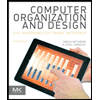
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
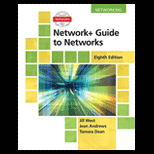
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
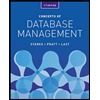
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
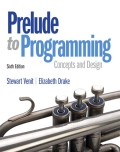
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
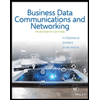
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY